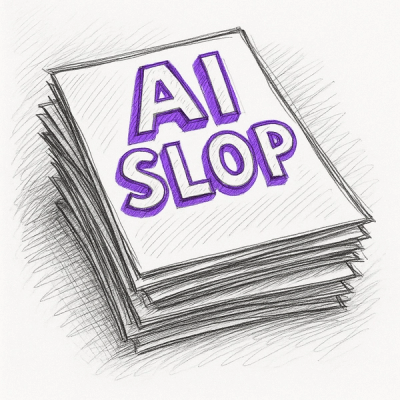
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
connect-mikro-orm
Advanced tools
Configure MikroORM with back end of your choice:
yarn add connect-mikro-orm @mikro-orm/core @mikro-orm/knex express-session
yarn add -D @types/express-session
Session
entity:// src/domain/Session/Session.ts
import type { ISession } from 'connect-mikro-orm';
import { Entity, Index, PrimaryKey, Property } from '@mikro-orm/core';
@Entity()
export class Session implements ISession {
@PrimaryKey()
id: string;
@Property({ columnType: 'text' })
json: string;
@Index()
@Property({ columnType: 'bigint' })
expiredAt: number;
}
Pass repository to MikroOrmStore
:
// src/app/Api/Api.ts
import { MikroOrmStore } from 'connect-mikro-orm';
import * as Express from 'express';
import * as ExpressSession from 'express-session';
import { Session } from '../../domain/Session/Session';
export class Api {
public sessionRepository = entityManager.getRepository(Session);
public express = Express().use(
ExpressSession({
resave: false,
saveUninitialized: false,
store: new MikroOrmStore({
ttl: 86400,
}).connect(this.sessionRepository),
secret: 'keyboard cat',
})
);
}
Constructor receives an object. Following properties may be included:
ttl
Session time to live (expiration) in seconds. Defaults to session.maxAge (if set), or one day. This may also be set to a function of the form (store, sess, sessionID) => number
.
onError
Error handler for database exception. It is a function of the form (store: MikroOrmStore, error: Error) => void
. If not set, any database error will cause the MikroOrmStore to be marked as "disconnected", and stop reading/writing to the database, therefore not loading sessions and causing all requests to be considered unauthenticated.
MIT
FAQs
A MikroORM-based session store
The npm package connect-mikro-orm receives a total of 0 weekly downloads. As such, connect-mikro-orm popularity was classified as not popular.
We found that connect-mikro-orm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.