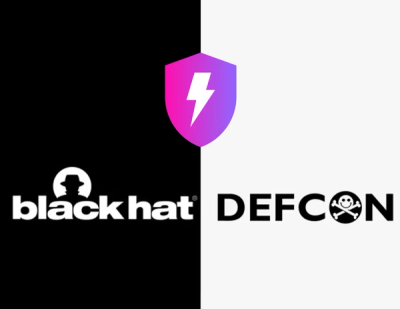
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
base-convert-int-array
Advanced tools
Converts arrays of integers from one base to another. Uses an O(N²) algorithm.
Converts arrays of integers from one base to another. Uses an O(N²) algorithm.
$ npm install base-convert-int-array
First, get an array of integers. For example when converting a value encoded in base 36:
const base36 = Array.from('jld2cyuq0000t3rmniod1foy', char => parseInt(char, 36))
// [ 19, 21, 13, 2, 12, 34, 30, 26, 0, 0, 0, 0, 29, 3, 27, 22, 23, 18, 24, 13, 1, 15, 24, 34 ]
Then, to convert to a byte array:
const baseConvertIntArray = require('base-convert-int-array')
const bytes = baseConvertIntArray(base36, {from: 36, to: 256})
// [ 9, 49, 119, 149, 90, 234, 41, 165, 216, 81, 246, 78, 200, 6, 114, 178 ]
Which you could turn into a buffer:
const buffer = Buffer.from(bytes)
You can specify a fixed length for the resulting array:
const bytes = baseConvertIntArray(base36, {from: 36, to: 256, fixedLength: 16})
A RangeError
will be thrown if the input requires more space than
fixedLength
allows for.
FAQs
Converts arrays of integers from one base to another. Uses an O(N²) algorithm.
The npm package base-convert-int-array receives a total of 220,808 weekly downloads. As such, base-convert-int-array popularity was classified as popular.
We found that base-convert-int-array demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.