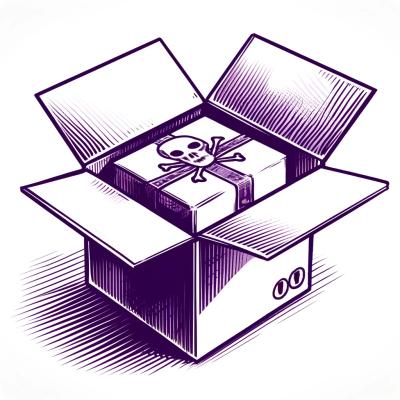
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
cookie-universal
Advanced tools
Universal cookie plugin, perfect for SSR
You can use cookie-universal
to set, get and remove cookies in the browser, node, connect and express apps.
cookie-universal
parse cookies with the popular cookie node module.
yarn add cookie-universal
npm i --save cookie-universal
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.set('cookie-name', 'cookie-value')
})
// browser, from import
import Cookie from 'cookie-universal'
const cookies = Cookie()
cookies.set('cookie-name', 'cookie-value')
// browser, from dist
// note: include dist/cookie-universal.js
const cookies = Cookie()
cookies.set('cookie-name', 'cookie-value')
By default cookie-universal will try to parse to JSON, however you can disable this functionality in several ways:
// server
const parseJSON = false
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res, parseJSON)
})
// browser, from import
import Cookie from 'cookie-universal'
const parseJSON = false
const cookies = Cookie(false, false, parseJSON)
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.parseJSON = false
})
// browser, from import
import Cookie from 'cookie-universal'
const cookies = Cookie(false, false)
cookies.parseJSON = false
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.set('cookie-name', 'cookie-value')
cookies.get('cookie-name', { parseJSON: false })
})
// browser, from import
import Cookie from 'cookie-universal'
const cookies = Cookie(false, false)
cookies.set('cookie-name', 'cookie-value')
cookies.get('cookie-name', { parseJSON: false })
set(name, value, opts)
name
(string): Cookie name to set.value
(string|object): Cookie value.opts
(object): Same as the cookie node module.
path
(string): Specifies the value for the Path Set-Cookie attribute. By default, the path is considered the "default path".expires
(date): Specifies the Date object to be the value for the Expires Set-Cookie attribute.maxAge
(number): Specifies the number (in seconds) to be the value for the Max-Age Set-Cookie attribute.httpOnly
(boolean): Specifies the boolean value for the [HttpOnly Set-Cookie attribute][rfc-6265-5.2.6].domain
(string): specifies the value for the Domain Set-Cookie attribute.encode
(function): Specifies a function that will be used to encode a cookie's value.sameSite
(boolean|string): Specifies the value for the SameSite
Set-Cookie
attribute.true
, false
, 'lax'
, 'none'
, 'strict'
(see details). Default is false
.secure
(boolean): Specifies the boolean value for the Secure Set-Cookie attribute.const cookieValObject = { param1: 'value1', param2: 'value2' }
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.set('cookie-name', 'cookie-value', {
path: '/',
maxAge: 60 * 60 * 24 * 7
})
cookies.set('cookie-name', cookieValObject, {
path: '/',
maxAge: 60 * 60 * 24 * 7
})
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
cookies.set('cookie-name', 'cookie-value', {
path: '/',
maxAge: 60 * 60 * 24 * 7
})
cookies.set('cookie-name', cookieValObject, {
path: '/',
maxAge: 60 * 60 * 24 * 7
})
setAll(cookieArray)
name
(string): Cookie name to set.value
(string|object): Cookie value.opts
(object): Same as the cookie node module.
