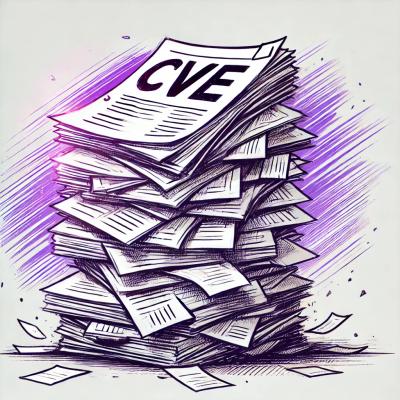
Security News
CISA Rebuffs Funding Concerns as CVE Foundation Draws Criticism
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
azure-keyvault
Advanced tools
This project provides a Node.js package for accessing keys, secrets and certificates on Azure Key Vault. Right now it supports:
npm install azure-keyvault
A sample that can be cloned and run can be found here.
Others you might want to take a look at: Soft delete, recovery, backup and restore Managed storage accounts Deploying certificates to a VM Fetching keyvault secret from a web-application during runtime with MSI
The following are some examples on how to create and consume secrets, certificates and keys. For a complete sample, please check one of the above links.
var KeyVault = require('azure-keyvault');
var msRestAzure = require('ms-rest-azure');
async function main(): Promise<void> {
const credentials = await msRestAzure.interactiveLogin();
// OR const credentials = await msRestAzure.loginWithServicePrincipalSecret("clientId", "secret", "domain");
// OR any other login method from msRestAzure.
const client = new KeyVault.KeyVaultClient(credentials);
}
main();
client.createKey(vaultUri, 'mykey', 'RSA', options).then( (keyBundle) => {
// Encrypt some plain text
return client.encrypt(keyBundle.key.kid, 'RSA-OAEP', "ciphertext");
});
// Create a certificate
client.createCertificate(vaultUri, 'mycertificate', options).then( (certificateOperation) => {
console.log(certificateOperation);
var parsedId = KeyVault.parseCertificateOperationIdentifier(certificateOperation.id);
// Poll the certificate status until it is created
var interval = setInterval( () => {
client.getCertificateOperation(parsedId.vault, parsedId.name).then( (pendingCertificate) => {
if (pendingCertificate.status.toUpperCase() === 'completed'.toUpperCase()) {
clearInterval(interval); // clear our polling function
console.log(pendingCertificate);
var parsedCertId = KeyVault.parseCertificateIdentifier(pendingCertificate.target);
client.deleteCertificate(parsedCertId.vault, parsedCertId.name).then( (deleteResponse) => {
console.log(deleteResponse);
});
}
});
});
});
FAQs
KeyVaultClient Library with typescript type definitions for node
We found that azure-keyvault demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.