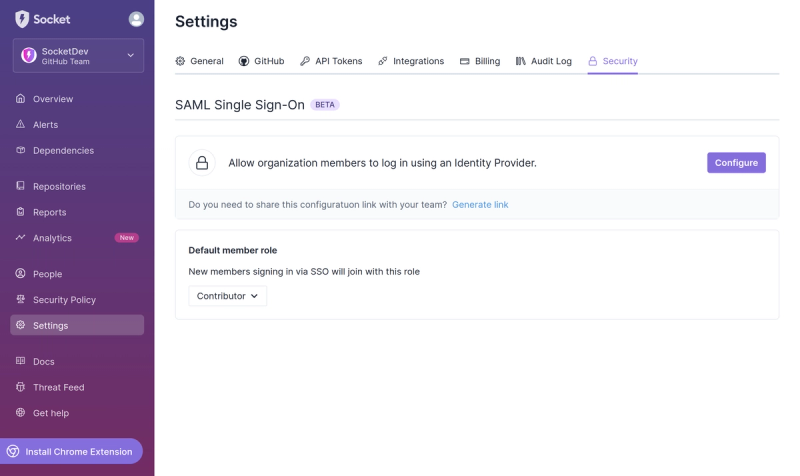
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
bfd-api-redux
Advanced tools
Readme
IMPORTANT: bfd-api-redux
versions 1.2.2 and below are NO LONGER SUPPORTED for interfacing with the Discords.com Bots API.
bfd-api-redux
is an official bot-page API wrapper for Discords.com (formerly botsfordiscord.com). Please direct all inqueries and support requests to our support server.
The current version of the Discords.com Bot API will be depracated upon the release of the BotsV3 runtime (website rewrite). Please keep in mind that any bots using a custom wrapper for the API will need to migrate, the current version if this package will also be deprecated and an update will be required.
Constructing a new API interface:
var bfd = require('bfd-api-redux');
var api = new bfd('BFD_Token', 'botID');
NOTE: If you do now wish to use callbacks, then all actions must be used inside async functions with an await
, for example:
You can easily update your Bot's guild count using this function:
let serverCount = client.guilds.cache.size;
api.setServers(serverCount)
api.getVotes().then(votes => {
console.log(votes)
})
// Or, if running inside an async function:
await api.getVotes()
api.checkVote("254287885585350666").then(vote => { //Provide a user id
console.log(vote) //true or false + vote structure
})
api.checkVoteLight("254287885585350666").then(vote => { //Provide a user id
console.log(vote) //true or false
})
FAQs
A node.js wrapper for the Bots For Discord API
The npm package bfd-api-redux receives a total of 10 weekly downloads. As such, bfd-api-redux popularity was classified as not popular.
We found that bfd-api-redux demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.