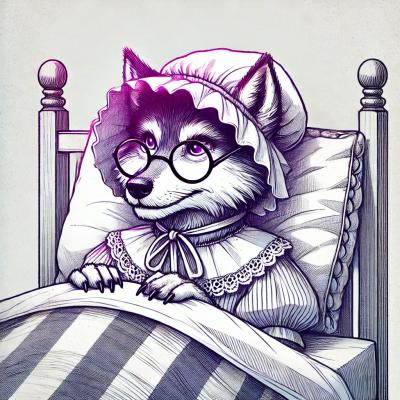
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Easily create descriptive comparators.
compareBy
Make sure to check out the documentation.
npm i comparing
Import all comparators, factories and types from 'comparing'
.
All comparators are fully tested, so you can find more examples in the unit tests. A lot of comparators also have examples in their documentation.
import { naturalOrder } from 'comparing';
const actual = [4, 1, 3, 5].sort(naturalOrder);
expect(actual).toEqual([1, 3, 4, 5]);
const comparator: Comparator<string | null> = composeComparators([nullishFirst, localeCompare]);
const actual = ['A', 'a', 'b', null, 'B'].sort(comparator);
expect(actual).toEqual([null, 'a', 'A', 'b', 'B']);
const reversedComparator = reverseComparator(comparator);
const actualReversed = ['A', 'a', 'b', null, 'B'].sort(reversedComparator);
expect(actual).toEqual(['B', 'b', 'A', 'a', null]);
The following example shows how to sort an array of objects, which have an optional order
property that should take precedence when sorting.
If equal or not present, then the objects should be sorted by their name
property.
const nameComparator: Comparator<{ name: string }> = compareBy((x) => x.name, ignoreCase);
const orderComparator = compareBy((x) => x.order, composeComparators([nullishLast, naturalOrder]));
const myObjectComparator = composeComparators([orderComparator, nameComparator]);
const actual = [
{ name: 'B', order: 1 },
{ name: 'F' },
{ name: 'C', order: 3 },
{ name: 'A', order: 1 },
{ name: 'D' },
{ name: 'E', order: 2 },
].sort(myObjectComparator);
expect(actual).toEqual([
{ name: 'A', order: 1 },
{ name: 'B', order: 1 },
{ name: 'E', order: 2 },
{ name: 'C', order: 3 },
{ name: 'D' },
{ name: 'F' },
]);
The library has no dependencies, thus only creates and works with the JavaScript objects and functions you already know.
Compare with dot path
import { compareBy } from 'comparing';
import get from 'lodash/fp/get';
const abcComparator = compareBy(get('a.b.c'));
const data = [
{ a: { b: { c: 1 } } },
{ a: { b: { c: 0 } } },
{ a: { b: { c: 2 } } },
].sort(abcComparator);
expect(data).toEqual([
{ a: { b: { c: 0 } } },
{ a: { b: { c: 1 } } },
{ a: { b: { c: 2 } } },
]);
Sort dependencies
import {
compareBy,
comparatorForOrder,
composeComparators,
Comparator,
} from 'comparing';
import toposort from 'toposort';
const graph = [
// must first put on the shirt before the jacket, and so on ...
['put on your shirt', 'put on your jacket'],
['put on your shorts', 'put on your jacket'],
['put on your shorts', 'put on your shoes'],
];
const taskComparator = comparatorForOrder(toposort(graph));
const comparator: Comparator<{ day: number; task: string }> = composeComparators([
compareBy((x) => x.day), // naturalOrder implicitly
compareBy((x) => x.task, taskComparator),
]);
const todoList = [
{ day: 0, task: 'put on your jacket' },
{ day: 0, task: 'put on your shirt' },
{ day: 1, task: 'put on your shoes' },
{ day: 2, task: 'put on your shirt' },
{ day: 1, task: 'put on your jacket' },
{ day: 1, task: 'put on your shorts' },
].sort(comparator);
expect(todoList).toEqual([
{ day: 0, task: 'put on your shirt' },
{ day: 0, task: 'put on your jacket' },
{ day: 1, task: 'put on your shorts' },
{ day: 1, task: 'put on your jacket' },
{ day: 1, task: 'put on your shoes' },
{ day: 2, task: 'put on your shirt' },
]);
FAQs
Easily create descriptive comparators
We found that comparing demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.