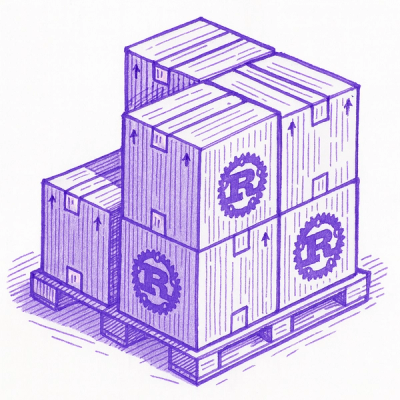
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
A wrapper for async/await calls without the need of try/catch block.
If someone told you that you should try / catch
once at the root of your application and then do your async calls anywhere in the codebase, they are wrong. You should try / catch
every async / await
call or use .then() / .catch()
chainables. Better than callback hell? I think it leads to similar problem...
This module attempts to solve that nesting hell and instead provide clean and fluent api with tuple destructuring.
npm i await-it
yarn add await-it
We'll pretend that we have some kind of Promise
already declared globally, to avoid bulky README file.
// Fake Global Promises
const fakeResolve = Promise.resolve({ name: 'Nenad Novakovic', age: 28 })
const fakeReject = Promise.reject(new Error('Rejection reason...'))
Without TypeScript
import { it } from 'await-it'
(async () => {
const [res, err] = await it(fakeResolve);
console.log(res) // { name: 'Nenad Novakovic', age: 28 }
console.log(err) // null
})()
(async () => {
const [res, err] = await it(fakeReject);
console.log(res) // undefined
console.log(err.message) // Rejection reason...
})
With TypeScript
import { it } from 'await-it'
(async () => {
interface User {
name: string;
age: number;
}
const [res] = await it<User>(fakeResolve);
console.log(res) // { name: 'Nenad Novakovic', age: 28 }
})()
Node Environment
// Import as CommonJS
const { it } = require('await-it')
// Import as ESM
import { it } from 'await-it'
FAQs
A wrapper for async/await calls without the need of try/catch block.
The npm package await-it receives a total of 1 weekly downloads. As such, await-it popularity was classified as not popular.
We found that await-it demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.