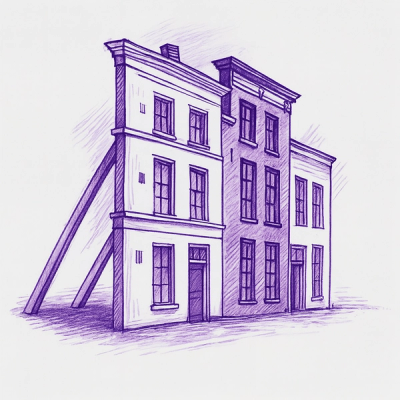
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
botmailroom
Advanced tools
The BotMailroom TypeScript client allows you to interact with the BotMailroom API. It provides asynchronous methods for interacting with the API.
You can get an API key by signing up for a BotMailroom account and creating an API key at https://auth.botmailroom.com/account/api_keys
npm install botmailroom
# or
yarn add botmailroom
import { BotMailRoom } from "botmailroom";
const client = new BotMailRoom("your_api_key"); // or set the BOTMAILROOM_API_KEY environment variable
const inbox = await client.createInbox(
"My Inbox",
"CHANGE_THIS@inbox.botmailroom.com"
);
Unless you have specific allow and block rules that prevent it, you will receive an email from support@inbox.botmailroom.com
after you create an inbox for the first time. If you'd like to send a test email to your inbox, you can do so by:
sendEmail
method:await client.sendEmail(inbox.id, "Test Email", "Hello from BotMailroom!", [
"recipient@example.com",
]);
You can then check for new emails using the getEmails
method:
const emails = await client.getEmails(true, inbox.id);
console.log(emails);
FAQs
TypeScript client for the BotMailRoom API
We found that botmailroom demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.