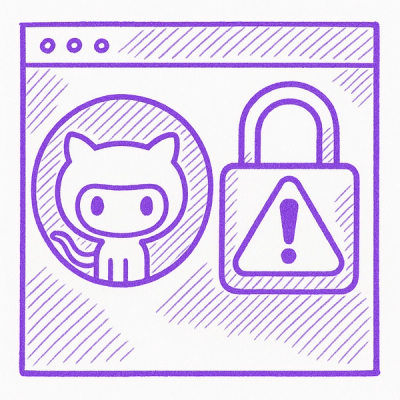
Research
/Security News
Toptal’s GitHub Organization Hijacked: 10 Malicious Packages Published
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
basic-auth-connect
Advanced tools
The basic-auth-connect npm package is a simple middleware for Node.js that provides basic HTTP authentication. It is typically used with the Connect framework or Express.js to protect routes with basic authentication.
Basic Authentication Middleware
This feature allows you to protect routes with basic HTTP authentication. The middleware checks the provided username and password against the specified credentials and grants access if they match.
const connect = require('connect');
const basicAuth = require('basic-auth-connect');
const app = connect();
app.use(basicAuth('username', 'password'));
app.use((req, res) => {
res.end('Authenticated!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
The express-basic-auth package provides similar functionality to basic-auth-connect but is specifically designed for use with Express.js. It offers more configuration options, such as custom challenge messages and multiple users.
The http-auth package is a more comprehensive solution for HTTP authentication in Node.js. It supports basic and digest authentication and can be used with various frameworks, including Express.js and Connect.
The passport-http package is a strategy for Passport.js that provides HTTP Basic and Digest authentication. It is more flexible and can be integrated with other Passport.js strategies for more complex authentication scenarios.
Connect's Basic Auth middleware in its own module. You should consider to create your own middleware with basic-auth.
var basicAuth = require('basic-auth-connect');
Simple username and password
connect()
.use(basicAuth('username', 'password'));
Callback verification
connect()
.use(basicAuth(function(user, pass){
return 'tj' == user && 'wahoo' == pass;
}))
Async callback verification, accepting fn(err, user)
.
connect()
.use(basicAuth(function(user, pass, fn){
User.authenticate({ user: user, pass: pass }, fn);
}))
Security Considerations
Important: When using the callback method, it is recommended to use a time-safe comparison function like crypto.timingSafeEqual to prevent timing attacks.
FAQs
Basic auth middleware for node and connect
We found that basic-auth-connect demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Threat actors hijacked Toptal’s GitHub org, publishing npm packages with malicious payloads that steal tokens and attempt to wipe victim systems.
Research
/Security News
Socket researchers investigate 4 malicious npm and PyPI packages with 56,000+ downloads that install surveillance malware.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.