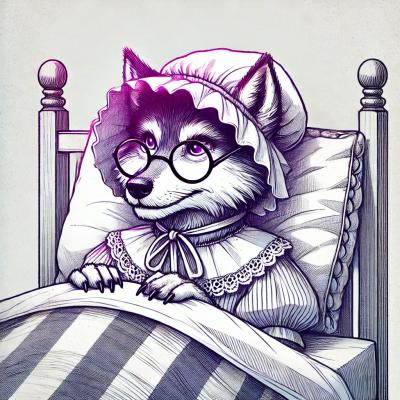
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Smart configuration control
npm i confige
This package by default read the config folder in your working directory:
const config = require("confige");
console.log(config.app.name);
console.log(config.database.mysql.host);
console.log(config.language.supported[0]);
If folder config does not exist, file config.json will be checked instead.
{
"app": {
"name": "MY APP"
}
}
const config = require("confige");
console.log(config.app.name);
You can override the config file path with an environment variable:
// read a folder
process.env.CONFIG_FILE=src/config
// read a file
process.env.CONFIG_FILE=src/config.json
// with absolute path
process.env.CONFIG_FILE=/path/to/src/config.json
If you specified a single file, the config object will be the file itself rather than a nested object with the filename as its key(like folder reading does).
src/config.json
{
"app": {
"name": "myapp"
}
}
process.env.CONFIG_FILE = src / config.json;
const config = require("confige");
console.log(config.app.name);
It won't be loaded again once done. To reload configs, you need to call:
// delete cached config
config.__.desolve();
// reload
config = require("confige");
By default, This package read a .env file in your working directory and get environment variables from this file.
NODE_ENV=production
CONFIG_FILE=src/config.json
You may override this behaviour by passing the following environment variable:
process.env.ENV_FILE=src/.env
const config = require('confige');
You may optionally set ENV_INJECT
to inject env file variables into process.env
.
Existing environment variables won't be overrided.
process.env.ENV_INJECT=true
NODE_ENV=development npm start
# NODE_ENV won't be set because it already exists
NODE_ENV=production
CONFIG_FILE=src/config.json
A bunch of keywords can be used in config files
{
"name": {
"$env": "APP_NAME"
},
"database": {
"port": {
"$env": ["DB_PORT", 3306]
},
"host": {
"$env": ["DB_HOST", "localhost"]
},
"username": {
"$env": "DB_USER"
},
"password": {
"$env": ["DB_PASS", { "$env": "DB_USER" }]
}
}
}
The $env
object reads environment varaibles with an optional fallback value. With the following env set:
APP_NAME=myapp
DB_HOST=10.10.10.100
DB_USER=admin
The loaded config will be
{
"name": "myapp",
"database": {
"port": 3306,
"host": "10.10.10.100",
"username": "admin",
"password": "admin"
}
}
Here is a list of available keywords:
$env
Get value from environment variables(first try env file, then process.env) with an optional fallback value
string
|[string, any]
any
$path
Resolve the path to absolute from current working directory
string
string
$file
Resolve the path and read the file with an optional encoding
string
|[string, string]
string
|Buffer
$json
Parse the given string into object
string
any
$number
Parse the given string into a number
string
number
$concat
Concat strings or arrays
string[]
|[][]
string
|any[]
$max
Return the max number in the array
number[]
number
$min
Return the min number in the array
number[]
number
$sum
Sum the numbers in the array
number[]
number
$avg
Return the average value of the array
number[]
number
$first
Return the first element in the array
any[]
any
$last
Return the last element in the array
any[]
any
$at
Return element in the array at the given index
[any[], number]
any
$asce
Sort the numbers in ascending order
number[]
number[]
$asce
Sort the numbers in descending order
number[]
number[]
$rand
Return an element at random index
any[]
any
$rands
Return the given amount of elements at random indices
[any[], number]
any[]
$reverse
Reverse an array
any[]
any[]
$slice
Slice an array from the given index to an optional end index
[any[], number]
|[any[], number, number]
any[]
$count
Return the length of an array
any[]
number
$merge
Return the merge of two objects
[any, any]
any
$keys
Return the keys of an object
any
string[]
$vals
Return the values of an object
any
any[]
$zip
Merge a series of key-value pairs into an object
[any, any][]
any
$zap
Split an object into key-value pairs
any
[any, any][]
$cond
Return the second or third element based on the boolean value of the first element
[boolean, any, any]
any
$and
Return true only if both two elements' boolean values are true
[boolean, boolean]
boolean
$or
Return true if any of the two elements' boolean value is true
[boolean, boolean]
boolean
$not
Return true only if the given value is false
boolean
boolean
$true
Return true if the given value is true or 'true'(case insensitive) or 1 or '1'
boolean|string
boolean
$null
Return true if the given value is null or undefined
any
boolean
$undefined
Return true only if the given value is undefined
any
boolean
$type
Return true only if the given value is of the given type
[any, string]
boolean
$test
Return boolean test result(!!) of the given value
[any, string]
boolean
Operators
$abs, $add(+), $sub(-), $mul(*), $div(/), $mod(%), $ceil, $floor, $round, $trunc, $sign
Comparers
$gt(>), $gte(>=), $lt(<), $lte(<=), $eq(===), $eqv(==), $ne(!==), $nev(!=), $in, $ni
String Morph
$upper, $lower, $snake, $pascal, $camel, $dash, $plural, $singular
A utils is attached to each config instance. You can acces it by the getter __
:
// make a deep cloned copy
const copy = config.__.clone(obj);
// merge target object's copy into source object's copy deeply
const merged = config.__.merge(source, target);
// load environment variables, optionally inject them into process.env
const envs = config.__.env("./.env", true);
// parse config by json string
let conf = config.__.resolve(
JSON.parse(fs.readFileSync("./config.json", "utf8"))
);
// load config by path(absolute or relative to working directory)
conf = config.__.load("./config.json");
// delete config referenced cache(the next time you reference this module, config file will be reloaded)
config.__.desolve();
You can also use utils without the config instance:
const utils = require("confige/utils");
// NOTE: utils.desolve will be undefined
To integrate into webpack, there is a built-in plugin:
const ConfigurePlugin = require("confige/utils/webpack");
webpack.config.js
plugins: [
new ConfigurePlugin({
filename: path.resolve(__dirname, "path/to/config.json")
})
];
And import that config in your modules:
index.js
import * as config from "./path/to/config.json";
You may add a resolve alias to shorten the import:
webpack.config.js
resolve: {
alias: {
config: path.resolve(__dirname, 'path/to/config.json')
}
},
index.js
import * as config from "config";
NOTE
By default the json config is loaded as string. It's also compatible with file-loader. If conflicts occur, you may need to bypass the specific loader(s) with include/exclude
filters. e.g.
{
test: /\.json$/,
exclude: /\/config\.json$/,
use: 'some-loader'
}
npm test
See License
FAQs
Smart configuration control
We found that confige demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.