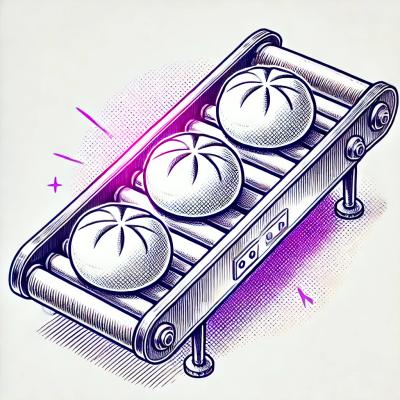
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
authentication-backend
Advanced tools
minimal API for integration with external authentication providers
Provides minimal backend functionality for integrating with external authentication providers.
Currently supports Google, GitHub and GitLab.
npm install --save authentication-backend
import express from 'express';
import { buildAuthenticationBackend } from 'authentication-backend';
const config = {
google: {
clientId: 'my-google-client-id',
authUrl: 'https://accounts.google.com/o/oauth2/auth',
tokenInfoUrl: 'https://oauth2.googleapis.com/tokeninfo',
},
github: {
clientId: 'my-github-client-id',
clientSecret: 'my-github-client-secret',
authUrl: 'https://github.com/login/oauth/authorize',
accessTokenUrl: 'https://github.com/login/oauth/access_token',
userUrl: 'https://api.github.com/user',
},
gitlab: {
clientId: 'my-gitlab-client-id',
authUrl: 'https://gitlab.com/oauth/authorize',
tokenInfoUrl: 'https://gitlab.com/oauth/token/info',
},
};
function tokenGranter(userId, service, externalId) {
// database-based example:
const myUserSessionToken = uuidv4();
myDatabase.recordUserSession(myUserSessionToken, userId);
return myUserSessionToken;
}
const auth = buildAuthenticationBackend(config, tokenGranter);
express()
.use('/my-prefix', auth.router)
.listen(8080);
You will need to do some work for each service on the client-side too.
See the source in /example/static
for a reference implementation.
This package also contains a mock SSO server, which can be run alongside your app (this is useful for local development and testing):
import express from 'express';
import { buildAuthenticationBackend, buildMockSsoApp } from 'authentication-backend';
buildMockSsoApp().listen(9000);
const config =
google: {
clientId: 'my-google-client-id',
authUrl: 'http://localhost:9000/auth',
tokenInfoUrl: 'http://localhost:9000/tokeninfo',
},
};
// ...
const auth = buildAuthenticationBackend(config, tokenGranter);
express()
.use('/my-prefix', auth.router)
.listen(8080);
You will need a Google client ID:
http://localhost:8080
/<my-prefix>/google
appended to the end.You can now configure the client ID in your app:
const config =
google: {
clientId: 'something.apps.googleusercontent.com', // <-- replace
authUrl: 'https://accounts.google.com/o/oauth2/auth',
tokenInfoUrl: 'https://oauth2.googleapis.com/tokeninfo',
},
};
You will need a GitHub client ID:
http://localhost:8080
/<my-prefix>/github
appended to the end.You can now configure the client ID and secret in your app:
const config =
github: {
clientId: 'my-github-client-id', // <-- replace
clientSecret: 'my-github-client-secret', // <-- replace
authUrl: 'https://github.com/login/oauth/authorize',
accessTokenUrl: 'https://github.com/login/oauth/access_token',
userUrl: 'https://api.github.com/user',
},
};
You will need a GitLab client ID:
/<my-prefix>/gitlab
appended to the end. e.g. for local
testing, this could be http://localhost:8080/<my-prefix>/gitlab
You can now configure the application ID in your app:
const config =
gitlab: {
clientId: 'my-gitlab-application-id', // <-- replace
authUrl: 'https://gitlab.com/oauth/authorize',
tokenInfoUrl: 'https://gitlab.com/oauth/token/info',
},
};
This expects you to create a frontend which handles the user interaction and propagates returned data to the API.
/
This will return the public parts of your config (i.e. clientId
and authUrl
for each service).
Example:
{
"google": {
"clientId": "my-google-client-id",
"authUrl": "https://accounts.google.com/o/oauth2/auth"
},
"github": {
"clientId": "my-github-client-id",
"authUrl": "https://github.com/login/oauth/authorize"
},
"gitlab": {
"clientId": "my-gitlab-client-id",
"authUrl": "https://gitlab.com/oauth/authorize"
}
}
Any services which have not been configured will be omitted from the response.
/<service-name>
Where <service-name>
is google
, github
or gitlab
.
This expects to receive JSON-encoded data:
{
"externalToken": "token-returned-by-service"
}
It will check the token with the service, and if successful, will invoke the configured
tokenGranter
function with a user ID, service name, and service user ID. The string
returned by tokenGranter
will be sent to the user in a JSON response:
{
"userToken": "returned-token-granter-value"
}
If the check fails, an error will be returned instead, with a status code of 4xx or 5xx:
{
"error": "an error message"
}
FAQs
minimal API for integration with external authentication providers
The npm package authentication-backend receives a total of 53 weekly downloads. As such, authentication-backend popularity was classified as not popular.
We found that authentication-backend demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.