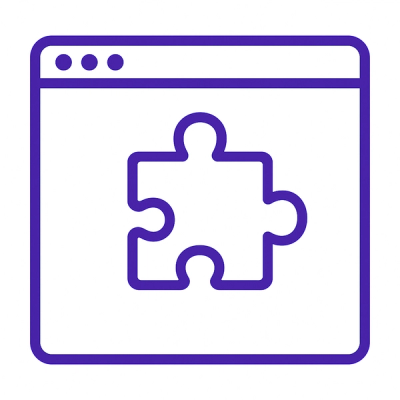
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
console-grid
Advanced tools
npm i console-grid
const CG = require("console-grid");
CG({
"columns": ["", "Name", "Value"],
"rows": [
[1, "Tom", "Value 1"],
[2, "Jerry", "Value 2"]
]
});
βββββ¬ββββββββ¬ββββββββββ
β β Name β Value β
βββββΌββββββββΌββββββββββ€
β 1 β Tom β Value 1 β
β 2 β Jerry β Value 2 β
βββββ΄ββββββββ΄ββββββββββ
const CG = require("console-grid");
CG({
"options": {
"headerVisible": false
},
"columns": ["", "Name", "Value"],
"rows": [
[1, "Tom", "Value 1"],
[2, "Jerry", "Value 2"]
]
});
βββββ¬ββββββββ¬ββββββββββ
β 1 β Tom β Value 1 β
β 2 β Jerry β Value 2 β
βββββ΄ββββββββ΄ββββββββββ
const CG = require("console-grid");
CG({
"columns": ["", {
"name": "Name",
"minWidth": 15
}, {
"name": "Value",
"maxWidth": 20
}, {
"name": "Multiple Line Header",
"maxWidth": 15
}],
"rows": [
[1, "Hello", "Long Text Value", "Long Text Value"],
[2, "Hello There", "Long Text Value Long Text Value", "Long Text Value Long Text Value"]
]
});
βββββ¬ββββββββββββββββββ¬βββββββββββββββββββββββ¬ββββββββββββββββββ
β β β β Multiple Line β
β β Name β Value β Header β
βββββΌββββββββββββββββββΌβββββββββββββββββββββββΌββββββββββββββββββ€
β 1 β Hello β Long Text Value β Long Text Value β
β 2 β Hello There β Long Text Value L... β Long Text Va... β
βββββ΄ββββββββββββββββββ΄βββββββββββββββββββββββ΄ββββββββββββββββββ
const CG = require("console-grid");
CG({
"options": {
"padding": 2
},
"columns": [{
"id": "default",
"name": "Default"
}, {
"id": "left",
"name": "Left",
"align": "left"
}, {
"id": "center",
"name": "Center",
"align": "center"
}, {
"id": "right",
"name": "Right",
"align": "right"
}, {
"id": "right",
"name": "Multiple Line Right",
"maxWidth": 12,
"align": "right"
}],
"rows": [{
"default": "Cell",
"left": "Markdown",
"center": "Start",
"right": "123.0"
}, {
"default": "Content",
"left": "Grid",
"center": "Complete",
"right": "8.1"
}]
});
βββββββββββββ¬βββββββββββββ¬βββββββββββββ¬ββββββββββ¬βββββββββββββββββ
β β β β β Multiple β
β Default β Left β Center β Right β Line Right β
βββββββββββββΌβββββββββββββΌβββββββββββββΌββββββββββΌβββββββββββββββββ€
β Cell β Markdown β Start β 123.0 β 123.0 β
β Content β Grid β Complete β 8.1 β 8.1 β
βββββββββββββ΄βββββββββββββ΄βββββββββββββ΄ββββββββββ΄βββββββββββββββββ
const CG = require("console-grid");
CG({
"columns": [{
"id": "name",
"name": "Name",
"type": "string",
"maxWidth": 30
}, {
"id": "value",
"name": "Value",
"type": "string",
"maxWidth": 7
}, {
"id": "null",
"name": "Null"
}, {
"id": "number",
"type": "number",
"name": "Number",
"maxWidth": 12
}],
"rows": [{
"name": "Row 1",
"value": "1",
"number": 1
}, {
"name": "Row Name",
"value": "2",
"number": 2
}, {
"name": "Row Long Name Long Name Long Name",
"value": "3",
"number": 3
