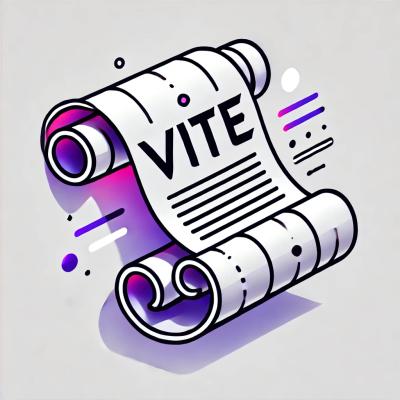
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
cacophony
Advanced tools
Cacophony is a powerful and intuitive audio library designed for modern web applications. It provides a high-level interface to the Web Audio API, simplifying complex audio operations while offering fine-grained control. Cacophony is perfect for projects ranging from simple sound playback to sophisticated audio processing and 3D audio positioning.
AudioBuffer
, URL strings, synthesizers, and live microphone input.npm install cacophony
import { Cacophony } from 'cacophony';
async function audioDemo() {
const cacophony = new Cacophony();
// Create and play a sound with 3D positioning
const sound = await cacophony.createSound('path/to/audio.mp3');
sound.play();
sound.position = [1, 0, -1]; // Set sound position in 3D space
// Create and play a synthesizer
const synth = cacophony.createOscillator({ frequency: 440, type: 'sine' });
synth.play();
// Apply a filter to the synth
const filter = cacophony.createBiquadFilter({ type: 'lowpass', frequency: 1000 });
synth.addFilter(filter);
// Create a group of sounds
const group = await cacophony.createGroupFromUrls(['sound1.mp3', 'sound2.mp3']);
group.play(); // Play all sounds in the group
// Capture microphone input
const micStream = await cacophony.getMicrophoneStream();
micStream.play();
}
audioDemo();
For a comprehensive overview of all classes, methods, and features, please refer to our detailed documentation.
Cacophony provides powerful audio filtering capabilities:
const cacophony = new Cacophony();
// Create a lowpass filter
const lowpassFilter = cacophony.createBiquadFilter({
type: 'lowpass',
frequency: 1000,
Q: 1
});
// Apply filter to a Sound
const sound = await cacophony.createSound('path/to/audio.mp3');
sound.addFilter(lowpassFilter);
sound.play();
// Apply filter to a Synth
const synth = cacophony.createOscillator({ frequency: 440, type: 'sawtooth' });
synth.addFilter(lowpassFilter);
synth.play();
Create and manipulate synthesized sounds with advanced control:
const cacophony = new Cacophony();
// Create a simple sine wave oscillator
const sineOsc = cacophony.createOscillator({ frequency: 440, type: 'sine' });
sineOsc.play();
// Create a complex sound with multiple oscillators
const complexSynth = cacophony.createOscillator({ frequency: 220, type: 'sawtooth' });
const subOsc = cacophony.createOscillator({ frequency: 110, type: 'sine' });
complexSynth.addFilter(cacophony.createBiquadFilter({ type: 'lowpass', frequency: 1000 }));
complexSynth.play();
subOsc.play();
// Modulate frequency over time
let time = 0;
setInterval(() => {
const frequency = 440 + Math.sin(time) * 100;
sineOsc.frequency = frequency;
time += 0.1;
}, 50);
// Apply envelope to volume
complexSynth.volume = 0;
complexSynth.fadeIn(0.5, 'linear');
setTimeout(() => complexSynth.fadeOut(1, 'exponential'), 2000);
Efficiently manage and control multiple sounds or synthesizers:
const cacophony = new Cacophony();
// Create a group of sounds
const soundGroup = await cacophony.createGroupFromUrls(['drum.mp3', 'bass.mp3', 'synth.mp3']);
// Play all sounds in the group
soundGroup.play();
// Control volume for all sounds
soundGroup.volume = 0.7;
// Apply 3D positioning to the entire group
soundGroup.position = [1, 0, -1];
// Create a group of synthesizers
const synthGroup = new SynthGroup();
const synth1 = cacophony.createOscillator({ frequency: 440, type: 'sine' });
const synth2 = cacophony.createOscillator({ frequency: 660, type: 'square' });
synthGroup.addSynth(synth1);
synthGroup.addSynth(synth2);
// Play and control all synths in the group
synthGroup.play();
synthGroup.setVolume(0.5);
synthGroup.stereoPan = -0.3; // Pan slightly to the left
// Remove a synth from the group
synthGroup.removeSynth(synth2);
Capture, process, and manipulate live audio input:
const cacophony = new Cacophony();
async function setupMicrophone() {
try {
const micStream = await cacophony.getMicrophoneStream();
micStream.play();
// Apply a low-pass filter to the microphone input
const lowPassFilter = cacophony.createBiquadFilter({ type: 'lowpass', frequency: 1000 });
micStream.addFilter(lowPassFilter);
// Add a delay effect
const delayFilter = cacophony.createBiquadFilter({ type: 'delay', delayTime: 0.5 });
micStream.addFilter(delayFilter);
// Control microphone volume
micStream.volume = 0.8;
// Pause and resume microphone input
setTimeout(() => {
micStream.pause();
console.log("Microphone paused");
setTimeout(() => {
micStream.resume();
console.log("Microphone resumed");
}, 2000);
}, 5000);
} catch (error) {
console.error("Error accessing microphone:", error);
}
}
setupMicrophone();
Create immersive soundscapes with precise spatial audio control:
const cacophony = new Cacophony();
async function create3DAudioScene() {
// Create sounds with HRTF panning
const ambience = await cacophony.createSound('forest_ambience.mp3', SoundType.Buffer, 'HRTF');
const birdSound = await cacophony.createSound('bird_chirp.mp3', SoundType.Buffer, 'HRTF');
const footsteps = await cacophony.createSound('footsteps.mp3', SoundType.Buffer, 'HRTF');
// Position sounds in 3D space
ambience.position = [0, 0, -5]; // Slightly behind the listener
birdSound.position = [10, 5, 0]; // To the right and above
footsteps.position = [-2, -1, 2]; // Slightly to the left and in front
// Play the sounds
ambience.play();
birdSound.play();
footsteps.play();
// Update listener position and orientation
cacophony.listenerPosition = [0, 0, 0];
cacophony.listenerOrientation = {
forward: [0, 0, -1],
up: [0, 1, 0]
};
// Animate bird sound position
let time = 0;
setInterval(() => {
const x = Math.sin(time) * 10;
birdSound.position = [x, 5, 0];
time += 0.05;
}, 50);
// Change listener position over time
setTimeout(() => {
cacophony.listenerPosition = [0, 0, 5];
console.log("Listener moved forward");
}, 5000);
}
create3DAudioScene();
Stream audio content efficiently:
const cacophony = new Cacophony();
async function streamAudio() {
try {
const streamedSound = await cacophony.createStream('https://example.com/live_radio_stream');
streamedSound.play();
// Apply real-time effects to the stream
const highPassFilter = cacophony.createBiquadFilter({ type: 'highpass', frequency: 500 });
streamedSound.addFilter(highPassFilter);
// Control streaming playback
setTimeout(() => {
streamedSound.pause();
console.log("Stream paused");
setTimeout(() => {
streamedSound.play();
console.log("Stream resumed");
}, 5000);
}, 10000);
} catch (error) {
console.error("Error streaming audio:", error);
}
}
streamAudio();
Cacophony is open-source software licensed under the MIT License.
FAQs
Typescript audio library with caching
The npm package cacophony receives a total of 10 weekly downloads. As such, cacophony popularity was classified as not popular.
We found that cacophony demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.