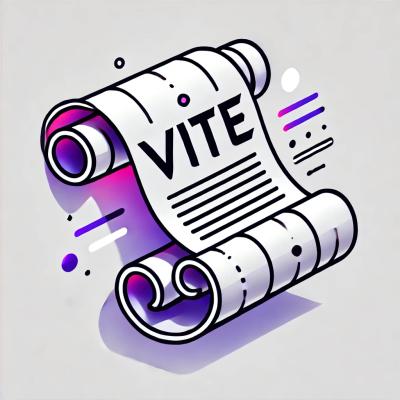
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
box-and-dice-crm
Advanced tools
JavaScript/TypeScript client for the Box and Dice CRM API
A JavaScript/TypeScript client for interacting with the Box+Dice CRM API. This library provides a simple and type-safe way to interact with the Box+Dice CRM system.
Install the package using npm:
npm install box-and-dice-crm
import { BoxDiceClient } from 'box-and-dice-crm';
const client = new BoxDiceClient({
apiKey: 'your-api-key',
domain: 'your-domain.boxdice.com.au'
});
// Get all contacts
const contacts = await client.getContacts();
// Create a new contact
const newContact = await client.createContact({
first_name: 'John',
last_name: 'Doe',
email: 'john@example.com',
mobile: '0400000000'
});
// Update a contact
await client.updateContact(123, {
email: 'newemail@example.com'
});
// Get sales listings
const salesListings = await client.getSalesListings();
// Get rental listings for a specific office
const rentalListings = await client.getRentalListings(officeId);
The client includes custom error types for common API errors:
try {
await client.getContacts();
} catch (error) {
if (error instanceof AuthenticationError) {
// Handle authentication error
} else if (error instanceof RateLimitError) {
// Handle rate limit error
const retryAfter = error.statusCode; // Seconds to wait
} else if (error instanceof BoxDiceError) {
// Handle other API errors
console.error(error.message, error.statusCode);
}
}
For detailed API documentation, please refer to the type definitions in the source code:
MIT License - see LICENSE file for details.
FAQs
JavaScript/TypeScript client for the Box and Dice CRM API
The npm package box-and-dice-crm receives a total of 0 weekly downloads. As such, box-and-dice-crm popularity was classified as not popular.
We found that box-and-dice-crm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.