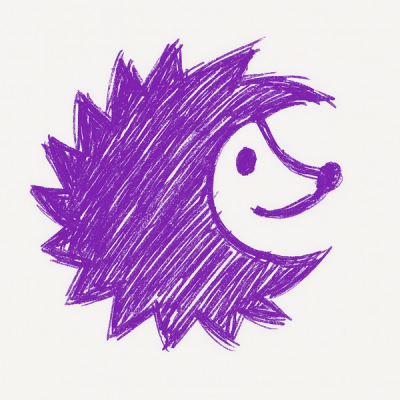
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Redux easy mode. Kill the boilerplate and focus on the logic.
Features simple reducer functions. Blosm automatically takes care of merging a new copy of the changed state. Internally Blosm uses Snoman for native immutable data structures.
npm i blosm
const { createStore } = require('redux')
const blosm = require('blosm')
// init blosm with the initial app state
const db = blosm({
tacos: []
})
// register actions with .on() and its corresponding reducer with .do()
db.on('add-taco')
.get(s => s.tacos)
.do((tacos, data) => tacos.push(data))
// shorthand version
db.on('add-taco', s => s.tacos).do((tacos, data) => tacos.push(data))
// pass the master reducer function to redux
const store = createStore(db.reducer)
Any array prototype methods that would normally mutate the array are proxied and will instead return a new copy of the array with the changes.
Blosm | Redux |
---|---|
const { createStore } = require('redux') const blosm = require('blosm') const db = blosm({ food: { tacos: [] } }) |
const { createStore } = require('redux') const initialState = { food: { tacos: [] } } |
To modify an object, return an object containing the changes. It is also possible to use Snoman object convenience methods.
Blosm | Redux |
---|---|
const { createStore } = require('redux') const blosm = require('blosm') const db = blosm({ food: { favorite: 'pasta', type: 'italian' } }) |
const { createStore } = require('redux') const initialState = { food: { favorite: 'pasta', type: 'italian' } } |
To modify primitives, return the changed value.
Blosm | Redux |
---|---|
const { createStore } = require('redux') const blosm = require('blosm') const db = blosm({ person: { age: 22 } }) |
const { createStore } = require('redux') const initialState = { person: { age: 22 } } |
blosm(initialState)
Returns a new Blosm object to configure state reducers
Object
- Initial state for applicationconst app = blosm({ name: 'lucy', age: 22 })
blosm().on(action)
Registers a new action with the Blosm object
string
- Name of the action typeconst app = blosm({ name: 'lucy', age: 22 })
app.on('birthday')
blosm().on().get(selector)
Specifies a selector to query your object graph. A selector minimizes the need of working with the entire object structure in your reducer. A selector will also be used to construct an object diff for Redux
function
- A function selector querying your object graph. e.g. state => state.food.mexican.tacos
const app = blosm({ name: 'lucy', age: 22 })
app.on('birthday').get(s => s.age)
blosm().on().do(updateReducer)
Specifies the update function to perform on the specified action. In Redux terminology this would the reducer corresponding to a particular action.
function
- A reducer function for the specified action. Whatever is returned from this function will be used to construct a new object for Redux. The updateReducer function receives two parameters:
get()
dispatch({ type: 'birthday', data: 1 })
const app = blosm({ name: 'lucy', age: 22 })
app.on('birthday').get(s => s.age).do((age, data) => age + data)
blosm().reducer
Returns the smart reducer to be passed into Redux createStore
method.
const app = blosm({ name: 'lucy', age: 22 })
app.on('birthday').get(s => s.age).do((age, data) => age + data)
const store = redux.createStore(app.reducer)
Install necessary dependencies
npm i
Run the tests
npm test
Contributions welcome. Submit a Pull Request :)
FAQs
Redux helper
The npm package blosm receives a total of 0 weekly downloads. As such, blosm popularity was classified as not popular.
We found that blosm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.