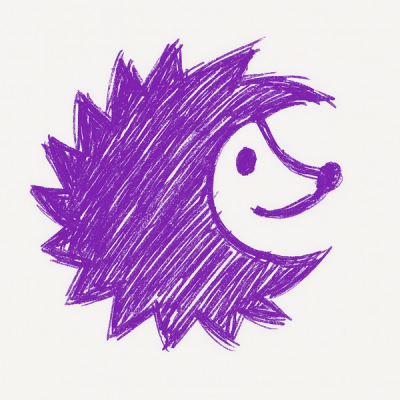
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
aws-lambda
Advanced tools
Deploy AWS Lambda functions from command line using a json or yaml config file.
The aws-lambda npm package provides types and interfaces for AWS Lambda functions, enabling developers to write TypeScript code for AWS Lambda with type safety. It includes type definitions for various AWS services and event sources that can trigger Lambda functions.
APIGatewayProxyHandler
This feature allows you to define a Lambda function that handles API Gateway proxy events. The code sample demonstrates a simple Lambda function that returns a JSON response with a 'Hello, world!' message.
const { APIGatewayProxyHandler } = require('aws-lambda');
const handler: APIGatewayProxyHandler = async (event, context) => {
return {
statusCode: 200,
body: JSON.stringify({ message: 'Hello, world!' })
};
};
exports.handler = handler;
S3Handler
This feature allows you to define a Lambda function that handles S3 events. The code sample demonstrates a Lambda function that logs the bucket name and object key for each record in the S3 event.
const { S3Handler } = require('aws-lambda');
const handler: S3Handler = async (event, context) => {
for (const record of event.Records) {
console.log(`Bucket: ${record.s3.bucket.name}, Key: ${record.s3.object.key}`);
}
};
exports.handler = handler;
DynamoDBStreamHandler
This feature allows you to define a Lambda function that handles DynamoDB stream events. The code sample demonstrates a Lambda function that logs the event ID and event name for each record in the DynamoDB stream event.
const { DynamoDBStreamHandler } = require('aws-lambda');
const handler: DynamoDBStreamHandler = async (event, context) => {
for (const record of event.Records) {
console.log(`Event ID: ${record.eventID}, Event Name: ${record.eventName}`);
}
};
exports.handler = handler;
The serverless package is a framework for building and deploying serverless applications. It supports multiple cloud providers, including AWS, and provides a higher-level abstraction compared to aws-lambda. It includes features for managing infrastructure, deploying functions, and integrating with various services.
The aws-sdk package is the official AWS SDK for JavaScript. It provides a comprehensive set of APIs for interacting with AWS services, including Lambda. While it does not provide type definitions for Lambda events like aws-lambda, it is essential for making API calls to AWS services from your Lambda functions.
The claudia package is a tool for deploying Node.js projects to AWS Lambda and API Gateway. It simplifies the deployment process and provides utilities for managing Lambda functions and API Gateway configurations. It focuses on ease of use and quick deployment, similar to aws-lambda but with additional deployment features.
Command line tool deploy code to AWS Lambda.
Versions prior to 1.0.5 suffer from "Command Injection" vulnerability,
thanks snyk.io and Song Li of Johns Hopkins University for reporting.
npm install -g aws-lambda
WARN: upgrading to v1.0.0 will remove your function environment and layers if they are not defined in the config file
lambda deploy <file.lambda>
credentials needs permissions to CreateFunction, UpdateFunctionConfiguration and UpdateFunctionCodelambda delete <file.lambda>
credentials needs permissions to DeleteFunctionlambda invoke <file.lambda>
credentials needs permissions to InvokeFunction
{
"PATH": "./test-function",
"AWS_KEY": { "Ref" : "env.AWS_ACCESS_KEY_ID" },,
"AWS_SECRET": { "Ref" : "env.AWS_SECRET_ACCESS_KEY"},
"AWS_REGION": "us-east-1",
"FunctionName": "test-lambda",
"Role": "your_amazon_role",
"Runtime": "nodejs10.x",
"Handler": "index.handler",
"MemorySize": "128",
"Timeout": "3",
"Environment": {
"Variables": {
"Hello": "World",
}
},
"Layers": [
"arn:aws:lambda:eu-central-1:452980636694:layer:awspilot-dynamodb-2_0_0-beta:1"
],
"Tags": {
"k1": "v1",
"k2": "v2"
},
"Description": ""
}
# unlike json, comments are allowed in yaml, yey!
# remember to use spaces not tabs 😞
PATH: ./new-function
AWS_KEY: !Ref "env.lambda_deploy_aws_key"
AWS_SECRET: !Ref "env.lambda_deploy_aws_secret"
AWS_REGION: "eu-central-1"
FunctionName: new-function-v12
Role: "arn:aws:iam::452980636694:role/CliLambdaDeploy-TestRole-1H89NZ845HHBK"
Runtime: "nodejs8.10"
Handler: "index.handler"
MemorySize: "128"
Timeout: "3"
Environment:
Variables:
Hello: "World"
Layers:
- "arn:aws:lambda:eu-central-1:452980636694:layer:awspilot-dynamodb-2_0_0-beta:1"
Tags:
k1: v1
k2: v2
Description: ""
// if installed globally then
$ lambda deploy /path/to/my-function.lambda
$ lambda deploy ../configs/my-function.lambda
// if 'npm installed' without the -g then you must use the full path
$ node_modules/.bin/lambda /path/to/my-function.lambda
// you can also add it in your scripts section of your package.json scripts: { "deploy-func1": "lambda deploy ../config/func1.lambda" }
$ npm run deploy-func1
aws-lambda can also watch the config file and the code folder specified in the config.PATH for changes and re-reploy on change
$ lambda start ../configs/my-function.lambda
FAQs
Deploy AWS Lambda functions from command line using a json or yaml config file.
The npm package aws-lambda receives a total of 933,329 weekly downloads. As such, aws-lambda popularity was classified as popular.
We found that aws-lambda demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.