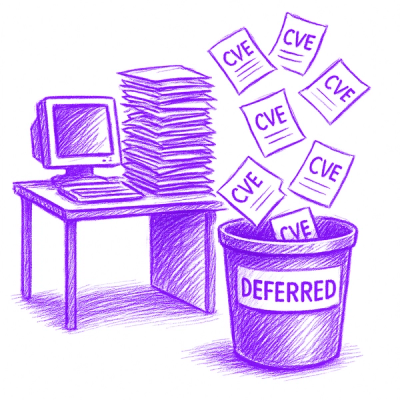
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Optimized bcrypt in TypeSCript with zero dependencies. Compatible to the C++ bcrypt binding on Node.js and also working in the browser.
Besides incorporating a salt to protect against rainbow table attacks, bcrypt is an adaptive function: over time, the iteration count can be increased to make it slower, so it remains resistant to brute-force search attacks even with increasing computation power. (see)
While bcrypt-ts is compatible to the C++ bcrypt binding, it is built by pure JavaScript and thus slower (about 30%), effectively reducing the number of iterations that can be processed in an equal time span.
The maximum input length is 72 bytes (note that UTF-8 encoded characters use up to 4 bytes) and the length of generated
hashes is 60 characters. Note that maximum input length is not implicitly checked by the library for compatibility with
the C++ binding on Node.js, but should be checked with truncates(password)
where necessary.
Install the package:
npm install bcrypt-ts
jsDelivr:
https://cdn.jsdelivr.net/npm/bcrypt-ts/dist/browser.mjs
(ESM)https://cdn.jsdelivr.net/npm/bcrypt-ts/dist/browser.umd.js
(UMD)unpkg:
https://unpkg.com/bcrypt-ts/dist/browser.mjs
(ESM)https://unpkg.com/n/bcrypt-ts/dist/browser.umd.js
(UMD)On Node.js, the inbuilt crypto module's randomBytes interface is used to obtain secure random numbers.
In the browser, bcrypt.js relies on Web Crypto API's getRandomValues interface to obtain secure random numbers. If no cryptographically secure source of randomness is available, the package will throw an error.
If you are using this package in pure Node.js environment, then you will probably use the node bundle.
If you are using bundler like webpack and vite, then you will probably use the browser bundle.
If you meet any issues that a incorrect bundle is used, you can use bcrypt-ts/node
and bcrypt-ts/browser
to force the correct bundle.
To hash a password:
import { genSaltSync, hashSync } from "bcrypt-ts";
const salt = genSaltSync(10);
const result = hashSync("B4c0//", salt);
// Store hash in your password DB
To check a password:
import { compareSync } from "bcrypt-ts";
// Load hash from your password DB
const hash = "xxx";
compareSync("B4c0//", hash); // true
compareSync("not_bacon", hash); // false
Auto-gen a salt and hash at the same time:
import { hashSync } from "bcrypt-ts";
const result = hashSync("bacon", 8);
To hash a password:
import { genSalt, hash } from "bcrypt-ts";
const salt = await genSalt(10);
const result = await hash("B4c0//", salt);
// Store hash in your password DB
To check a password:
import { compare } from "bcrypt-ts";
// Load hash from your password DB
const hash = "xxxxxx";
await bcrypt.compare("B4c0//", hash); // true
await bcrypt.compare("not_bacon", hash); // false
Auto-gen a salt and hash:
import { hash } from "bcrypt-ts";
const result = await bcrypt.hash("B4c0//", 10);
// Store hash in your password DB
Note: Under the hood, asynchronous APIs split an operation into small chunks. After the completion of a chunk, the execution of the next chunk is placed on the back of the JS event queue, efficiently yielding for other computation to execute.
Usage: bcrypt <input> [rounds|salt]
/**
* Synchronously tests a string against a hash.
*
* @param content String to compare
* @param hash Hash to test against
*/
export const compareSync: (content: string, hash: string) => boolean;
/**
* Asynchronously compares the given data against the given hash.
*
* @param content Data to compare
* @param hash Data to be compared to
* @param progressCallback Callback successively called with the percentage of rounds completed
* (0.0 - 1.0), maximally once per `MAX_EXECUTION_TIME = 100` ms.
*/
export const compare: (
content: string,
hash: string,
progressCallback?: ((percent: number) => void) | undefined,
) => Promise<boolean>;
/**
* Synchronously generates a hash for the given string.
*
* @param contentString String to hash
* @param salt Salt length to generate or salt to use, default to 10
* @returns Resulting hash
*/
export const hashSync: (
contentString: string,
salt?: string | number,
) => string;
/**
* Asynchronously generates a hash for the given string.
*
* @param contentString String to hash
* @param salt Salt length to generate or salt to use
* @param progressCallback Callback successively called with the percentage of rounds completed
* (0.0 - 1.0), maximally once per `MAX_EXECUTION_TIME = 100` ms.
*/
export const hash: (
contentString: string,
salt: number | string,
progressCallback?: ((progress: number) => void) | undefined,
) => Promise<string>;
/**
* Gets the number of rounds used to encrypt the specified hash.
*
* @param hash Hash to extract the used number of rounds from
* @returns Number of rounds used
* @throws {Error} If `hash` is not a string
*/
export const getRounds: (hash: string) => number;
/**
* Gets the salt portion from a hash. Does not validate the hash.
*
* @param hash Hash to extract the salt from
* @returns Extracted salt part
* @throws {Error} If `hash` is not a string or otherwise invalid
*/
export const getSalt: (hash: string) => string;
/**
* Synchronously generates a salt.
*
* @param rounds Number of rounds to use, defaults to 10 if omitted
* @returns Resulting salt
* @throws {Error} If a random fallback is required but not set
*/
export const genSaltSync: (rounds?: number) => string;
/**
* Asynchronously generates a salt.
*
* @param rounds Number of rounds to use, defaults to 10 if omitted
*/
export const genSalt: (rounds?: number) => Promise<string>;
Based on bcrypt.js
FAQs
bcrypt written in typescript
The npm package bcrypt-ts receives a total of 16,467 weekly downloads. As such, bcrypt-ts popularity was classified as popular.
We found that bcrypt-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.