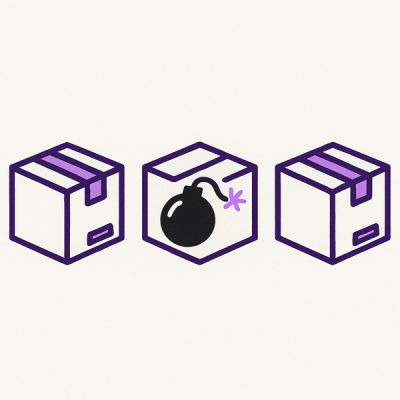
Research
Security News
The Landscape of Malicious Open Source Packages: 2025 Mid‑Year Threat Report
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
class-validator-jsonschema
Advanced tools
Convert class-validator-decorated classes into JSON schema
Convert class-validator-decorated classes into OpenAPI-compatible JSON Schema. The aim is to provide a best-effort conversion: since some of the class-validator
decorators lack a direct JSON Schema counterpart, the conversion is bound to be somewhat opinionated. To account for this multiple extension points are available.
npm install class-validator-jsonschema
Note the peer dependency versions in package.json. Try installing a previous major version of class-validator-jsonschema
in case you're stuck with older peer dependencies.
import { IsOptional, IsString, MaxLength } from 'class-validator'
import { validationMetadatasToSchemas } from 'class-validator-jsonschema'
class BlogPost {
@IsString() id: string
@IsOptional()
@MaxLength(20, { each: true })
tags: string[]
}
const schemas = validationMetadatasToSchemas()
console.log(schemas)
which prints out:
{
"BlogPost": {
"properties": {
"id": {
"type": "string"
},
"tags": {
"items": {
"maxLength": 20,
"type": "string"
},
"type": "array"
}
},
"required": ["id"],
"type": "object"
}
}
validationMetadatasToSchemas
takes an options
object as an optional parameter. Check available configuration objects and defaults at options.ts
.
With options.additionalConverters
you can add new validation metadata converters or override the existing ones. Let's say we want to, for example, add a handy description
field to each @IsString()
-decorated property:
import { ValidationTypes } from 'class-validator'
// ...
const schemas = validationMetadatasToSchemas({
additionalConverters: {
[ValidationTypes.IS_STRING]: {
description: 'A string value',
type: 'string',
},
},
})
which now outputs:
{
"BlogPost": {
"properties": {
"id": {
"description": "A string value",
"type": "string"
}
// ...
}
}
}
An additional converter can also be supplied in form of a function that receives the validation metadata item and global options, outputting a JSON Schema property object (see below for usage):
type SchemaConverter = (
meta: ValidationMetadata,
options: IOptions
) => SchemaObject | void
class-validator
allows you to define custom validation classes. You might for example validate that a string's length is between given two values:
import {
Validate,
ValidationArguments,
ValidatorConstraint,
ValidatorConstraintInterface,
} from 'class-validator'
// Implementing the validator:
@ValidatorConstraint()
export class CustomTextLength implements ValidatorConstraintInterface {
validate(text: string, validationArguments: ValidationArguments) {
const [min, max] = validationArguments.constraints
return text.length >= min && text.length <= max
}
}
// ...and putting it to use:
class Post {
@Validate(CustomTextLength, [0, 11])
title: string
}
Now to handle your custom validator's JSON Schema conversion include a CustomTextLength
converter in options.additionalConverters
:
const schemas = validationMetadatasToSchemas({
additionalConverters: {
CustomTextLength: (meta) => ({
maxLength: meta.constraints[1],
minLength: meta.constraints[0],
type: 'string',
}),
},
})
Validation classes can also be supplemented with the JSONSchema
decorator. JSONSchema
can be applied both to classes and individual properties; any given keywords are then merged into the JSON Schema derived from class-validator decorators:
import { JSONSchema } from 'class-validator-jsonschema'
@JSONSchema({
description: 'A User object',
example: { id: '123' },
})
class BlogPost {
@IsString()
@JSONSchema({
description: 'User primary key',
format: 'custom-id',
})
id: string
}
Results in the following schema:
{
"BlogPost": {
"description": "A User object",
"example": { "id": "123" },
"properties": {
"id": {
"description": "User primary key",
"format": "custom-id",
"type": "string"
}
},
"required": ["id"],
"type": "object"
}
}
JSONSchema
decorators also flow down from parent classes into inherited validation decorators. Note though that if the inherited class uses JSONSchema
to redecorate a property from the parent class, the parent class JSONSchema
gets overwritten - i.e. there's no merging logic.
Alternatively JSONSchema
can take a function of type (existingSchema: SchemaObject, options: IOptions) => SchemaObject
. The return value of this function is then not automatically merged into existing schema (i.e. the one derived from class-validator
decorators). Instead you can handle merging yourself in whichever way is preferred, the idea being that removal of existing keywords and other more complex overwrite scenarios can be implemented here.
class-validator
supports validating nested objects via the @ValidateNested
decorator. Likewise JSON Schema generation is supported out-of-the-box for nested properties such as
@ValidateNested()
user: UserClass
However, due to limitations in Typescript's reflection system we cannot infer the inner type of a generic class. In effect this means that properties like
@ValidateNested({ each: true })
users: UserClass[]
@ValidateNested()
user: Promise<UserClass>
would resolve to classes Array
and Promise
in JSON Schema. To work around this limitation we can use @Type
from class-transformer
to explicitly define the nested property's inner type:
import { Type } from 'class-transformer'
import { validationMetadatasToSchemas } from 'class-validator-jsonschema'
const { defaultMetadataStorage } = require('class-transformer/cjs/storage') // See https://github.com/typestack/class-transformer/issues/563 for alternatives
class User {
@ValidateNested({ each: true })
@Type(() => BlogPost) // 1) Explicitly define the nested property type
blogPosts: BlogPost[]
}
const schemas = validationMetadatasToSchemas({
classTransformerMetadataStorage: defaultMetadataStorage, // 2) Define class-transformer metadata in options
})
Note also how the classTransformerMetadataStorage
option has to be defined for @Type
decorator to take effect.
Under the hood we grab validation metadata from the default storage returned by class-validator
's getMetadataStorage()
. In case of a version clash or something you might want to manually pass in the storage:
const schemas = validationMetadatasToSchemas({
classValidatorMetadataStorage: myCustomMetadataStorage,
})
There's no handling for class-validator
s validation groups or conditional decorator (@ValidateIf
) out-of-the-box. The above-mentioned extension methods can be used to fill the gaps if necessary.
The OpenAPI spec doesn't currently support the new JSON Schema draft-06 keywords const
and contains
. This means that constant value decorators such as @IsEqual()
and @ArrayContains()
translate to quite complicated schemas. Hopefully in a not too distant future these keywords are adopted into the spec and we'll be able to provide neater conversion.
Handling null values is also tricky since OpenAPI doesn't support JSON Schema's type: null
, providing its own nullable
keyword instead. The default @IsEmpty()
converter for example opts for nullable
but you can use type: null
instead via options.additionalConverters
:
// ...
additionalConverters: {
[ValidationTypes.IS_EMPTY]: {
anyOf: [
{type: 'string', enum: ['']},
{type: 'null'}
]
}
}
skipMissingProperties
and @isDefined()
A Base64-encoded string
)FAQs
Convert class-validator-decorated classes into JSON schema
The npm package class-validator-jsonschema receives a total of 88,708 weekly downloads. As such, class-validator-jsonschema popularity was classified as popular.
We found that class-validator-jsonschema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.