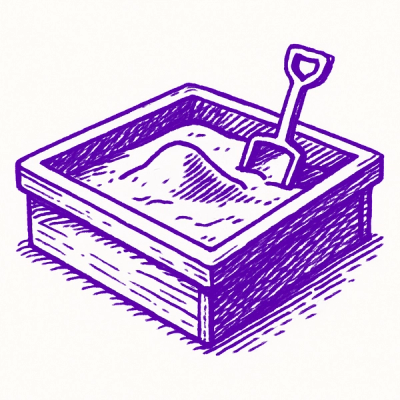
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
A node wrapper for carrot's staff api.
Note: This project is in early development, and versioning is a little different. Read this for more details.
npm i carrot-api --save
All endpoints return a promise - callbacks are not supported because they are just worse in every way. If you are not familiar with promises, here's how to use a promise rather than a callback:
// callback
method('param', function(err, data){
if (err) { return console.error(err); }
console.log('callback!');
});
// promise
method('param')
.catch(console.error)
.then(console.log);
Very simple switch. You can do a lot more complex and wonderful things with promises, but if you don't want to deal with that, just use them as demonstrated above and they are essentially the same thing as callbacks, just with very slightly different syntax. Ok, on to the endpoints!
Returns a full array of all carrot employees and all info that is publicy available for each employees.
Example Usage:
var api = require('carrot-api');
api.staff().then(console.log);
Example Return:
[
{
id: "2",
employee_id: "1",
display_order: "2",
name: "Mike Germano",
title: "CEO & Co-Founder",
department: "Operations & Management",
office: "brooklyn",
slug: "mike",
bio: "Mike makes the impossible happen every day; from his 2005 political campaign making him one of the youngest elected officials in the country to co-founding Carrot to becoming Chief Digital Officer at VICE. Tasked with guiding brands on social and in digital, he is always after adventure. Mike lives in Brooklyn with his wife, son, and French bulldog.",
social_links: {
twitter: "http://twitter.com/mikegermano",
facebook: "http://facebook.com/mikegermano",
tumblr: "http://mikegermano.tumblr.com",
instagram: "http://instagram.com/mikegermano",
pinterest: "http://pinterest.com/mikegermano",
linkedin: "http://linkedin.com/in/mikegermano",
foursquare: "http://foursqare.com/mikegermano"
},
media_avatar: "http://api.bycarrot.com/media/avatars/2.jpg",
media_couch_photo: "http://api.bycarrot.com/media/couch_photos/2.jpg",
media_svg: "http://api.bycarrot.com/media/svgs/2.svg",
commute_method: "Walk",
tattoos: "2",
personal_stat_name: "Times Found Guilty",
personal_stat_value: "0",
home_region: "NJ",
living_region: "Brooklyn",
pets: {
count: "1",
type: "dog"
}
}
// other employees follow the same format
]
Returns a single carrot employee, found by their slug or id, which can be passed in as a string or integer.
Parameters:
id
: Employee slug as a string, or id as an integer or string.Example Usage:
var api = require('carrot-api');
api.staff.find({ id: 'kyle' }).then(console.log);
Example Response:
{
id: "6",
employee_id: "6",
display_order: "4",
name: "Kyle MacDonald",
title: "Chief Technology Officer",
department: "Development",
office: "brooklyn",
slug: "kyle",
bio: "Kyle messed around and got a triple-double",
social_links: {
twitter: "http://twitter.com/kylemac",
linkedin: "http://linkedin.com/pub/kyle-macdonald/27/439/490",
github: "http://github.com/kylemac"
},
media_avatar: "http://api.bycarrot.com/media/avatars/6.jpg",
media_couch_photo: "http://api.bycarrot.com/media/couch_photos/6.jpg",
media_svg: "http://api.bycarrot.com/media/svgs/6.svg",
commute_method: "Walk",
tattoos: null,
personal_stat_name: "Metal pieces in body",
personal_stat_value: "2",
home_region: null,
living_region: "Brooklyn",
pets: {
count: 0,
type: "plants"
}
}
Returns the selected employee's carrot avatar image.
