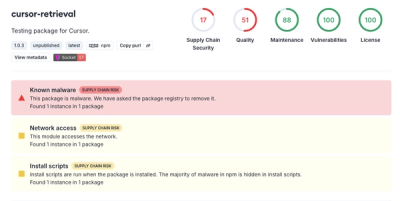
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
The camelize npm package is designed to convert strings (including object keys) from various formats like snake_case, kebab-case, etc., into camelCase. This is particularly useful in JavaScript programming where camelCase is a common convention for naming variables and object keys.
Camelizing strings
Converts a kebab-case or any other non-camelCase string into camelCase. For example, 'my-example-string' would be converted to 'myExampleString'.
"my-example-string".camelize()
Camelizing object keys
Converts all keys in an object from other formats into camelCase. For example, an object with keys 'my-key' and 'another_key' would have its keys converted to 'myKey' and 'anotherKey', respectively.
camelize({ 'my-key': true, 'another_key': 'value' })
Similar to camelize, humps is designed to convert strings and object keys between camelCase and snake_case. It offers more comprehensive options for decamelizing and camelize keys recursively in nested objects, which might make it a more versatile choice for some projects.
Change-case is a more comprehensive string manipulation library that includes functions for transforming strings into various cases, including camel case. It offers a wider range of case transformations compared to camelize, making it suitable for projects that require more than just camelization.
recursively transform key strings to camel-case
var camelize = require('camelize');
var obj = {
fee_fie_foe: 'fum',
beep_boop: [
{ 'abc.xyz': 'mno' },
{ 'foo-bar': 'baz' }
]
};
var res = camelize(obj);
console.log(JSON.stringify(res, null, 2));
output:
{
"feeFieFoe": "fum",
"beepBoop": [
{
"abcXyz": "mno"
},
{
"fooBar": "baz"
}
]
}
var camelize = require('camelize')
Convert the key strings in obj
to camel-case recursively.
With npm do:
npm install camelize
To use in the browser, use browserify.
MIT
v1.0.1 - 2022-10-11
1c978b3
640a9c9
0c27439
c2f99b1
auto-changelog
18083fe
tape
f11a870
funding
in package.json 83d0195
npmignore
to autogenerate an npmignore file 34862da
270fb10
fa51c88
safe-publish-latest
f141183
aud
in posttest
276e8a2
FAQs
recursively transform key strings to camel-case
We found that camelize demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.