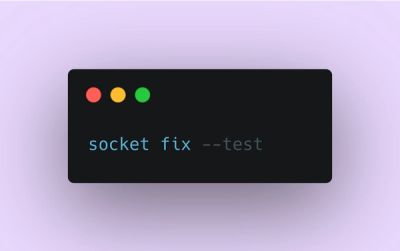
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
A JS library for Color Conversions, Manipulations, Harmony Generations, Accessibility Analysis and Parsing.
Β Β Β Β Β Β Easily manipulate colors with functions to lighten, darken, saturate, desaturate, invert, and blend colors.
Β Β Β Β Β Β Generate harmonious colors using monochromatic, complementary, triadic, tetradic, and more color schemes.
Β Β Β Β Β Β Validate color formats to ensure correct color values before applying transformations.
Β Β Β Β Β Β Convert colors between all popular color formats such as RGB, HEX, HSL, LAB, LCH, and more.
Β Β Β Β Β Β Calculate contrast ratios, luminance, and other color metrics for accessibility.
Β Β Β Β Β Β Parse individual components of colors, decompose and recompose colors.
Β Β Β Β Β Β Optimized for performance with a small footprint.
Β Β Β Β Β Β Designed to be lean and efficient without any external dependencies.
Β Β Β Β Β Β ~10 KB gzipped.
To install the Colore library, use the follow command:
npm install colore-js
Alternatively, if you use Yarn:
yarn add colore-js
import { hexToRgb, lightenColor, saturateColor, setCssVariableValue } from 'colore-js';
// Lightening a color
const lightenedRgb = lightenColor('rgb(255, 0, 0)', 20);
console.log(lightenedRgb); // Output: 'rgb(255, 51, 51)'
// Saturating a color
const saturatedRgb = saturateColor('rgb(255, 0, 0)', 50);
console.log(saturatedRgb); // Output: 'rgb(255, 51, 51)'
// Converting HEX to RGB color format
const rgbString = hexToRgb('#ff5733');
console.log(rgbString); // Output: 'rgb(255, 87, 51)'
// Setting a new CSS Variable Value
const element = document.querySelector('.my-element');
setCssVariableValue(element, '--my-variable', 'blue');
import { getContrastRatio } from 'colore-js';
const result = getContrastRatio('#ffffff', '#000000');
console.log(result); // Output: { ratio: 21, ratioString: "21.00:1", isAccessible: true, level: 'AAA' }
import { getLuminance } from 'colore-js';
const luminance = getLuminance('#ffffff');
console.log(luminance); // Output: 1
import { cmykToRgb } from 'colore-js';
const rgbString = cmykToRgb(0, 100, 100, 0);
console.log(rgbString); // Output: "rgb(255, 0, 0)"
import { hexAlphaToHsla } from 'colore-js';
const hslaColor = hexAlphaToHsla('#ff5733cc');
console.log(hslaColor); // Output: "hsla(14, 100%, 60%, 0.8)"
import { hexAlphaToHsva } from 'colore-js';
const hsvaString = hexAlphaToHsva('#ff5733cc');
console.log(hsvaString); // Output: "hsva(11, 0.8, 1, 0.8)"
import { hexAlphaToRgba } from 'colore-js';
const rgbaString = hexAlphaToRgba('#FF5733CC');
console.log(rgbaString); // Output: "rgba(255, 87, 51, 0.8)"
import { hexToHexAlpha } from 'colore-js';
const hexWithAlpha = hexToHexAlpha('#ff0000', 0.5);
console.log(hexWithAlpha); // Output: '#ff000080'
See all Conversions.
