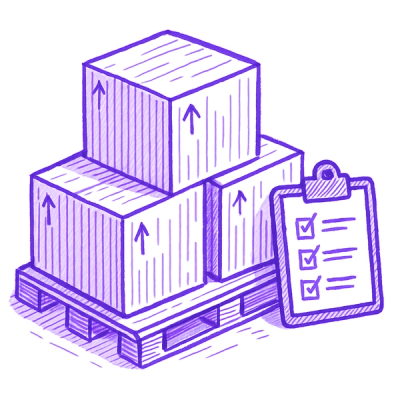
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
The 'bser' npm package is designed to encode and decode Binary Serialized Object Representation (BSER) format. BSER is a binary format used primarily for efficient data serialization and communication, particularly in applications where performance is critical. It is commonly used in conjunction with the Watchman file watching service.
Encoding to BSER
This feature allows you to encode JavaScript objects into BSER format. The example shows how to encode a simple object containing a greeting message.
const bser = require('bser');
const data = { hello: 'world' };
const encoded = bser.dumpToBuffer(data);
console.log(encoded);
Decoding from BSER
This feature enables the decoding of BSER formatted data back into JavaScript objects. The code sample demonstrates decoding a BSER encoded buffer to retrieve the original object.
const bser = require('bser');
const encoded = Buffer.from([0, 1, 3, 11, 1, 5, 104, 101, 108, 108, 111, 2, 5, 119, 111, 114, 108, 100]);
const decoded = bser.loadFromBuffer(encoded);
console.log(decoded);
Protobufjs is a package that provides tools to encode and decode Protocol Buffers, a language-neutral, platform-neutral, extensible mechanism for serializing structured data. It is similar to bser in terms of providing efficient data serialization but uses the Protocol Buffers format, which is more widely adopted and supported across different programming languages.
Msgpack5 is a package for encoding and decoding MessagePack, another efficient binary serialization format. Like bser, it aims for high performance and compact binary size. However, MessagePack is more commonly used and has implementations in various programming languages, making it more versatile for cross-platform applications.
BSER is a binary serialization scheme that can be used as an alternative to JSON. BSER uses a framed encoding that makes it simpler to use to stream a sequence of encoded values.
It is intended to be used for local-IPC only and strings are represented as binary with no specific encoding; this matches the convention employed by most operating system filename storage.
For more details about the serialization scheme see Watchman's docs.
var bser = require('bser');
The is the synchronous decoder; given an input string or buffer, decodes a single value and returns it. Throws an error if the input is invalid.
var obj = bser.loadFromBuffer(buf);
Synchronously encodes a value as BSER.
var encoded = bser.dumpToBuffer(['hello']);
console.log(bser.loadFromBuffer(encoded)); // ['hello']
The asynchronous decoder API is implemented in the BunserBuf object.
You may incrementally append data to this object and it will emit the
decoded values via its value
event.
var bunser = new bser.BunserBuf();
bunser.on('value', function(obj) {
console.log(obj);
});
Then in your socket data
event:
bunser.append(buf);
Read BSER from socket:
var bunser = new bser.BunserBuf();
bunser.on('value', function(obj) {
console.log('data from socket', obj);
});
var socket = net.connect('/socket');
socket.on('data', function(buf) {
bunser.append(buf);
});
Write BSER to socket:
socket.write(bser.dumpToBuffer(obj));
FAQs
JavaScript implementation of the BSER Binary Serialization
The npm package bser receives a total of 24,959,053 weekly downloads. As such, bser popularity was classified as popular.
We found that bser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.