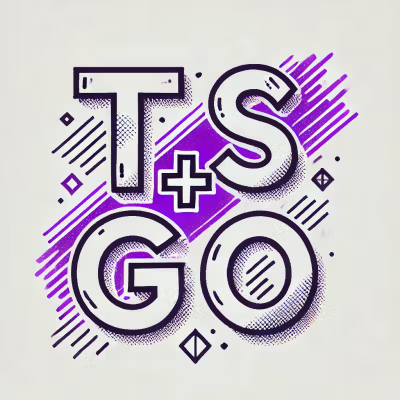
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
bitmap-integers
Advanced tools
Small utility module to convert between integer representations of bitmaps and arrays.
const bitmap = require('bitmap-integers');
You can pass in an array of integers you want to set
bitmap.fromIntegerArray([1]); // 1
bitmap.fromIntegerArray([2]); // 2
bitmap.fromIntegerArray([1,2]); // 3
bitmap.fromIntegerArray([3]); // 4
bitmap.fromIntegerArray([1,3]); // 5
bitmap.fromIntegerArray([2,3]); // 6
bitmap.fromIntegerArray([1,2,3]); // 7
You can pass in an array of booleans in LE form
bitmap.fromBooleanArrayLE([true]); // 1
bitmap.fromBooleanArrayLE([false, true]); // 2
bitmap.fromBooleanArrayLE([true, true]); // 3
bitmap.fromBooleanArrayLE([false, false, true]); // 4
bitmap.fromBooleanArrayLE([true, false, true]); // 5
bitmap.fromBooleanArrayLE([false, true, true]); // 6
bitmap.fromBooleanArrayLE([true, true, true]); // 7
You can pass in an array of booleans in BE form
bitmap.fromBooleanArrayBE([true]); // 1
bitmap.fromBooleanArrayBE([true, false]); // 2
bitmap.fromBooleanArrayBE([true, true]); // 3
bitmap.fromBooleanArrayBE([true, false, false]); // 4
bitmap.fromBooleanArrayBE([true, false, true]); // 5
bitmap.fromBooleanArrayBE([true, true, false]); // 6
bitmap.fromBooleanArrayBE([true, true, true]); // 7
You can get an integer array from a value
bitmap.toIntegerArray(1); // [1]
bitmap.toIntegerArray(2); // [2]
bitmap.toIntegerArray(3); // [1,2]
bitmap.toIntegerArray(4); // [3]
bitmap.toIntegerArray(5); // [1,3]
bitmap.toIntegerArray(6); // [2,3]
bitmap.toIntegerArray(7); // [1,2,3]
You can get an array of booleans in LE form
bitmap.toBooleanArrayLE(1); // [true]
bitmap.toBooleanArrayLE(2); // [false, true]
bitmap.toBooleanArrayLE(3); // [true, true]
bitmap.toBooleanArrayLE(4); // [false, false, true]
bitmap.toBooleanArrayLE(5); // [true, false, true]
bitmap.toBooleanArrayLE(6); // [false, true, true]
bitmap.toBooleanArrayLE(7); // [true, true, true]
You can get an array of booleans in BE form
bitmap.toBooleanArrayBE(1); // [true]
bitmap.toBooleanArrayBE(2); // [true, false]
bitmap.toBooleanArrayBE(3); // [true, true]
bitmap.toBooleanArrayBE(4); // [true, false, false]
bitmap.toBooleanArrayBE(5); // [true, false, true]
bitmap.toBooleanArrayBE(6); // [true, true, false]
bitmap.toBooleanArrayBE(7); // [true, true, true]
npm test
FAQs
Convert integer and boolean arrays to bitmap integers
The npm package bitmap-integers receives a total of 24 weekly downloads. As such, bitmap-integers popularity was classified as not popular.
We found that bitmap-integers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.