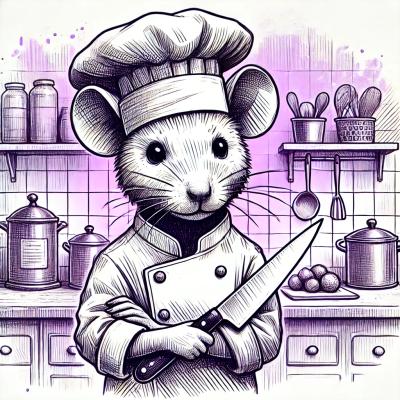
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
bacon-cipher
Advanced tools
A robust JavaScript implementation of Bacon’s cipher, a.k.a. the Baconian cipher.
bacon-cipher is a JavaScript implementation of Bacon’s cipher, a.k.a. the Baconian cipher. It can be used to encode plaintext to Bacon-ciphertext, or the other way around (i.e. decoding).
By default it uses the most common Bacon cipher alphabet, i.e. ABCDEFGHIKLMNOPQRSTUWXYZ
(24 letters). This boils down to the following translations:
a AAAAA g AABBA n ABBAA t BAABA
b AAAAB h AABBB o ABBAB u-v BAABB
c AAABA i-j ABAAA p ABBBA w BABAA
d AAABB k ABAAB q ABBBB x BABAB
e AABAA l ABABA r BAAAA y BABBA
f AABAB m ABABB s BAAAB z BABBB
Via npm:
npm install bacon-cipher
Via Bower:
bower install bacon-cipher
Via Component:
component install mathiasbynens/bacon-cipher
In a browser:
<script src="bacon.js"></script>
In Narwhal, Node.js, and RingoJS:
var bacon = require('bacon-cipher');
In Rhino:
load('bacon.js');
Using an AMD loader like RequireJS:
require(
{
'paths': {
'bacon': 'path/to/bacon'
}
},
['bacon'],
function(bacon) {
console.log(bacon);
}
);
bacon.version
A string representing the semantic version number.
bacon.encode(text, options)
This function takes a string of text (the text
parameter) and encrypts it using Bacon’s cipher.
bacon.encode('steganography');
// → 'BAAABBAABAAABAAAABBAAAAAAABBAAABBABAABBABAAAAAAAAAABBBAAABBBBABBA'
By default it uses the most common Bacon cipher alphabet, i.e. ABCDEFGHIKLMNOPQRSTUWXYZ
(24 letters). In this case instances of J
are replaced with I
, and instances of V
are replaced with U
before further encoding the input string.
bacon.encode('James Vendetta');
// → 'ABAAAAAAAAABABBAABAABAAAB BAABBAABAAABBAAAAABBAABAABAABABAABAAAAAA'
It’s possible to pass an (optional) options
object with an alphabet
property to override the cipher alphabet. Note that in that case, no preprocessing (like replacing j
and v
) is done. For example, to use the full 26-letter alphabet:
bacon.encode('James Vendetta', {
'alphabet': 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
});
// → 'ABAABAAAAAABBAAAABAABAABA BABABAABAAABBABAAABBAABAABAABBBAABBAAAAA'
bacon.decode(text, options)
This function takes a string of text (the text
parameter) and decrypts it using Bacon’s cipher.
By default it uses the most common Bacon cipher alphabet, i.e. ABCDEFGHIKLMNOPQRSTUWXYZ
(24 letters).
bacon.decode('BAAABBAABAAABAAAABBAAAAAAABBAAABBABAABBABAAAAAAAAAABBBAAABBBBABBA');
// → 'STEGANOGRAPHY'
bacon.decode('ABAAAAAAAAABABBAABAABAAAB BAABBAABAAABBAAAAABBAABAABAABABAABAAAAAA');
// → 'IAMES UENDETTA'
It’s possible to pass an (optional) options
object with an alphabet
property to override the cipher alphabet. For example, to use the full 26-letter alphabet:
bacon.decode('ABAABAAAAAABBAAAABAABAABA BABABAABAAABBABAAABBAABAABAABBBAABBAAAAA', {
'alphabet': 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
});
// → 'JAMES VENDETTA'
bacon
binaryTo use the bacon
binary in your shell, simply install bacon-cipher globally using npm:
npm install -g bacon-cipher
After that you will be able to use Bacon’s cipher from the command line:
$ bacon --encode 'foo bar baz'
AABABABBABABBAB AAAABAAAAABAAAA AAAABAAAAABABBB
$ bacon --decode 'AABABABBABABBAB AAAABAAAAABAAAA AAAABAAAAABABBB'
FOO BAR BAZ
$ bacon --encode --alphabet=ABCDEFGHIJKLMNOPQRSTUVWXYZ 'Julius Caesar'
ABAABBABAAABABBABAAABABAABAABA AAABAAAAAAAABAABAABAAAAAABAAAB
$ bacon --decode --alphabet=ABCDEFGHIJKLMNOPQRSTUVWXYZ 'ABAABBABAAABABBABAAABABAABAABA AAABAAAAAAAABAABAABAAAAAABAAAB'
JULIUS CAESAR
Read a local text file, encrypt it using Bacon’s cipher with a non-default cipher alphabet, and save the result to a new file:
$ bacon --encode < foo.txt > foo-bacon.txt
Or do the same with an online text file:
$ curl -sL 'http://mths.be/brh' | bacon --encode > bacon.txt
Or, the opposite — read a local file containing Bacon ciphertext, decode it back to plain text, and save the result to a new file:
$ bacon --decode < bacon.txt > original.txt
See bacon --help
for the full list of options.
bacon is designed to work in at least Node.js v0.10.0, Narwhal 0.3.2, RingoJS 0.8-0.9, PhantomJS 1.9.0, Rhino 1.7RC4, as well as old and modern versions of Chrome, Firefox, Safari, Opera, and Internet Explorer.
After cloning this repository, run npm install
to install the dependencies needed for development and testing. You may want to install Istanbul globally using npm install istanbul -g
.
Once that’s done, you can run the unit tests in Node using npm test
or node tests/tests.js
. To run the tests in Rhino, Ringo, Narwhal, and web browsers as well, use grunt test
.
To generate the code coverage report, use grunt cover
.
Mathias Bynens |
bacon is available under the MIT license.
FAQs
A robust JavaScript implementation of Bacon’s cipher, a.k.a. the Baconian cipher.
We found that bacon-cipher demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.