import { getCssVariableValue } from 'colore-js';
const element = document.querySelector('.my-element');
const variableValue = getCssVariableValue(element, '--my-variable');
console.log(variableValue); // Output: 'your-css-variable-value'
import { setCssVariableValue } from 'colore-js';
const element = document.querySelector('.my-element');
setCssVariableValue(element, '--my-variable', 'blue');
import { generateInterpolatedColors } from 'colore-js';
const color1 = '#ff0000';
const color2 = '#00ff00';
const steps = 5;
const interpolatedColorsStrings = generateInterpolatedColors(color1, color2, steps);
console.log(interpolatedColorsStrings);
import { generateRandomColor, ColorFormats } from 'colore-js';
const randomHexColor = generateRandomColor(ColorFormats.HEX);
console.log(randomHexColor); // Output: "#a1b2c3" (example)
import { analogousColors } from 'colore-js';
const analogous = analogousColors('#ff0000');
console.log(analogous); // Output: ['#ff8000', '#ff0080']
import { complementaryColor } from 'colore-js';
const complementary = complementaryColor('#ff0000');
console.log(complementary); // Output: '#00ffff'
import { monochromaticColors } from 'colore-js';
const monochromatic = monochromaticColors('#ff0000');
console.log(monochromatic); // Output: ['#4c0000', '#b20000', '#ff0000', '#ff4c4c', '#ff9999']
import { tetradicColors } from 'colore-js';
const tetradic = tetradicColors('#ff0000');
console.log(tetradic); // Output: ['#00ff00', '#0000ff', '#ff00ff']
import { triadicColors } from 'colore-js';
const triadic = triadicColors('#ff0000');
console.log(triadic); // Output: ['#00ff00', '#0000ff']
import { blendColors, BlendingModes } from 'colore-js';
const blended = blendColors('#ff0000', '#0000ff', BlendingModes.MULTIPLY);
console.log(blended); // Output: '#000000'
import { darkenColor } from 'colore-js';
const darkened = darkenColor('#ff0000', 20);
console.log(darkened); // Output: '#cc0000'
import { desaturateColor } from 'colore-js';
const desaturated = desaturateColor('#ff0000', 50);
console.log(desaturated); // Output: '#804040'
import { invertColor } from 'colore-js';
const invertedColor = invertColor("#ff5733");
console.log(invertedColor); // Output: "#00a8cc"
import { lightenColor } from 'colore-js';
const lightened = lightenColor('#ff0000', 20);
console.log(lightened); // Output: '#ff6666'
See all Manipulations.
import { decomposeColor } from 'colore-js';
const decomposedHex = decomposeColor('#ff0000');
console.log(decomposedHex); // Output: { r: 255, g: 0, b: 0 }
import { detectColorFormat } from 'colore-js';
const formatHex = detectColorFormat('#ff0000');
console.log(formatHex); // Output: 'HEX'
import { parseColorToRgba } from 'colore-js';
const rgbaHex = parseColorToRgba('#ff0000');
console.log(rgbaHex); // Output: { r: 255, g: 0, b: 0 }
import { parseHex } from 'colore-js';
const rgb = parseHex('#ff0000');
console.log(rgb); // Output: { r: 255, g: 0, b: 0 }
import { parseHexAlpha } from 'colore-js';
const rgba = parseHexAlpha('#ff000080');
console.log(rgba); // Output: { r: 255, g: 0, b: 0, a: 0.502 }
See all Parsers.
import { isValidHex } from 'colore-js';
console.log(isValidHex('#ff0000')); // Output: true
import { isValidHexAlpha } from 'colore-js';
console.log(isValidHexAlpha('#ff0000ff')); // Output: true
import { isValidHsl } from 'colore-js';
console.log(isValidHsl('hsl(120, 100%, 50%)')); // Output: true
import { isValidHsla } from 'colore-js';
console.log(isValidHsla('hsla(120, 100%, 50%, 0.5)')); // Output: true
import { isValidLab } from 'colore-js';
console.log(isValidLab('lab(50% 0% 0%)')); // Output: true
See all Validations.
See Documentation for complete API reference.
We welcome contributions from the community to make Colore better. If you find any issues or have suggestions for improvements, feel free to contribute or open an issue on our GitHub Repository.
This project is licensed under the MIT License - see the LICENSE file for details.
If you find any issues or have suggestions for improvements, feel free to contribute or open an issue on our GitHub Repository. We welcome contributions from the community to make Colore better.
FAQs
A JS library for Color Conversions, Manipulations, Harmony Generations, Accessibility Analysis and Parsing.
The npm package colore-js receives a total of 909 weekly downloads. As such, colore-js popularity was classified as not popular.
We found that colore-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
Weβre excited to announce a powerful new capability in Socket: historical data and enhanced analytics.