Parameters:
id
: Employee slug as a string, or id as an integer or string.size
: A single value for the width and height, or an array containing width followed by height. All values are in pixels, the default is 200
.format
: Either jpg
or svg
. The default is jpg
.Example Usage:
var api = require('carrot-api');
api.staff.avatar({ id: 'noah', size: 400, format: 'svg' }).then(console.log);
Example Response:
{ url: 'http://api.bycarrot.com/staff/noah/avatar/svg/400' }
Returns the carrot couch photo for the selected user.
Parameters:
id
: Employee slug as a string, or id as an integer or string.Example Usage:
var api = require('carrot-api');
api.staff.couchPhoto({ id: 'kevinpruett' }).then(console.log);
Example Response:
{ url: 'http://api.bycarrot.com/media/couch_photos/27.jpg' }
Returns all carrot staff grouped by department, and ordered by position within each department.
Example Usage:
var api = require('carrot-api');
api.departments().then(console.log);
Example Return:
[
{
name: "Strategy",
staff: [
{
id: "38",
employee_id: "63",
display_order: "10",
name: "RG Logan",
title: "Director of Strategy",
department: "Strategy",
slug: "rg",
bio: "R.G. likes cheap sunglasses, expensive whisky (without the "e") and understanding what makes people tick. He loves it when a plan comes together. When R.G.'s not telling brand stories, he's on the slopes shredding or begging the football gods for a NY Jets Super Bowl.",
social_links: {
twitter: "http://twitter.com/rglogan",
facebook: "http://facebook.com/loganrg",
tumblr: "http://whoisrg.tumblr.com",
instagram: "http://instagram.com/rglogan",
linkedin: "http://linkedin.com/in/rglogan",
foursquare: "http://foursquare.com/rglogan"
},
media_avatar: "http://api.bycarrot.com/media/avatars/38.jpg",
media_couch_photo: "http://api.bycarrot.com/media/couch_photos/38.jpg",
media_svg: "http://api.bycarrot.com/media/svgs/38.svg",
personal_stat_name: "How I keep it",
personal_stat_value: "100"
}
// other staff follow the same format
]
}
// other departments follow the same format
]
Returns all of carrot's committees, subcommittees, and members of each.
Example Usage:
var api = require('carrot-api');
api.committees().then(console.log);
Example Response:
[
{
id: "9",
owning_committee_id: null,
display_order: "2",
name: "Awards Committee",
description: "Creates and awards recognition and prizes to outstanding Carrots. <br/>Plans and implements Carrot's own version of <a href=\"http://en.wikipedia.org/wiki/The_Dundies\" target=\"_blank\">The Dundies</a> - the TOATEMs. <br/>Awards can range from serious honors to silly mentions. <br/>Works with other committees to help them present appropriate awards at events and parties. <br/>Ensures the rules and integrity of the Carrot Cup are being followed and assists in its presentation.",
quote: null,
subcommittees: [ ],
chairs: [
{
id: "12",
employee_id: "27",
name: "Daniela Asaro",
title: "Senior Producer",
slug: "daniela",
media_avatar: "http://api.bycarrot.com/media/avatars/12.jpg",
media_svg: "http://api.bycarrot.com/media/svgs/12.svg",
media_couch_photo: "http://api.bycarrot.com/media/couch_photos/na.jpg"
}
],
members: [
{
id: "33",
employee_id: "56",
name: "Kaitlin Vignali",
title: "QA Manager",
slug: "kaitlinvignali",
media_avatar: "http://api.bycarrot.com/media/avatars/33.jpg",
media_svg: "http://api.bycarrot.com/media/svgs/33.svg",
media_couch_photo: "http://api.bycarrot.com/media/couch_photos/na.jpg"
}
]
}
// other committees follow the same format
]
FAQs
A node wrapper for carrot's company api
The npm package carrot-api receives a total of 0 weekly downloads. As such, carrot-api popularity was classified as not popular.
We found that carrot-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.