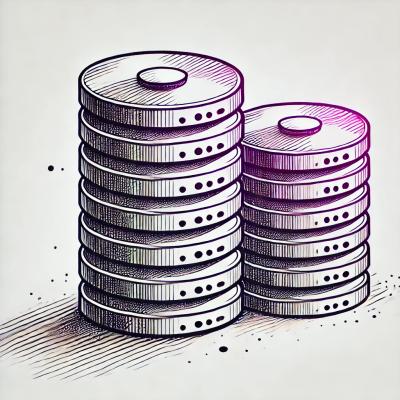
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Encode and parse data in the Concise Binary Object Representation (CBOR) data format (RFC7049).
Assimilate all your JavaScript objects into the Concise Binary Object Representation (CBOR) data format (RFC7049) as fast as possible.
This library is a fork of the awesome node-cbor. It borrows a lot of the interface, but drops all streaming and async processing in favor of a minimal syn api and being as fast as possible.
$ npm install --save borc
TODO
const cbor = require('borc')
const assert = require('assert')
const encoded = cbor.encode(true) // returns <Buffer f5>
const decoded = cbor.decodeFirst(encoded)
// decoded is the unpacked object
assert.ok(decoded === true)
// Use integers as keys
var m = new Map()
m.set(1, 2)
encoded = cbor.encode(m) // <Buffer a1 01 02>
See https://dignifiedquire.github.io/borc for details
The sync encoding and decoding are exported as a
leveldb encoding, as
cbor.leveldb
.
The following types are supported for encoding:
Decoding supports the above types, including the following CBOR tag numbers:
Tag | Generated Type |
---|---|
0 | Date |
1 | Date |
2 | bignumber |
3 | bignumber |
4 | bignumber |
5 | bignumber |
32 | url.URL |
35 | RegExp |
Borc supports custom tags as well as custom input types.
class MyType {
constructor (val) {
this.val = val
}
// Gets called when encoding this object
// gen - instance of the encoder
// obj - the object being encoded
//
// should return true on success and false otherwise
encodeCBOR (gen) {
return gen.pushAny('mytype:' + this.val)
}
}
cbor.encode([new MyType('hello')])
cbor.encode([new cbor.Tagged(42, 'hello')])
const decoder = new cbor.Decoder({
tags: {
42: (val) => val + ' world'
}
})
MIT
FAQs
Encode and parse data in the Concise Binary Object Representation (CBOR) data format (RFC7049).
The npm package borc receives a total of 121,730 weekly downloads. As such, borc popularity was classified as popular.
We found that borc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.