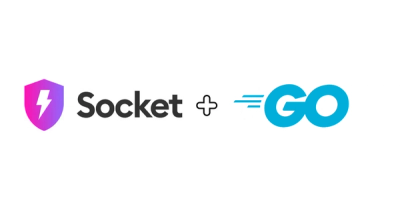
Product
Go Support Is Now Generally Available
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
aws-dynamo-table
Advanced tools
Managing records saved in Dynamodb
I want to save scheduling information
{
recurrence: string,
time: string,
timeZone: string,
mediumType: enum, // SLACK, GMAIL, ...
meta: json // ex: { channelId, slackBotId, slackBotName }
}
Create node package that exposes a DynamoDBService
along with CRUD methods.
const DynamoDBService = require('dynamodb-service');
// profile = 'not-default' // => this is the aws credentials found in `.aws/credentials` file
let dynamoDBService = new DynamoDBService(tableName, primaryKeyName, profile);
dynamoDBService.create(data) // => @return Promise
[default]
aws_access_key_id = your_access_key
aws_secret_access_key = your_secret_key
[not-default]
aws_access_key_id = your_access_key
aws_secret_access_key = your_secret_key
Other ways to provide credentials
aws dynamodb create-table --table-name scheduling-configuration \
--attribute-definitions AttributeName=configurationId,AttributeType=S \
--key-schema AttributeName=configurationId,KeyType=HASH \
--provisioned-throughput ReadCapacityUnits=1,WriteCapacityUnits=1 \
--query TableDescription.TableArn --output text \
--region=us-east-1 --profile default
# the tableName 'scheduling-configuration' can be anything
# note the 'AttributeName', you can change 'configurationId' to whatever you want
The TDD way
mkdir dynamodb-service
cd dynamodb-service && npm init
// follow the prompt and provide all required info
mkdir test
// we first create a test file for create method
// it should take in data and return a Promise that resolve with
// the saved record
// ./test/create.test.js
let DynamoDBService = require('../index.js');
let tableName = 'scheduling-configuration',
primaryKeyName = 'configurationId';
let dynamodbService = new DynamodbService(tableName, primaryKeyName);
let data = {
recurrence: 'string',
time: 'string',
timeZone: 'string',
mediumType: 'SLACK', // SLACK, GMAIL, ...
meta: { channelId: 'string', slackBotId: 'string', slackBotName: 'string' }
};
dynamoDBService.create(data)
.then(createdRecord => console.log(createdRecord))
.catch(e => console.error(e.message || 'error', e));
# you should get errors
node test/create.test.js
index.js
file// ./index.js
'use strict';
const DynamoDBService = require('./src/services/DynamoDBService');
module.exports = DynamoDBService;
DynamoDBService
file# ./src/services/DynamoDBService.js
// we are using es6 classes
// ./src/services/DynamoDBService.js
...
constructor(tableName, primaryKeyName, profile) {
this.tableName = tableName;
this.primaryKeyName = primaryKeyName;
let credentials = new AWS.SharedIniFileCredentials({profile});
AWS.config.credentials = credentials;
this.DYNAMO_DB = new AWS.DynamoDB.DocumentClient();
}
...
// ./src/services/DynamoDBService.js
...
let params = {
TableName: this.tableName,
Item: data
};
return this.dynamoDb.put(params).promise()
.then((/* success */) => this.read(data[this.keyName]));
...
// ./src/services/DynamoDBService.js
...
let Key = {};
Key[this.keyName] = id;
let params = {
TableName: this.tableName,
Key
};
return this.dynamoDb.get(params).promise()
.then(function (response) {
return response.Item;
});
...
FAQs
Managing records saved in Dynamodb
We found that aws-dynamo-table demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
Security News
vlt adds real-time security selectors powered by Socket, enabling developers to query and analyze package risks directly in their dependency graph.
Security News
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.