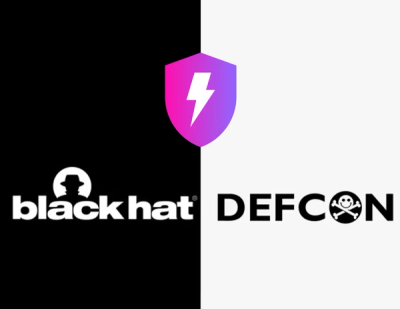
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
concat-with-sourcemaps
Advanced tools
Concatenate file contents with a custom separator and generate a source map
The concat-with-sourcemaps npm package allows you to concatenate multiple files and generate source maps for the concatenated output. This is particularly useful in build processes where you need to combine multiple JavaScript or CSS files into a single file while maintaining the ability to debug the original source files.
Concatenate files
This feature allows you to concatenate multiple files into a single file. The example code demonstrates how to concatenate two JavaScript files, 'file1.js' and 'file2.js', into a single file 'all.js'.
const Concat = require('concat-with-sourcemaps');
const concat = new Concat(true, 'all.js', '\n');
concat.add('file1.js', 'console.log("file1");\n');
concat.add('file2.js', 'console.log("file2");\n');
console.log(concat.content.toString());
console.log(concat.sourceMap);
Generate source maps
This feature allows you to generate source maps for the concatenated output. The example code demonstrates how to add source maps for 'file1.js' and 'file2.js' with a specified source root.
const Concat = require('concat-with-sourcemaps');
const concat = new Concat(true, 'all.js', '\n');
concat.add('file1.js', 'console.log("file1");\n', { sourceRoot: 'src' });
concat.add('file2.js', 'console.log("file2");\n', { sourceRoot: 'src' });
console.log(concat.content.toString());
console.log(concat.sourceMap);
gulp-concat is a Gulp plugin that concatenates files. It is similar to concat-with-sourcemaps but is designed to work within the Gulp build system. It also supports source maps but requires additional plugins like gulp-sourcemaps to generate them.
broccoli-concat is a Broccoli plugin for concatenating files. It is similar to concat-with-sourcemaps but is designed to work within the Broccoli build system. It supports source maps and offers more configuration options for handling input and output files.
webpack-concat-plugin is a Webpack plugin that concatenates files and generates source maps. It is similar to concat-with-sourcemaps but is designed to work within the Webpack build system. It offers additional features like minification and custom file ordering.
NPM module for concatenating files and generating source maps.
var concat = new Concat(true, 'all.js', '\n');
concat.add(null, "// (c) John Doe");
concat.add('file1.js', file1Content);
concat.add('file2.js', file2Content, file2SourceMap);
var concatenatedContent = concat.content;
var sourceMapForContent = concat.sourceMap;
Initialize a new concat object.
Parameters:
Add a file to the output file.
Parameters:
The resulting concatenated file content (Buffer).
The resulting source map of the concatenated files (string).
FAQs
Concatenate file contents with a custom separator and generate a source map
The npm package concat-with-sourcemaps receives a total of 1,374,758 weekly downloads. As such, concat-with-sourcemaps popularity was classified as popular.
We found that concat-with-sourcemaps demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.