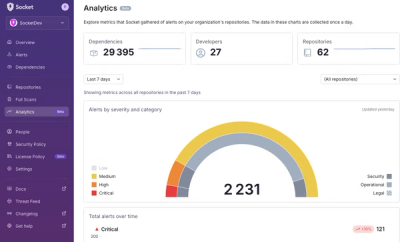
Product
Introducing Historical Analytics – Now in Beta
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
The b64-lite npm package provides lightweight utilities for encoding and decoding base64 strings. It is designed to be a simple and efficient solution for handling base64 operations in JavaScript.
Base64 Encoding
This feature allows you to encode a string into base64 format. The code sample demonstrates how to use the `encode` method from b64-lite to convert a regular string into a base64 encoded string.
const b64 = require('b64-lite');
const encoded = b64.encode('Hello, World!');
console.log(encoded); // Outputs: 'SGVsbG8sIFdvcmxkIQ=='
Base64 Decoding
This feature allows you to decode a base64 encoded string back into its original form. The code sample shows how to use the `decode` method from b64-lite to convert a base64 string back to a regular string.
const b64 = require('b64-lite');
const decoded = b64.decode('SGVsbG8sIFdvcmxkIQ==');
console.log(decoded); // Outputs: 'Hello, World!'
The js-base64 package is a robust solution for base64 encoding and decoding in JavaScript. It offers similar functionality to b64-lite but includes additional features such as URL-safe encoding and support for binary data. Compared to b64-lite, js-base64 might be more suitable for projects requiring more comprehensive base64 handling capabilities.
The base-64 package provides basic utilities for encoding and decoding base64 strings. It is similar to b64-lite in terms of simplicity and ease of use. However, base-64 is a more established package with a larger user base, which might be beneficial for projects that prioritize community support and reliability.
Node, browser, and React Native base64 library
const b64 = require('b64-lite');
// Base64 in ASCII to byte string
b64.atob('aGkgdGhlcmU=');
// hi there
// byte string to Base64 in ASCII
b64.btoa('hi there');
// aGkgdGhlcmU=
// convert unicode to b64
b64.toBase64('hello 你好');
// aGVsbG8g5L2g5aW9
// convert a buffer to b64
b64.toBase64(new Uint8Array([228, 189, 160, 229, 165, 189]).buffer);
// 5L2g5aW9
// decode b64 to unicode
b64.fromBase64('aGVsbG8g5L2g5aW9');
// hello 你好
// convert b64 to a buffer
b64.toBuffer('5L2g5aW9');
// new Uint8Array([228, 189, 160, 229, 165, 189]).buffer
If you use ES6 imports with a bundler that supports tree-shaking, yes!
import { toBase64 } from 'b64-lite'
MIT
FAQs
isomorphic base64 library in 152 bytes
The npm package b64-lite receives a total of 151,171 weekly downloads. As such, b64-lite popularity was classified as popular.
We found that b64-lite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.
Product
Module Reachability filters out unreachable CVEs so you can focus on vulnerabilities that actually matter to your application.
Company News
Socket is bringing best-in-class reachability analysis into the platform — cutting false positives, accelerating triage, and cementing our place as the leader in software supply chain security.