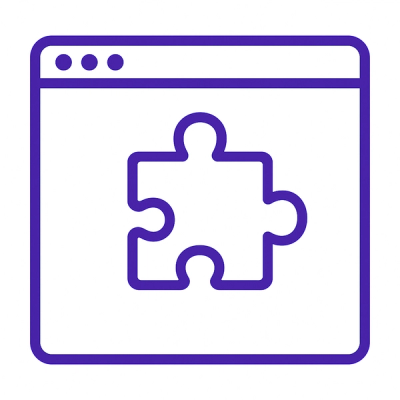
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
A TypeScript Node package that provides a fluent semi-typesafe SQL query builder akin to the one shipped with Laravel. Supports sqlite (using [sqlite3](https://github.com/mapbox/node-sqlite3)), MySQL (using [mysql2](https://github.com/sidorares/node-mysql
Supply Chain Security
Vulnerability
Quality
Maintenance
License
A TypeScript Node package that provides a fluent semi-typesafe SQL query builder akin to the one shipped with Laravel. Supports sqlite (using sqlite3), MySQL (using mysql2) and PostgreSQL (using pg). Find the documentation here.
import Basie, { BaseModel, R, A } from "basie";
class _User extends BaseModel {
public name: string;
public age: number;
public phones: A<Phone> = this.hasMany(Phone);
}
const User = Basie.wrap<_User>()(_User);
type User = _User;
class _Phone extends BaseModel {
public number: string;
public user_id: number;
public user: R<User> = this.belongsTo(User);
}
const Phone = Basie.wrap<_Phone>()(_Phone);
type Phone = _Phone;
// Fluent querying on objects.
const allUsersAbove20 = await User.where("age", ">", 20).get();
// Complex querying supported:
const complex = await User.where("age", ">", 20).orWhere(nested => {
nested.where("name", "LIKE", "%a%");
nested.orWhere("name", "LIKE", "%A%");
}).limit(10).orderByDesc("age").get();
// 'SELECT * FROM users WHERE age > ? OR (name LIKE ? OR name LIKE ?) ORDER BY age DESC LIMIT ?'
// Methods directly on the object.
const user = new User();
user.name = "Thijs";
user.age = 17;
await user.save(); // inserts
user.age = 18;
await user.save(); // updates
await user.delete(); // deletes
// Lazy relationships.
const phones = await user.phones();
console.assert(phones === await user.phones(), "Not lazy");
// Allows relationships to be further narrowed down.
const longPhones = await user.phones.select("LEN(number) as number_length").where("number_length", ">=", 10).get();
// 'SELECT LEN(number) as number_length FROM phones WHERE user_id = ? AND number_length >= ?'
where(nonexistentKey, 10)
will fail, and that where("is_admin", "true")
won't compile if is_admin
is supposed to be a boolean.save()
and delete()
on objects to either insert, update or delete the specific model.For a complete overhead view of all methods available, it is recommended you check out the documentation. The next few sections describe some common usecases and gotchas.
Basie supports SQLite, MySQL and PostgreSQL. Simply import the static Basie instance and call the appropriate method to configure Basie to use that database:
import Basie from "basie";
// SQLite.
import { Database } from "sqlite3";
const db = new Database("path_to_database.db", error => {
if (error) throw new Error(); // handle error.
Basie.sqlite(db);
});
// MySQL
import * as mysql from "mysql2";
const connection = await mysql.createConnection(<any>{
user: databaseUsername,
password: databasePassword,
database: databaseName,
host: databaseHost,
port: databasePort,
decimalNumbers: true // recommended to ensure that you receive numbers as numbers instead of strings
});
Basie.mysql(connection);
// PostgreSQL
import * as pg from "pg";
const pool = new pg.Pool({
user: databaseUsername,
password: databasePassword,
database: databaseName,
host: databaseHost,
port: databasePort,
max: 10,
idleTimeoutMillis: 30000
});
pg.types.setTypeParser(20, parseInt); // convert strings to numbers (int8)
pg.types.setTypeParser(1700, parseFloat); // convert decimal strings to numbers (numeric)
Basie.postgres(pool);
Your models are simple TypeScript classes, which means that they support methods and computed properties. Basie will ensure that whenever a model instance gets loaded from the database, it will have the appropriate prototype.
class _User extends BaseModel {
public name: string;
public lastChangeTimestamp: number;
get lastChange(): Date {
return new Date(this.lastChangeTimestamp);
}
set lastChange(newDate: Date) {
this.lastChangeTimestamp = newDate.getTime();
}
greet() {
console.log("Hello, I'm " + this.name);
}
}
const User = Basie.wrap<_User>()(_User);
type User = _User;
For a full overview of all relations, please check the documentation. Relations work similar to how Laravel's Eloquent handles relations. Do note that foreign keys will need to be present on objects.
import Basie, { BaseModel, A, R } from "basie";
class _User extends BaseModel {
public name: string;
public age: number;
public readonly phones: A<Phone> = this.hasMany(Phone);
}
const User = Basie.wrap<_User>()(_User);
type User = _User;
class _Phone extends BaseModel {
public number: string;
public user_id: number;
public readonly user: R<User> = this.belongsTo(User);
}
const Phone = Basie.wrap<_Phone>()(_Phone);
type Phone = _Phone;
Relations are lazy and return a promise. Calling a relation without ()
will instead return a query builder that can be refined further:
const phones = await user.phones(); // Phone.where("user_id", user.id).all()
const phoneQueryBuilder = user.phones; // Phone.where("user_id", user.id). Can be further refined.
Begin by running npm install
or yarn install
. Use npm run watch
(or yarn watch
) to start the TypeScript compilation service. Tests can be ran using npm test
or yarn test
. Pull requests are welcome! ;)
FAQs
A TypeScript Node package that provides a fluent semi-typesafe SQL query builder akin to the one shipped with Laravel. Supports sqlite (using [sqlite3](https://github.com/mapbox/node-sqlite3)), MySQL (using [mysql2](https://github.com/sidorares/node-mysql
The npm package basie receives a total of 1 weekly downloads. As such, basie popularity was classified as not popular.
We found that basie demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.