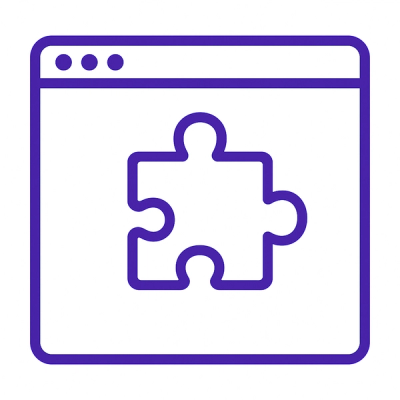
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
base64-arraybuffer
Advanced tools
The base64-arraybuffer npm package provides functionality to encode and decode base64 strings to and from ArrayBuffers, which are a generic, fixed-length raw binary data buffer in JavaScript. It is useful when dealing with binary data in web applications, such as files handling and network communication.
Encode ArrayBuffer to base64
This feature allows you to convert an ArrayBuffer into a base64 encoded string. The code sample demonstrates how to create an ArrayBuffer and encode it to a base64 string using the Encoder class.
const base64 = require('base64-arraybuffer');
const encoder = new base64.Encoder();
const arrayBuffer = new ArrayBuffer(8);
const base64String = encoder.encode(arrayBuffer);
Decode base64 to ArrayBuffer
This feature enables you to convert a base64 encoded string back into an ArrayBuffer. The code sample shows how to decode a base64 string to an ArrayBuffer using the Decoder class.
const base64 = require('base64-arraybuffer');
const decoder = new base64.Decoder();
const base64String = 'someBase64String';
const arrayBuffer = decoder.decode(base64String);
base64-js is a similar package that provides tools for encoding and decoding base64 data. It operates on byte arrays (TypedArray or Array), and it's similar to base64-arraybuffer but does not directly use ArrayBuffers.
The buffer package includes functionality for handling binary data in Node.js. It can be used to convert data to and from base64 and other formats. It is more comprehensive than base64-arraybuffer as it provides a Buffer class that is similar to the global Buffer class in Node.js.
Encode/decode base64 data into ArrayBuffers
You can install the module via npm:
npm install base64-arraybuffer
The library encodes and decodes base64 to and from ArrayBuffers
ArrayBuffer
into base64 stringArrayBuffer
You can run the test suite with:
npm test
Copyright (c) 2012 Niklas von Hertzen Licensed under the MIT license.
FAQs
Encode/decode base64 data into ArrayBuffers
The npm package base64-arraybuffer receives a total of 6,870,802 weekly downloads. As such, base64-arraybuffer popularity was classified as popular.
We found that base64-arraybuffer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.