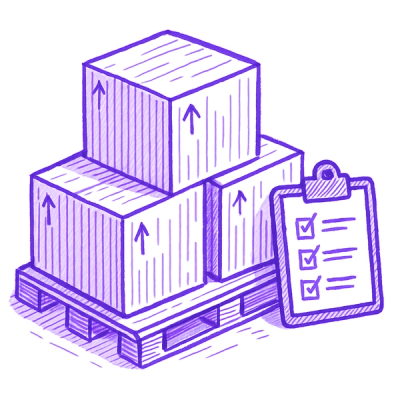
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
base-class-extend
Advanced tools
Base Class constructor for easy class definition - supports getter/setter, inherit/extend Array Error or EventEmitter etc
BaseClass.extend defines class in JavaScript.
This is simple module providing a simple Class function to
simplify class definition in JavaScript.
Supports getter/setter.
Easy to use, easy to inherit/extend.
Also supports inheritance from Array
, Error
, or Node.js events.EventEmitter
.
no difficult keywords,
no prototype
, no __proto__
,
no Object.defineProperty
, no Object.setPrototypeOf
, etc ...
needs constructor
only.
Supports Google Chrome, Mozilla Firefox, Microsoft ie11/10/9/8/7/6 and Node.js/io.js.
$ npm install base-class-extend
or
http://lightspeedworks.github.io/base-class-extend/lib/base-class-extend.js
<script src="http://lightspeedworks.github.io/base-class-extend/lib/base-class-extend.js"></script>
var BaseClass = require('base-class-extend');
Define new class (constructor function) that inherited from Base Class.
var YourClass = BaseClass.extend([name], [proto], [staticProps]);
var YourSubClass = YourClass.extend([name], [proto], [staticProps]);
You have to omit staticProps also, if you omit proto.
You have to specify proto or {}
, if you want to specify staticProps.
The newly defined class (constructor function). (Your class is subclass of BaseClass)
A simple and quick sample:
var BaseClass = require('base-class-extend');
var MyClass = BaseClass.extend({
constructor: function MyClass(value) {
this.value = value; // via setter
},
show: function show() {
console.log(this.value); // via getter
},
// getter
get value() { return this._value; },
// setter
set value(value) {
if (value < 1 || value > 6)
throw new RangeError('Out of range');
this._value = value; },
});
var myObj = new MyClass(5);
myObj.value++; // 5 -> 6
myObj.show();
myObj.value++; // 6 -> 7 throws Error
Create an object, instance of the Class.
var YourClass = BaseClass.extend('YourClass');
var yourObj = YourClass.create();
// or
var yourObj = YourClass.new();
// or
var yourObj = new YourClass();
// or
var yourObj = YourClass();
// required: default constructor or right defined constructor
Your new object, instance of the Class.
var BaseClass = require('base-class-extend').extendPrototype(Object);
// or
// Object.extend = BaseClass.extend;
var SimpleClass = Object.extend('SimpleClass');
// or simply
var SimpleClass = BaseClass.extend.call(Object, 'SimpleClass');
var BaseClass = require('base-class-extend').extendPrototype(Array);
// or
// Array.extend = BaseClass.extend;
var CustomArray = Array.extend('CustomArray');
// or simply
var CustomArray = BaseClass.extend.call(Array, 'CustomArray');
var ca = new CustomArray(1, 2, 3);
// returns [1, 2, 3] like custom array.
var BaseClass = require('base-class-extend').extendPrototype(Error);
// or
// Error.extend = BaseClass.extend;
var CustomError = Error.extend('CustomError');
// or simply
var CustomError = BaseClass.extend.call(Error, 'CustomError');
var ce = new CustomError('message');
var EventEmitter = require('events').EventEmitter;
var BaseClass = require('base-class-extend').extendPrototype(EventEmitter);
// or
// EventEmitter.extend = BaseClass.extend;
var CustomEventEmitter = EventEmitter.extend('CustomEventEmitter');
// or simply
var CustomEventEmitter = BaseClass.extend.call(EventEmitter, 'CustomEventEmitter');
var BaseClass = require('base-class-extend').extendPrototype();
// or
// Function.prototype.extend = BaseClass.extend;
var SimpleClass = Object.extend('SimpleClass');
var CustomArray = Array.extend('CustomArray');
var CustomError = Error.extend('CustomError');
var EventEmitter = require('events').EventEmitter;
var CustomEventEmitter = EventEmitter.extend('CustomEventEmitter');
You can define private variables, hidden variables.
Also support getter/setter, and normal methods to access private variables.
// defined in 'constructor' method or 'new' function
{
constructor: function () {
var private1;
this.addPrototype({
method1: function method1() {
console.log(private1); },
get prop1() { return private1; },
set prop1(val) { private1 = val; },
});
}
}
The prototype object you passed.
