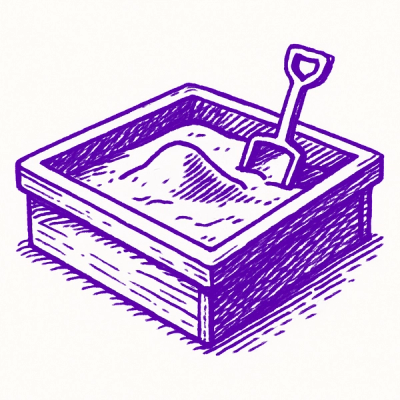
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
The buffer-xor npm package is a utility that allows for the bitwise XOR operation to be performed on two buffers. This operation is useful in various cryptographic functions and data manipulation tasks where you need to combine data in a way that preserves or manipulates information in a reversible manner.
Bitwise XOR operation on buffers
This feature allows you to perform a bitwise XOR operation on two buffers. The code sample demonstrates how to use the buffer-xor package to XOR two hexadecimal buffers and print the result. This is particularly useful in cryptographic operations where XOR is a common operation.
"use strict";\nconst xor = require('buffer-xor');\nlet a = Buffer.from('f00d', 'hex');\nlet b = Buffer.from('dead', 'hex');\nlet output = xor(a, b);\nconsole.log(output.toString('hex')); // Output will be '2f73'
While not directly offering XOR functionality, the 'buffer' package in Node.js can be used in conjunction with custom code to perform similar operations. It provides a way to handle binary data directly with buffers, but you would need to implement the XOR logic manually.
Crypto-js is a collection of cryptographic algorithms implemented in JavaScript, including various operations that can be performed on buffers. While it is more comprehensive and includes a wide range of cryptographic functions beyond XOR, it can be used for similar purposes when working with encryption and data manipulation.
A simple module for bitwise-xor on buffers.
var xor = require('buffer-xor')
var a = new Buffer('00ff0f', 'hex')
var b = new Buffer('f0f0', 'hex')
console.log(xor(a, b))
// => <Buffer f0 0f 0f>
Or for those seeking those few extra cycles, perform the operation in place with
xorInplace
:
NOTE: xorInplace
won't xor past the bounds of the buffer it mutates so make
sure it is long enough!
var xorInplace = require('buffer-xor/inplace')
var a = new Buffer('00ff0f', 'hex')
var b = new Buffer('f0f0', 'hex')
console.log(xorInplace(a, b))
// => <Buffer f0 0f 0f>
// See that a has been mutated
console.log(a)
// => <Buffer f0 0f 0f>
FAQs
A simple module for bitwise-xor on buffers
The npm package buffer-xor receives a total of 11,048,912 weekly downloads. As such, buffer-xor popularity was classified as popular.
We found that buffer-xor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.