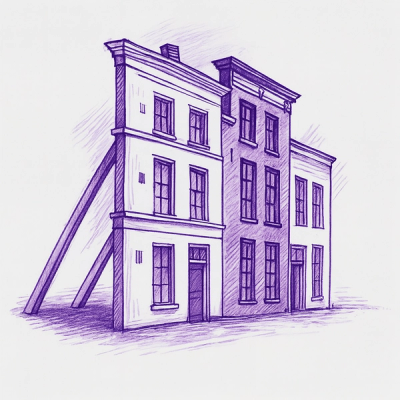
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
aws-secrets-manager-wrapper
Advanced tools
A TypeScript wrapper for AWS Secrets Manager that simplifies common operations and provides a more user-friendly interface.
A TypeScript wrapper for AWS Secrets Manager that simplifies common operations and provides a more user-friendly interface.
npm install aws-secrets-manager-wrapper
import { AWSSecretsManager } from 'aws-secrets-manager-wrapper';
const secretsManager = new AWSSecretsManager({
region: 'us-east-1',
// Optional: Provide credentials if not using environment variables or IAM roles
// accessKeyId: 'YOUR_ACCESS_KEY_ID',
// secretAccessKey: 'YOUR_SECRET_ACCESS_KEY',
});
const secretName = 'my-secret';
const secret = await secretsManager.getSecret(secretName);
console.log(secret);
const secretNames = ['secret1', 'secret2', 'secret3'];
const result = await secretsManager.batchGetSecrets({ secretIds: secretNames });
console.log(result.secrets);
console.log(result.errors);
const secretName = 'new-secret';
const secretValue = { key: 'value' };
const arn = await secretsManager.createSecret(secretName, secretValue);
console.log(`Secret created with ARN: ${arn}`);
const secretName = 'existing-secret';
const newSecretValue = { updatedKey: 'updatedValue' };
const arn = await secretsManager.updateSecret(secretName, newSecretValue);
console.log(`Secret updated with ARN: ${arn}`);
const exists = await secretsManager.secretExists('my-secret');
console.log(`Secret exists: ${exists}`);
const { secretNames, nextToken } = await secretsManager.listSecrets({
maxResults: 100,
filters: [{ Key: 'tag-key', Values: ['environment'] }],
});
console.log(secretNames);
// Add tags
const tagResult = await secretsManager.tagSecret('my-secret', {
environment: 'production',
team: 'backend',
});
console.log(tagResult.message);
// Get tags
const tags = await secretsManager.getTags('my-secret');
console.log(tags); // { environment: 'production', team: 'backend' }
const versions = await secretsManager.getSecretVersions('my-secret');
console.log(versions);
// [{ versionId: 'v1', createdDate: Date, isLatest: true }, ...]
const secretName = 'secret-to-delete';
await secretsManager.deleteSecret(secretName);
console.log(`Secret "${secretName}" deleted`);
AWSSecretsManager
constructor(config: AWSSecretsManagerConfig = {})
config
: Optional configuration object
region
: AWS region (default: 'us-east-1')accessKeyId
: AWS access key IDsecretAccessKey
: AWS secret access keycredentials
: AWS credentials object (alternative to accessKeyId and secretAccessKey)getSecret<T = any>(secretName: string, options?: GetSecretOptions): Promise<T>
batchGetSecrets(options: BatchGetSecretOptions): Promise<BatchGetSecretResult>
createSecret<T = any>(secretName: string, secretValue: T, options?: SecretOptions): Promise<string>
updateSecret<T = any>(secretName: string, secretValue: T, options?: SecretOptions): Promise<string>
deleteSecret(secretName: string, options?: DeleteSecretOptions): Promise<void>
secretExists(secretName: string): Promise<boolean>
listSecrets(options?: ListAllSecretOptions): Promise<{ secretNames: string[]; nextToken?: string }>
tagSecret(secretName: string, tags: Record<string, string>): Promise<{ success: true; message: string }>
getTags(secretName: string): Promise<Record<string, string>>
getSecretVersions(secretName: string): Promise<Array<{ versionId: string; createdDate?: Date; isLatest: boolean }>>
The wrapper uses a custom SecretsManagerError
class for error handling. All methods throw this error type, which includes the original AWS SDK error for reference.
Contributions are welcome! Please feel free to submit a Pull Request.
This project is licensed under the MIT License.
FAQs
A TypeScript wrapper for AWS Secrets Manager that simplifies common operations and provides a more user-friendly interface.
We found that aws-secrets-manager-wrapper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.