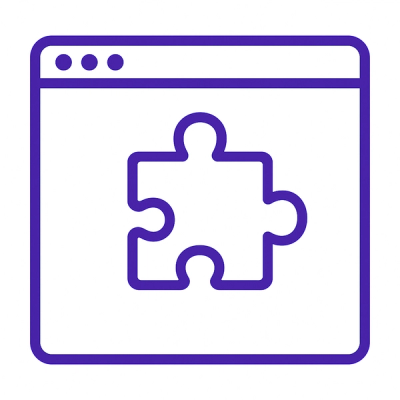
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
captcha-ge is a Node.js library for generating CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) images. It provides both a JavaScript API and a Command-Line Interface (CLI) for easy integration into web applications.
To use captcha-ge in your Node.js project, follow these steps:
Install Node.js:
Install Captcha-Generator:
npm install captcha-ge
#or
npm install -g captcha-ge
You can use the CaptchaGenerator
class to programmatically create CAPTCHA images in your JavaScript code:
import CaptchaGenerator from 'captcha-ge';
// Create an instance of CaptchaGenerator with options
const captchaGenerator = new CaptchaGenerator({
characters: 'ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789',
});
// Generate CAPTCHA image and save it to a file
captchaGenerator.generateImageCaptcha().then((imageBuffer) => {
// Save the image to a file (e.g., captcha.png)
fs.writeFileSync('captcha.png', imageBuffer);
console.log('CAPTCHA image created successfully.');
}).catch((error) => {
console.error('Error creating CAPTCHA:', error);
});
getText()
:The CaptchaGenerator
class also provides a method getText()
to retrieve formatted text from the generated CAPTCHA:
// Generate CAPTCHA text
const captchaText = captchaGenerator.getText();
console.log('Original Text:', captchaText.original);
console.log('Reversed Text:', captchaText.reversed);
console.log('Original UpperCase Text:', captchaText.originalUpperCase);
console.log('Original LowerCase Text:', captchaText.originalLowerCase);
console.log('Reversed UpperCase Text:', captchaText.reversedUpperCase);
console.log('Reversed LowerCase Text:', captchaText.reversedLowerCase);
console.log('Text Length:', captchaText.length);
// ==== OUTPUT ====
// Original Text: OU57A4
// Reversed Text: 4A75UO
// Original UpperCase Text: OU57A4
// Original LowerCase Text: ou57a4
// Reversed UpperCase Text: 4A75UO
// Reversed LowerCase Text: 4a75uo
// Text Length: 6
npm install -g captcha-ge
captcha-ge ./path/to/save/captcha.png
# OUTPUT
# CAPTCHA created successfully: C:\Users\hp\captcha.png
# CAPTCHA text: {
# length: 6,
# original: 'HSQZQB',
# reversed: 'BQZQSH',
# originalUpperCase: 'HSQZQB',
# originalLowerCase: 'hsqzqb',
# reversedUpperCase: 'BQZQSH',
# reversedLowerCase: 'bqzqsh'
# }
You can customize the CAPTCHA generation by providing options to the CaptchaGenerator
constructor, such as characters
Here's an example of how to integrate Captcha-Generator with Express for generating CAPTCHA images on an HTTP endpoint:
import express from 'express';
import CaptchaGenerator from 'captcha-ge';
const app = express();
// Middleware to generate and serve CAPTCHA image
app.get('/captcha', (req, res) => {
const captchaGenerator = new CaptchaGenerator();
captchaGenerator.generateImageCaptcha().then((imageBuffer) => {
res.set({ 'Content-Type': 'image/png' });
res.send(imageBuffer);
}).catch((error) => {
console.error('Error generating CAPTCHA:', error);
res.status(500).send('Error generating CAPTCHA');
});
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
You can integrate Captcha-Generator with a Telegram bot to send CAPTCHA images in a chat:
import TelegramBot from 'node-telegram-bot-api';
import CaptchaGenerator from 'captcha-ge';
const botToken = 'YOUR_TELEGRAM_BOT_TOKEN';
const bot = new TelegramBot(botToken, { polling: true });
bot.onText(/\/captcha/, async (msg) => {
const chatId = msg.chat.id;
const captchaGenerator = new CaptchaGenerator();
const imageBuffer = await captchaGenerator.generateImageCaptcha();
bot.sendPhoto(chatId, imageBuffer, { caption: 'Please solve this CAPTCHA:' })
.catch((error) => {
console.error('Error sending CAPTCHA:', error);
bot.sendMessage(chatId, 'Failed to send CAPTCHA. Please try again later.');
});
});
Captcha-Generator relies on the canvas
library for image generation. Make sure to install all dependencies (node-canvas
and its dependencies) for your environment.
FAQs
Unknown package
We found that captcha-ge demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.