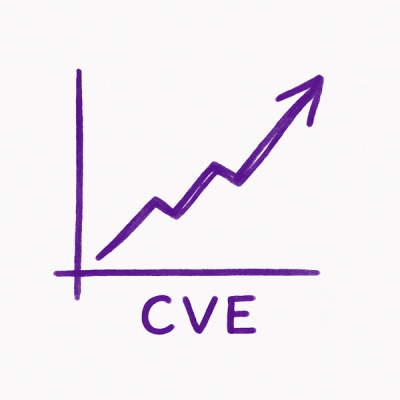
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
async-utils-ts
Advanced tools
A collection of utility functions for asynchronous operations in TypeScript.
This is a collection of TypeScript utility functions for handling asynchronous tasks.cript.
Using npm:
npm install async-utils-ts
Using yarn:
yarn add async-utils-ts
Creates a new Promise which resolves by queueing a microtask.
microtask().then(() => {
console.log('This will run as soon as possible, asynchronously.');
});
Creates a promise that resolves after the current event loop has been processed (i.e., after all microtasks have been completed).
macrotask().then(() => {
console.log('This is a macrotask');
});
Creates a new Promise that is resolved using requestAnimationFrame
.
async function animate() {
// Wait for the next frame
await animationFrame();
}
Creates a Promise that resolves after a specified duration.
// Wait for 1 second (1000 ms)
await timeout(1000);
Filters elements of the input array asynchronously based on the provided predicate function.
const arr = [1, 2, 3, 4, 5];
// Predicate function to check if a number is even
async function isEven(num) {
return num % 2 === 0;
}
// Usage
const result = await filter(arr, isEven);
console.log(result); // outputs: [2, 4]
Checks if any of the elements from the iterable satisfy the condition of the provided predicate function.
const iter = [1, 2, 3, 4, 5];
// Predicate function to check if a number is even
async function isEven(num) {
return num % 2 === 0;
}
// Usage
const result = await pSome(iter, isEven);
console.log(result); // outputs: true
Checks if none of the elements from the iterable pass the condition of the provided predicate function.
const iter = [1, 2, 3, 4, 5];
// Predicate function to check if a number is even
async function isEven(num) {
return num % 2 === 0;
}
// Usage
const result = await pNone(iter, isEven);
console.log(result); // outputs: false
Checks if all elements from the iterable satisfy the condition of the provided predicate function.
const iter = [1, 2, 3, 4, 5];
// Predicate function to check if a number is even
async function isEven(num) {
return num % 2 === 0;
}
// Usage
const result = await pEvery(iter, isEven);
console.log(result); // outputs: false
Retrieves the first value from an async generator.
async function* asyncGen() {
yield 1;
yield 2;
yield 3;
}
// Usage
const result = await first(asyncGen());
console.log(result); // outputs: 1
async-utils-ts
is licensed under the MIT License. Feel free to use
and contribute!
For issues or suggestions, please open an issue.
Happy async coding! 🚀
FAQs
A collection of utility functions for asynchronous operations in TypeScript.
We found that async-utils-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.