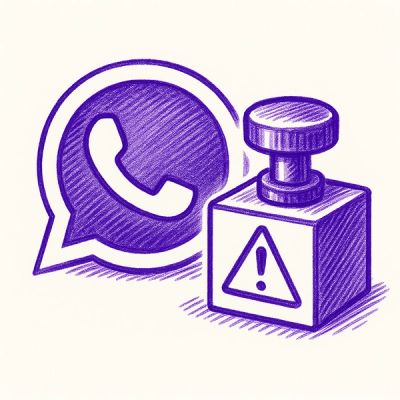
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
classcharts-api
Advanced tools
A Typescript wrapper for getting information from the ClassCharts API
A Node.js and Deno wrapper for getting information from the Classcharts API.
Source • Issues • NPM • Deno • Discord • Library Docs • API Docs
The ClassCharts API is a typescript wrapper around the ClassCharts API. It allows you to easily make requests to the ClassCharts API without having to worry about the underlying implementation of making requests.
For any help with the library, please join the discord where you can ask questions and get help from the community.
Contributions are welcome! There are lots of API endpoints which we simply do not have access to to implement, so if you have access to them, please consider contributing! If you have any questions, feel free to ask in the discord.
npm install classcharts-api
The imports in the examples are for Node.js, but can easily be substituted for Deno.
import {
ParentClient,
StudentClient,
} from "https://deno.land/x/classcharts_api/mod.ts";
Before making any requests, you must login to the client. This will get you a session ID which will be used for all requests.
import { StudentClient } from "classcharts-api";
// Date of birth MUST in the format DD/MM/YYYY
const client = new StudentClient("classchartsCode", "01/01/2000");
await client.login();
?> The parent client is not tested, as I do not have access to a parent account. If you experience any issues, please open an issue or PR.
import { ParentClient } from "classcharts-api";
const client = new ParentClient("username", "password");
await client.login();
All the following methods can be used on both the student and parent client. Each example expects the client to be already logged in.
.getStudentInfo
const studentInfo = await client.getStudentInfo();
console.log(studentInfo);
/**
{
success: 1,
data: {
user: {
id: 2339528,
...
}
},
meta: {
session_id: '5vf2v7n5uk9jftrxaarrik39vk6yjm48',
...
}
}
*/
.getActivity
// Dates must be in format YYYY-MM-DD
const activity = await client.getActivity({
from: "2023-04-01",
to: "2023-05-10",
last_id: "12",
});
console.log(activity);
.getFullActivity
// Dates must be in format YYYY-MM-DD
const activity = await client.getFullActivity({
from: "2023-04-01",
to: "2023-05-10",
});
console.log(activity);
.getBehaviour
Gets behaviour for a given date range.
// Dates must be in format YYYY-MM-DD
const behaviour = await client.getBehaviour({
from: "2023-04-01",
to: "2023-05-10",
});
console.log(behaviour);
/**
{
"success": 1,
"data": {
"timeline": [
{
"positive": 426,
...
},
],
...
"meta": {
"start_date": "2023-04-01T00:00:00+00:00",
...
}
}
*/
.getHomeworks
Gets homeworks for a given date range.
// Dates must be in format YYYY-MM-DD
const homeworks = await client.getHomeworks({
from: "2023-04-01",
to: "2023-05-10",
displayDate: 'issue_date' // Can be 'due_date' or 'issue_date'
});
console.log(homeworks);
/**
{
success: 1,
data: [
{
lesson: '7A/Pe1',
...
},
],
meta: {
start_date: '2023-04-01T00:00:00+00:00',
...
}
}
.getLessons
Gets lessons for a specific date.
// Dates must be in format YYYY-MM-DD
const lessons = await client.getLessons({
date: "2023-04-01",
});
console.log(lessons);
/**
{
"success": 1,
"data": [
{
"teacher_name": "Mr J Doe",
...
}
...
],
"meta": {
"dates": [
"2023-05-04"
],
...
}
}
*/
.getBadges
Gets all earned badges.
const badges = await client.getBadges();
console.log(badges);
/**
{
success: 1,
data: [
{
id: 123,
name: 'Big Badge',
...
},
...
],
meta: []
}
*/
.getAnnouncements
Gets all announcements.
const announcements = await client.getAnnouncements();
console.log(announcements);
/**
{
success: 1,
data: [
{
id: 321453,
title: "A big announcement",
description: "<p>School will be closing early today!</p>",
...
}
],
meta: []
}
*/
.getDetentions
?> This method does not include meta
in the response, since I do not have
access to this endpoint to test it. If you have access to this endpoint, please
open a PR to add the meta
response. Thanks!
Gets all detentions.
const detentions = await client.getDetentions();
console.log(detentions);
/**
{
"success": 1,
"data": [
{
"id": 12345678,
"attended": "no",
"date": "2024-03-28T00:00:00+00:00",
...
}
],
"meta": {
"detention_alias_plural": "detentions"
}
}
*/
.getAttendance
?> This method does not include meta
in the response, since I do not have
access to this endpoint to test it. If you have access to this endpoint, please
open a PR to add the meta
response. Thanks!
Gets attendance.
const attendance = await client.getAttendance();
console.log(attendance);
.getPupils
Gets a list of all pupils the parent has access to.
const pupils = await client.getPupils();
console.log(pupils);
/**
[
{
id: 123,
name: 'John Doe',
...
},
...
]
*/
.selectPupil
Selects a pupil to make subsequent requests for.
await client.selectPupil(123);
.changePassword
Changes the parent's password.
await client.changePassword("oldPassword", "newPassword");
FAQs
A Typescript wrapper for getting information from the ClassCharts API
The npm package classcharts-api receives a total of 5 weekly downloads. As such, classcharts-api popularity was classified as not popular.
We found that classcharts-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.