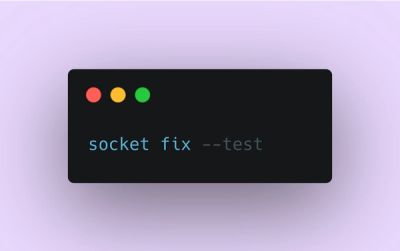
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Cherry3 is a SQlite And Postgre object modeling tool designed to work in an asynchronous environment.
Using npm:
$ npm install cherry3
$ npm install sqlite3 -g # ( For SQlite )
$ npm install pg -g # ( For PostgreSQL )
Other Installations:
$ yarn add cherry3
$ pnpm add cherry3
import { Model, Schema, Types } from 'cherry3';
/* const { Model, Schema, Types } = require('cherry3'); For CommonJS */
// Define a schema for a collection
const userSchema = Schema({
username: { type: Types.String, default: 'fivesobes' },
age: Types.Number,
email: { type: Types.String, default: "support@luppux.com" },
isAdmin: Types.Boolean,
interests: Types.Array,
balance: Types.Float,
date: Types.Date
});
// Create a model for the "users" collection
const UserModel = new Model('Users', userSchema);
// Example data
const userData = {
username: 'fivesobes',
age: 20,
email: 'support@luppux.com',
isAdmin: false,
interests: ['coding', 'reading', 'ertus-mom'],
};
(async () => {
// CRUD operations
try {
/* FIND USERS */
// Find multiple users with conditions
const users = await UserModel.find({ age: 20, isAdmin: false });
console.log(users);
} catch (error) {
console.error('Error performing CRUD operations:', error.message);
}
})();
import { Types } from 'cherry3';
Types.Number // Example => 5
Types.Object // Example => { test:"data text", test3: 5 }
Types.Date // Example => 1.03.2024
Types.String // Example => "test data text"
Types.Boolean // Example => true or false
Types.Array // Example => [ "five" , "so" , 5 ]
Cherry3 is licensed under the GPL 3.0 License. See the LICENSE file for details.
Version 5.2.0
FAQs
Cherry3 is a SQlite And Postgre object modeling tool designed to work in an asynchronous environment.
The npm package cherry3 receives a total of 26 weekly downloads. As such, cherry3 popularity was classified as not popular.
We found that cherry3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.