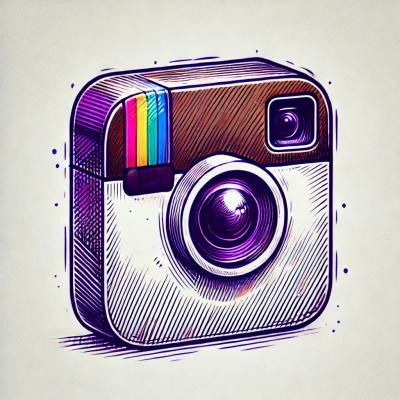
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
content-type-parser
Advanced tools
The content-type-parser npm package is used to parse and handle Content-Type headers in HTTP requests and responses. It helps in extracting the media type, parameters, and other relevant information from Content-Type strings.
Parsing Content-Type
This feature allows you to parse a Content-Type string and extract the media type and parameters. In the example, the Content-Type 'application/json; charset=utf-8' is parsed to extract the type 'application/json' and the charset parameter 'utf-8'.
const contentTypeParser = require('content-type-parser');
const parsed = contentTypeParser('application/json; charset=utf-8');
console.log(parsed.type); // 'application/json'
console.log(parsed.parameters.charset); // 'utf-8'
Handling Invalid Content-Type
This feature demonstrates how the package handles invalid Content-Type strings. If the Content-Type string is invalid, an error is thrown, which can be caught and handled appropriately.
const contentTypeParser = require('content-type-parser');
try {
const parsed = contentTypeParser('invalid-content-type');
} catch (error) {
console.error('Invalid Content-Type:', error.message);
}
Comparing Media Types
This feature allows you to compare two parsed Content-Type objects to check if they represent the same media type. In the example, 'application/json' and 'application/json; charset=utf-8' are considered equal.
const contentTypeParser = require('content-type-parser');
const parsed1 = contentTypeParser('application/json');
const parsed2 = contentTypeParser('application/json; charset=utf-8');
console.log(parsed1.isEqual(parsed2)); // true
The content-type package provides similar functionality for parsing and formatting Content-Type headers. It offers methods to parse Content-Type strings into objects and format objects back into strings. Compared to content-type-parser, it has a simpler API and is widely used in the Node.js ecosystem.
The media-typer package is another alternative for parsing and formatting media types. It focuses on parsing media type strings and formatting them back into strings. It is more lightweight compared to content-type-parser and is often used in conjunction with other HTTP-related packages.
The mime-types package provides utilities for handling MIME types, including methods to look up MIME types based on file extensions and vice versa. While it does not focus solely on Content-Type parsing, it offers a broader range of MIME type-related functionalities compared to content-type-parser.
Content-Type
Header StringsThis package will parse the Content-Type
header field into an introspectable data structure, whose parameters can be manipulated:
const contentTypeParser = require("content-type-parser");
const contentType = contentTypeParser(`Text/HTML;Charset="utf-8"`);
console.assert(contentType.toString() === "text/html;charset=utf-8");
console.assert(contentType.type === "text");
console.assert(contentType.subtype === "html");
console.assert(contentType.get("charset") === "utf-8");
contentType.set("charset", "windows-1252");
console.assert(contentType.get("charset") === "windows-1252");
console.assert(contentType.toString() === "text/html;charset=windows-1252");
console.assert(contentType.isHTML() === true);
console.assert(contentType.isXML() === false);
console.assert(contentType.isText() === true);
Note how parsing will lowercase the type, subtype, and parameter name tokens (but not parameter values).
If the passed string cannot be parsed as a content-type, contentTypeParser
will return null
.
ContentType
instance APIThis package's main module's default export will return an instance of the ContentType
class, which has the following public APIs:
type
: the top-level media type, e.g. "text"
subtype
: the subtype, e.g. "html"
parameterList
: an array of { separator, key, value }
pairs representing the parameters. The separator
field contains any whitespace, not just the ;
character.In general you should not directly manipulate parameterList
. Instead, use the following APIs:
get("key")
: returns the value of the parameter with the given key, or undefined
if no such parameter is presentset("key", "value")
: adds the given key/value pair to the parameter list, or overwrites the existing value if an entry already existedBoth of these will lowercase the keys.
isHTML()
: returns true if this instance's MIME type is the HTML MIME type, "text/html"
isXML()
: returns true if this instance's MIME type is an XML MIME typeisText()
: returns true if this instance's top-level media type is "text"
toString()
will return a canonicalized representation of the content-type, re-built from the parsed componentsThis package was originally based on the excellent work of @nicolashenry, in jsdom. It has since been pulled out into this separate package.
FAQs
Parse the value of the Content-Type header
The npm package content-type-parser receives a total of 314,861 weekly downloads. As such, content-type-parser popularity was classified as popular.
We found that content-type-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.