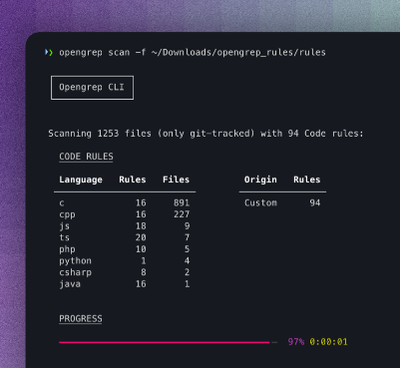
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
concurrent-task
Advanced tools
An observable to run async tasks in parallel with a concurrency limit
A brisky-struct
instance to run async tasks in parallel with a concurrency limit
npm install concurrent-task --save
Below is an example of 5 tasks with 2 steps to execute for each. Each step has a run
method which returns an aborting function. If step is not resolved or rejected in time, it will be aborted and an error will be emitted.
const concurrent = require('concurrent-task')
const steps = [
{
timeout: 1000,
tryCount: 2,
run (task, resolve, reject) {
return clearTimeout.bind(null, setTimeout(() => {
return task.success ? resolve() : reject(new Error('some error'))
}, task.seconds * 1000))
}
},
{
timeout: 500,
tryCount: 3,
run (task, resolve, reject) {
return clearTimeout.bind(null, setTimeout(() => {
return task.success ? resolve({ [task.id]: 'result' }) : reject(new Error('some error'))
}, task.seconds * 1000))
}
}
]
// create a task runner with steps
const runner = concurrent(steps)
// this method can be called several times
// so to add more tasks on the go
runner.addTask([
{ id: 'task1', seconds: 0.3, success: true },
{ id: 'task2', seconds: 0.7, success: true },
{ id: 'task3', seconds: 0.2, success: false },
{ id: 'task4', seconds: 1.2, success: false },
{ id: 'task5', seconds: 0.3, success: true }
])
runner
.on('error', (task, error) => {
// one of tasks had an error on one of steps
// task is something like { id: 'task3', seconds: 0.2, success: false }
})
.on('task-done', task => {
// one of tasks completed all steps with no error
// task is something like { id: 'task3', seconds: 0.2, success: false }
})
.on('complete', () => {
// all the tasks completed all steps
// if any of them had errors
// tryCounts are exhausted
// log runner status
console.log(runner.status())
// logs something like: { waiting: 0, running: 0, error: 2, done: 3 }
// log task results
console.log(runner.results())
// logs something like: { task1: 'result', task5: 'result' }
// keep memory clean
runner.set(null)
})
// run with concurrency limited to 2
.run(2)
FAQs
An observable to run async tasks in parallel with a concurrency limit
We found that concurrent-task demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.