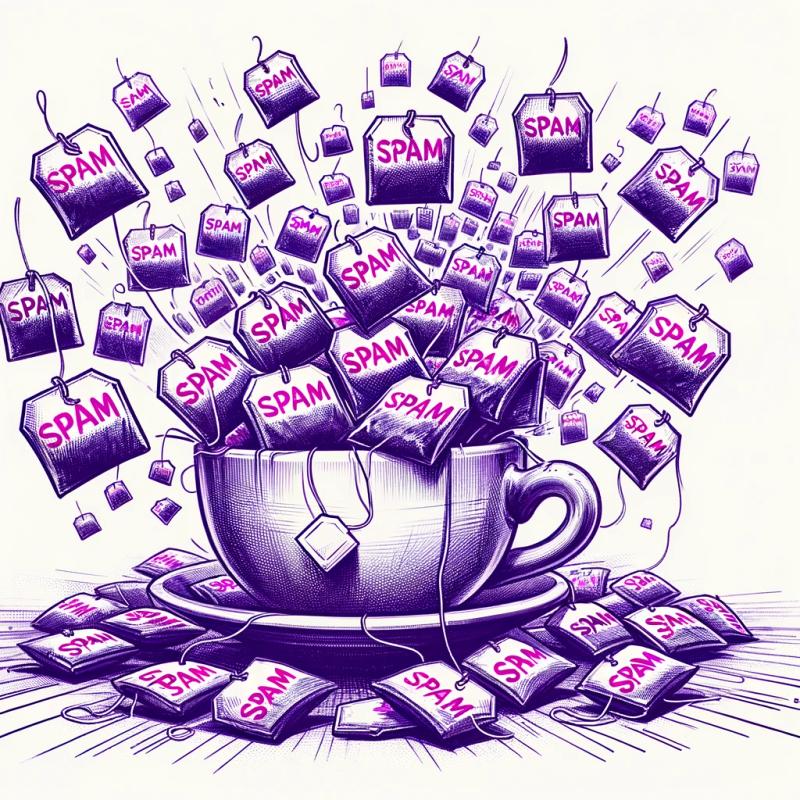
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
bh-property-helpers
Advanced tools
Readme
This is helper for fast and nice property setter/getter creation in context object.
var Method = require('bh-property-helpers');
method = new Method({ protected: false });
funciton Context() {
function pickModsProperty() {
return '_object.' + (this._context.get('object').elem ? 'elemMods' : 'mods');
}
method(this)
.before(function () {
this._object = this._context.get('object');
})
.named('attr').changes('_object.attrs').property()
.named('attrs').changes('_object.attrs').property()
.named('bem').changes('_object.bem').value()
.named('cls').changes('_object.cls').value()
.named('content').changes('_object.content').value()
.named('js').changes('_object.js').value()
.named('param').changes('_object').property()
.named('tag').changes('_object.tag').value()
.named('mix').changes('_object.mix').array()
.named('mod').changes(pickModsProperty).property()
.named('mods').changes(pickModsProperty).object();
}
Constructor of method builder.
Returns Builder
.
Returns builder for context object. All methods will be defined on this object.
Returns Builder
.
Defines name for the method.
Returns Builder
.
Points created method to propertyPath, which can be String
or Function
. In case of Function
property path will be resolved each time method is called.
Returns Builder
.
This methods tells builder about value type, with which created method will be working.
Type | What it does | Setter/getter signature |
---|---|---|
value() | Just sets passed value to property | function (value, force) |
property() | Sets key-value in property object | function (key, value, force) |
array() | Appends passed value to Array property | function (array, force) |
object() | Extends property with passed object | function (object, force) |
By default this setters will not override previous property value (unless force
is true).
Returns Builder
.
FAQs
Helpers for nice property setters/getters creation
The npm package bh-property-helpers receives a total of 0 weekly downloads. As such, bh-property-helpers popularity was classified as not popular.
We found that bh-property-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.