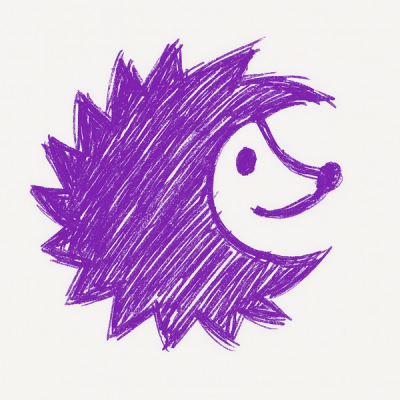
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
backbone-attribute-types
Advanced tools
A non-obtrusive plugin that extends models to force `get()`ing of attributes to return values in a specific type (integer, date, etc).
A non-obtrusive plugin that extends models to force
get()
ing of attributes to return values in a specific type (integer, date, etc).
var TypedModel = Backbone.Model.extend({
attrTypes: {
'count': 'int',
'runtime': 'float',
'created_at': 'moment'
}
});
var typedModel = new TypedModel({
count: '10',
runtime: '4.75',
created_at: '2017-09-19'
})
typedModel.get('count') // 10
typedModel.get('runtime') // 4.75
typedModel.get('created_at') // date object
// a use-case
typedModel.attributes['count'] + 9 // = "109" (this would normally happen)
typedModel.get('count') + 9 // = 19
attrTypes
There are default attribute types under Backbone.ModelAttrTypes
. You can add to this or specify a custom type on the model.
attrTypes can also be defined on the Collection.
window.Backbone.ModelAttrTypes = {
'string': function(val){ return String(val) },
'json': function(val){ return _.isString(val) ? JSON.parse(val) : val },
'bool': function(val){ return !!val },
'int': function(val){ return parseInt(val) },
'float': function(val){ return parseFloat(val) },
'num': function(val){ return parseFloat(val) }, // alias for float
'date': function(val){ return new Date(val) },
// moment.js support
'moment': function(val){ return window.moment ? moment(val) : new Date(val) }
}
var CustomTypedModel = Backbone.Model.extend({
attrTypes: {
'attrName': function(val){
// convert val to type
return val
}
}
});
You can cast an attribute to a type on the fly by appending a pipe |
and the type at the end of the attribute name.
typedModel.get('runtime') // 4.75
typedModel.get('runtime|int') // 4
typedModel.get('runtime|string') // "4.75"
You can also cast as raw
to get the original value stored in attributes
typedModel.get('runtime|raw') // "4.75"
The reason for this plugin is for when your model has an attribute in a string format (for JSON and database consistency) but you wish to use the value in code as a certain type. Rather than 1) continually converting the value to your desired type or 2) creating a secondary method to make this conversion, this plugin will do it for you.
It's designed to be non-obtrusive by refraining from overwriting the real value in attributes
and allows you to continue using .get(attr)
like normal.
v0.1.3
v0.1.2
MIT © Kevin Jantzer
FAQs
A non-obtrusive plugin that extends models to force `get()`ing of attributes to return values in a specific type (integer, date, etc).
The npm package backbone-attribute-types receives a total of 3 weekly downloads. As such, backbone-attribute-types popularity was classified as not popular.
We found that backbone-attribute-types demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.