}, {
"name": "Group",
"value": "4",
"number": 4,
"subs": [{
"name": "Sub Group 1",
"value": "5",
"number": 5,
"subs": [{
"name": "Sub Group 1 Sub Row 1",
"value": "6",
"number": 6
}, {
"name": "Sub Group 1 Sub Row 2",
"value": "7",
"number": 7
}]
}, {
"name": "Sub Row 1",
"value": "8",
"number": 8
}, {
"name": "Sub Row 2",
"value": "9",
"number": 9
}]
}]
});
ββββββββββββββββββββββββββββββββββ¬ββββββββ¬βββββββ¬βββββββββ
β Name β Value β Null β Number β
ββββββββββββββββββββββββββββββββββΌββββββββΌβββββββΌβββββββββ€
β Row 1 β 1 β - β 1.00 β
β Row Name β 2 β - β 2.00 β
β Row Long Name Long Name Lon... β 3 β - β 3.00 β
β Group β 4 β - β 4.00 β
β β Sub Group 1 β 5 β - β 5.00 β
β β β Sub Group 1 Sub Row 1 β 6 β - β 6.00 β
β β β Sub Group 1 Sub Row 2 β 7 β - β 7.00 β
β β Sub Row 1 β 8 β - β 8.00 β
β β Sub Row 2 β 9 β - β 9.00 β
ββββββββββββββββββββββββββββββββββ΄ββββββββ΄βββββββ΄βββββββββ
const CG = require("console-grid");
CG({
"columns": [{
"id": "name",
"name": "Name"
}, {
"id": "value",
"name": "Value"
}],
"rows": [{
"name": "Total",
"value": 80
}, {
"innerBorder": true
}, {
"name": "Item 1",
"value": 30
}, {
"name": "Item 2",
"value": 50,
"subs": [{
"name": "Sub 21"
}, {
"name": ""
}, {
"name": "Sub 22"
}]
}]
});
ββββββββββββ¬ββββββββ
β Name β Value β
ββββββββββββΌββββββββ€
β Total β 80 β
ββββββββββββΌββββββββ€
β Item 1 β 30 β
β Item 2 β 50 β
β β Sub 21 β - β
β β β - β
β β Sub 22 β - β
ββββββββββββ΄ββββββββ
const CG = require("console-grid");
CG({
"options": {
"sortField": "value",
"sortAsc": false
},
"columns": [{
"id": "name",
"name": "Name"
}, {
"id": "value",
"name": "Value",
"type": "number"
}],
"rows": [{
"name": "Item 1",
"value": 80
}, {
"name": "Item 2",
"value": 30
}, {
"name": "Item 3",
"value": 50
}]
});
ββββββββββ¬βββββββββ
β Name β Value* β
ββββββββββΌβββββββββ€
β Item 1 β 80 β
β Item 3 β 50 β
β Item 2 β 30 β
ββββββββββ΄βββββββββ
const CG = require("console-grid");
const EC = require("eight-colors");
const data = {
columns: ['Name', EC.cyan('Color Text'), EC.bg.cyan('Color Background')],
rows: [
['Red', EC.red('red text'), EC.bg.red('red bg')],
['Green', EC.green('green text'), EC.bg.green('green text')]
]
};
CG(data);
// silent output and remove color
data.options = {
silent: true
};
const lines = CG(data);
const withoutColor = EC.remove(lines.join(os.EOL));
console.log(withoutColor);
βββββββββ¬βββββββββββββ¬βββββββββββββββββββ
β Name β Color Text β Color Background β
βββββββββΌβββββββββββββΌβββββββββββββββββββ€
β Red β red text β red bg β
β Green β green text β green text β
βββββββββ΄βββββββββββββ΄βββββββββββββββββββ
const CG = require("console-grid");
const Papa = require("papaparse");
const csvString = `Column 1,Column 2,Column 3,Column 4
1-1,1-2,1-3,1-4
2-1,2-2,2-3,2-4
3-1,3-2,3-3,3-4
4,5,6,7`;
const json = Papa.parse(csvString);
