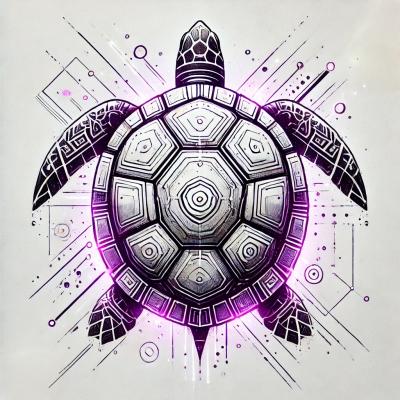
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
collection-deep-merge
Advanced tools
Deep merge array of objects by passed key. Objects with same key vals will merge together
Genereal purpose arrays of objects by key merger.
Ready for browsers (const
, let
compatible) and node.js v4+.
IE11+, FF36+, Safari5.1+, Android
Works well as redux store helper.
Extensible via custom merger and comparator (Dependency Injection factory). Look more below.
It does not mutate arguments, it returns a new collection (Immutability).
Open test.js with examples
const mergeById = mergeCollectionsBy('id')
//
// Deep merge object-items
const list1 = [ { a: 1, b: { c: 1 } }, { a: 2, b: { d: 2 } } ];
const list2 = [ { a: 1, b: { c: 3 } }, { a: 2, b: { c: 4 } } ];
const merged = [ { a: 1, b: { c: 3 } }, { a: 2, b: { c: 4, d: 2 } } ];
mergeById(list, [ item ]) ==== merged // deep equal
//
// Merge item with a collection
const list = [ { a: 1, b: 2 }, { a: 2, d: 4 } ];
const item = { a: 1, c: 3 };
const merged = [{ a: 1, b: 2, c: 3 }, { a: 2, d: 4 }]
mergeById(list, [ item ]) ==== merged // deep equal
item[key]
s comparators and item
s mergersconst mergeCollectionsBy = require('collection-deep-merge');
const is = (a, b) => a !== undefined && a === b;
// You can merge in a shallow manner
// or implement very custom strategy
const merge = (a, b) => {/* custom merger */};
const mergeDeepById = mergeCollectionsBy('id', { is, merge });
import mergeCollectionsBy, { mergeShallow } = 'collection-deep-merge';
const mergeShallowById = mergeCollectionsBy('id', { merge: mergeShallow });
You can implement custom merger based on
const { mergeDeep } = require('collection-deep-merge')
with an especial behaviour on nested collections.
You should support followed api:
(Object a, Object b)
argsObject c
collection-deep-merge like its fullJoin
with deep item-objects merge
New native Map has all you need:
You can implement tiny union function (w/o deep items merging):
function union(map1, map2) {
return new Map([...map1, ...map2]);
}
or you can use the collection-deep-merge if you want to merge items deeply
O(n*m) + O(?)
complexity (n*m
not so good; ?
stands for deep merge).
import mergeArrays from 'collection-deep-merge';
const mergeById = mergeArrays('id', { eq: (item1, item2) => {} });
const mergeMapsById = (map1, map2) => {
mergeById([...map1], [...map2])
};
or ... // todo: create map-deep-union
library special for Maps with O(n)+O(?)
complexity.
FAQs
Deep merge array of objects by passed key. Objects with same key vals will merge together
We found that collection-deep-merge demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.