Sample:
var YourClass = BaseClass.extend({
constructor: function YourClass() {
var private1 = 123; // access via getter/setter
var private2 = 'abc'; // access via getter, no setter
this.addPrototype({
get private1() { return private1; }, // getter
set private1(val) { private1 = val; }, // setter
get private2() { return private2; }, // getter
});
},
});
You can define private variables, hidden variables.
Also support getter/setter, and normal methods to access private variables.
// defined in 'constructor' method or 'new' function
{
constructor: function () {
var private1;
this.private({
method1: function method1() {
console.log(private1); },
get prop1() { return private1; },
set prop1(val) { private1 = val; },
});
}
}
The prototype object you passed.
Sample:
var YourClass = BaseClass.extend({
constructor: function YourClass() {
var private1 = 123; // access via getter/setter
var private2 = 'abc'; // access via getter, no setter
this.private({
get private1() { return private1; }, // getter
set private1(val) { private1 = val; }, // setter
get private2() { return private2; }, // getter
});
},
});
// Animal
// BaseClass
var BaseClass = require('base-class-extend');
// SimpleClass
var SimpleClass = BaseClass.extend('SimpleClass');
var s1 = new SimpleClass();
// Animal
var Animal = BaseClass.extend({
constructor: function Animal(name) {
if (!(this instanceof Animal))
return Animal.create.apply(Animal, arguments);
BaseClass.apply(this); // or Animal.super_.apply(this);
this.name = name;
},
get name() { return this._name; }, // getter
set name(name) { this._name = name; }, // setter
introduce: function () {
console.log('My name is ' + this.name);
},
}, {
init: function () {
console.log('Animal class init');
},
animalClassMethod: function () {
console.log('Animal class method');
}
}); // -> Animal class init
var a1 = new Animal('Annie');
a1.introduce(); // -> My name is Annie
Animal.animalClassMethod(); // -> Animal class method
// Bear
var Bear = Animal.extend('Bear');
var b1 = Bear('Pooh'); // new less
b1.introduce(); // -> My name is Pooh
var Cat = Animal.extend({
constructor: function Cat() {
if (!(this instanceof Cat))
return Cat.create.apply(Cat, arguments);
Cat.super_.apply(this, arguments);
}
});
var c1 = Cat.create('Kitty');
c1.introduce(); // -> My name is Kitty
var Dog = Animal.extend({
constructor: function Dog() {
if (!(this instanceof Dog))
return Dog.create.apply(Dog, arguments);
Dog.super_.apply(this, arguments);
},
}, {
init: function () {
console.log('Dog class init');
},
dogClassMethod: function () {
this.animalClassMethod();
console.log('Dog class method');
}
}); // -> Dog init
var d1 = Dog.create('Hachi'); // Class method create call
d1.introduce(); // -> My name is Hachi
Dog.dogClassMethod(); // -> Animal class method, Dog class method
Dog.animalClassMethod(); // -> Animal class method
// Vector2D/Vector3D
// BaseClass
var BaseClass = require('base-class-extend');
// sample: JavaScript Object.defineProperty - SONICMOOV LAB
// http://lab.sonicmoov.com/development/javascript-object-defineproperty/
var Vector2D = BaseClass.extend({
constructor: function Vector2D(x, y) {
this._length = 0;
this._changed = true;
this._x = x;
this._y = y;
},
get x() { return this._x; },
set x(x) { this._x = x; this._changed = true; },
get y() { return this._y; },
set y(y) { this._y = y; this._changed = true; },
get length() {
if (this._changed) {
this._length = Math.sqrt(this._x * this._x + this._y * this._y);
this._changed = false;
}
return this._length;
},
set: function (x, y) { this._x = x; this._y = y; this._changed = true; },
});
var v2 = new Vector2D(3, 4);
console.log('V2D(3, 4):', v2.length);
v2.set(1, 2);
console.log('V2D(1, 2):', v2.length);
v2.set(1, 1);
console.log('V2D(1, 1):', v2.length);
var Vector3D = Vector2D.extend({
constructor: function Vector3D(x, y, z) {
Vector2D.call(this, x, y);
this._z = z;
},
get length() {
if (this._changed) {
this._length = Math.sqrt(this._x * this._x + this._y * this._y + this._z * this._z);
this._changed = false;
}
return this._length;
},
set: function (x, y, z) { this._x = x; this._y = y; this._z = z; this._changed = true; },
});
var v3 = new Vector3D(3, 4, 5);
console.log('V3D(3, 4, 5):', v3.length);
MIT
FAQs
Base Class constructor for easy class definition - supports getter/setter, inherit/extend Array Error or EventEmitter etc
The npm package base-class-extend receives a total of 0 weekly downloads. As such, base-class-extend popularity was classified as not popular.
We found that base-class-extend demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.