const data = {
columns: json.data.shift(),
rows: json.data
};
CG(data);
ββββββββββββ¬βββββββββββ¬βββββββββββ¬βββββββββββ
β Column 1 β Column 2 β Column 3 β Column 4 β
ββββββββββββΌβββββββββββΌβββββββββββΌβββββββββββ€
β 1-1 β 1-2 β 1-3 β 1-4 β
β 2-1 β 2-2 β 2-3 β 2-4 β
β 3-1 β 3-2 β 3-3 β 3-4 β
β 4 β 5 β 6 β 7 β
ββββββββββββ΄βββββββββββ΄βββββββββββ΄βββββββββββ
const CG = require("console-grid");
CG({
"columns": ["Special", "Character"],
"rows": [
["Chinese,δΈζ", "12γζ οΌηΉγγ"],
["γγγ’γ€γ΅γ¦γ€γ", "βββΓββ€β¬"],
["γγ
γ‘γ
γ
γ
γ
γ
", "β β΅ββ
£βΊΚΙts"],
["ζ±εηΉι«", "ΠΠΠΠΡΡΡΡ"],
["Emojiππ©ββ
", "βββ²βΌβββ‘β₯"]
]
});
βββββββββββββββββββββ¬βββββββββββββββββββ
β Special β Character β
βββββββββββββββββββββΌβββββββββββββββββββ€
β Chinese,δΈζ β 12γζ οΌηΉγγ β
β γγγ’γ€γ΅γ¦γ€γ β βββΓββ€β¬ β
β γγ
γ‘γ
γ
γ
γ
γ
β β β΅ββ
£βΊΚΙts β
β ζ±εηΉι« β ΠΠΠΠΡΡΡΡ β
β Emojiππ©ββ
β βββ²βΌβββ‘β₯ β
βββββββββββββββββββββ΄βββββββββββββββββββ
const CG = require("console-grid");
const eaw = require("eastasianwidth");
CG({
options: {
getCharLength: (char) => {
return eaw.length(char);
}
},
columns: ["Special", "Character"],
rows: [
["Chinese,δΈζ", "12γζ οΌηΉγγ"],
["γγγ’γ€γ΅γ¦γ€γ", "βββΓββ€β¬"],
["γγ
γ‘γ
γ
γ
γ
γ
", "β β΅ββ
£βΊΚΙts"],
["ζ±εηΉι«", "ΠΠΠΠΡΡΡΡ"],
["Emojiππ©ββ
", "βββ²βΌβββ‘β₯"]
]
});
ββββββββββββββββββββ¬βββββββββββββββββββ
β Special β Character β
ββββββββββββββββββββΌβββββββββββββββββββ€
β Chinese,δΈζ β 12γζ οΌηΉγγ β
β γγγ’γ€γ΅γ¦γ€γ β βββΓββ€β¬ β
β γγ
γ‘γ
γ
γ
γ
γ
β β β΅ββ
£βΊΚΙts β
β ζ±εηΉι« β ΠΠΠΠΡΡΡΡ β
β Emojiππ©ββ
β βββ²βΌβββ‘β₯ β
ββββββββββββββββββββ΄βββββββββββββββββββ
{
options: Object, //define grid level options
columns: Array, //define column list and header
rows: Array //define row list
}
{
silent: false,
headerVisible: false,
padding: 1,
defaultMinWidth: 1,
defaultMaxWidth: 50,
sortField: '',
sortAsc: false,
sortIcon: '*',
treeId: 'name',
treeIcon: 'β ',
treeLink: 'β ',
treeLast: 'β ',
treeIndent: ' ',
nullPlaceholder: '-',
//border definition:
//H: horizontal, V: vertical
//T: top, B: bottom, L: left, R: right, C: center
borderH: 'β',
borderV: 'β',
borderTL: 'β',
borderTC: 'β¬',
borderTR: 'β',
borderCL: 'β',
borderCC: 'βΌ',
borderCR: 'β€',
borderBL: 'β',
borderBC: 'β΄',
borderBR: 'β',
getCharLength: defaultGetCharLength
}
{
id: String,
name: String,
type: String, //string, number
align : String, //left(default), center, right
minWidth: Number,
maxWidth: Number,
formatter: Function //custom cell formatter
}
{
//column id key: cell value
innerBorder: Boolean,
subs: Array //sub rows
}
markdown-grid - Markdown Grid Generator
FAQs
Console log a grid
The npm package console-grid receives a total of 277,337 weekly downloads. As such, console-grid popularity was classified as popular.
We found that console-grid demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.