path
(string): Specifies the value for the Path Set-Cookie attribute. By default, the path is considered the "default path".expires
(date): Specifies the Date object to be the value for the Expires Set-Cookie attribute.maxAge
(number): Specifies the number (in seconds) to be the value for the Max-Age Set-Cookie attribute.httpOnly
(boolean): Specifies the boolean value for the [HttpOnly Set-Cookie attribute][rfc-6265-5.2.6].domain
(string): specifies the value for the Domain Set-Cookie attribute.encode
(function): Specifies a function that will be used to encode a cookie's value.sameSite
(boolean|string): Specifies the value for the SameSite
Set-Cookie
attribute.true
, false
, 'lax'
, 'none'
, 'strict'
(see details). Default is false
.secure
(boolean): Specifies the boolean value for the Secure Set-Cookie attribute.const options = {
path: '/',
maxAge: 60 * 60 * 24 * 7
}
const cookieList = [
{ name: 'cookie-name1', value: 'value1', opts: options },
{ name: 'cookie-name2', value: 'value2', opts: options },
{ name: 'cookie-name3', value: 'value3', opts: options },
{ name: 'cookie-name4', value: 'value4', opts: options }
]
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.setAll(cookieList)
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
cookies.setAll(cookieList)
get(name, opts)
name
(string): Cookie name to get.opts
fromRes
(boolean): Get cookies from res instead of req.parseJSON
(boolean): Parse json, true by default unless overridden globally or locally.// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
const cookieRes = cookies.get('cookie-name')
const cookieRes = cookies.get('cookie-name', { fromRes: true }) // get from res instead of req
// returns the cookie value or undefined
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
const cookieRes = cookies.get('cookie-name')
// returns the cookie value or undefined
getAll(opts)
opts
fromRes
(boolean): Get cookies from res instead of req.parseJSON
(boolean): Parse json, true by default unless overridden globally or locally.// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
const cookiesRes = cookies.getAll()
const cookiesRes = cookies.getAll({ fromRes: true }) // get from res instead of req
// returns all cookies or {}
{
"cookie-1": "value1",
"cookie-2": "value2",
}
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
const cookiesRes = cookies.getAll()
// returns all cookies or {}
{
"cookie-1": "value1",
"cookie-2": "value2",
}
remove(name, opts)
name
(string): Cookie name to remove.opts
path
(string): Specifies the value for the Path Set-Cookie attribute. By default, the path is considered the "default path".expires
(date): Specifies the Date object to be the value for the Expires Set-Cookie attribute.maxAge
(number): Specifies the number (in seconds) to be the value for the Max-Age Set-Cookie attribute.httpOnly
(boolean): Specifies the boolean value for the [HttpOnly Set-Cookie attribute][rfc-6265-5.2.6].domain
(string): specifies the value for the Domain Set-Cookie attribute.encode
(function): Specifies a function that will be used to encode a cookie's value.sameSite
(boolean|string): Specifies the value for the SameSite
Set-Cookie
attribute.true
, false
, 'lax'
, 'none'
, 'strict'
(see details). Default is false
.secure
(boolean): Specifies the boolean value for the Secure Set-Cookie attribute.// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.remove('cookie-name')
cookies.remove('cookie-name', {
// this will allow you to remove a cookie
// from a different path
path: '/my-path'
})
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
cookies.remove('cookie-name')
removeAll(opts)
opts
path
(string): Specifies the value for the Path Set-Cookie attribute. By default, the path is considered the "default path".expires
(date): Specifies the Date object to be the value for the Expires Set-Cookie attribute.maxAge
(number): Specifies the number (in seconds) to be the value for the Max-Age Set-Cookie attribute.httpOnly
(boolean): Specifies the boolean value for the [HttpOnly Set-Cookie attribute][rfc-6265-5.2.6].domain
(string): specifies the value for the Domain Set-Cookie attribute.encode
(function): Specifies a function that will be used to encode a cookie's value.sameSite
(boolean|string): Specifies the value for the SameSite
Set-Cookie
attribute.true
, false
, 'lax'
, 'none'
, 'strict'
(see details). Default is false
.secure
(boolean): Specifies the boolean value for the Secure Set-Cookie attribute.// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
cookies.removeAll()
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
cookies.removeAll()
nodeCookie
This property will expose the cookie node module so you don't have to include it yourself.
// server
app.get('/', (req, res) => {
const cookies = require('cookie-universal')(req, res)
const cookieRes = cookies.nodeCookie.parse('cookie-name', 'cookie-value')
cookieRes['cookie-name'] // returns 'cookie-value'
})
// client
import Cookie from 'cookie-universal'
const cookies = Cookie()
const cookieRes = cookies.nodeCookie.parse('cookie-name', 'cookie-value')
cookieRes['cookie-name'] // returns 'cookie-value'
Copyright (c) Salvatore Tedde microcipcip@gmail.com
FAQs
Universal cookie plugin, perfect for SSR
The npm package cookie-universal receives a total of 76,336 weekly downloads. As such, cookie-universal popularity was classified as popular.
We found that cookie-universal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.