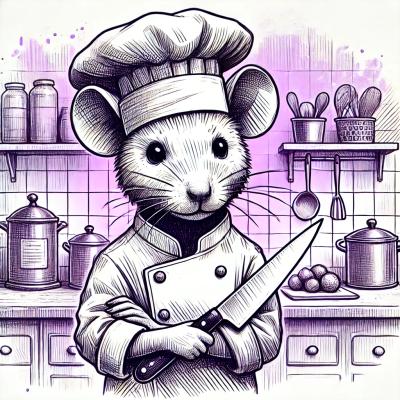
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
babel-compat-loader
Advanced tools
A node loader to import modules compiled by Babel a if they were native ESM.
A node loader to
import
modules compiled by Babel a if they were native ESM.
npm install --save babel-compat-loader
or
yarn add babel-compat-loader
node --experimental-modules --loader babel-compat-loader ./your-file.mjs
ECMAScript modules transpiled by Babel have a "problem": they are not ECMAScript modules anymore. They become CommonJS modules, but this creates an interoperability problem with ECMAScript modules in NodeJS.
Consider this exaple:
// index.mjs
import { val } from "./dependency";
console.log(val);
// dependency.mjs
export const val = 3;
When this program is run using node --experimental-modules index.mjs
it logs 3
, as expected.
When both the files are transpiled by Babel it still logs 3
, because Babel knows how to handle named exports defined in transpiled modules:
// index.js
var _dependency = require("./dependency");
console.log(_dependency.val);
// dependency.js
Object.defineProperty(exports, "__esModule", { value: true });
var val = exports.val = 2;
When only the dependency is transpiled by Babel the program breaks:
// index.mjs
import { val } from "./dependency";
console.log(val);
// dependency.mjs
Object.defineProperty(exports, "__esModule", { value: true });
var val = exports.val = 2;
import { val } from "./dependency";
^^^
SyntaxError: The requested module './dependency' does not provide an export named 'val'
at ModuleJob._instantiate (internal/modules/esm/module_job.js:80:21)
The problem is that, since JavaScript engines need to know which variables are exported by a module before executing it, CommonJS modules only export a default value which represents the module.exports
object.
This loader fixes this problem, by analyzing the imported CommonJS modules before executing them.
FAQs
A node loader to import modules compiled by Babel a if they were native ESM.
The npm package babel-compat-loader receives a total of 0 weekly downloads. As such, babel-compat-loader popularity was classified as not popular.
We found that babel-compat-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.