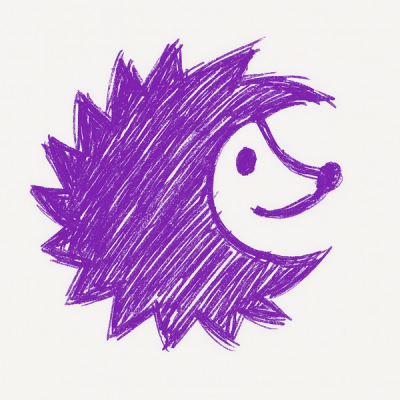
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
core-router
Advanced tools
Primitives for building your own router.
match()
match()
provides flexible rule-based URL matching.
You can:
match({ path: "/account/:accountId" }, "/account/123");
result = {
params: {
accountId: "123";
}
}
match(
{
path: "/account/:accountId",
search: {
from: ":fromDate?",
to: ":toDate?"
},
condition: ({ params }) => {
return isDate(params.fromDate) && isDate(params.toDate);
}
},
"/account/123?from=2020-01-01&to=2020-01-31"
);
result = {
params: {
accountId: "123",
fromDate: "2020-01-01",
toDate: "2020-01-31"
}
};
match(
{
condition: ({ url }) => {
return url.hash === "#secret";
}
},
"/you/must/have/the#secret"
);
result = {
params: {}
};
Absolute URLs will only match if they belong to the same domain:
// On https://foo.com:
match({ path: "/hello" }, "https://bar.com/hello");
result = null;
Params will be decoded for you:
match({ search: { q: ":searchTerms" } }, "/?q=hello%20world");
result = {
params: {
searchTerms: "hello world"
}
};
toHref()
Any rule that can be used for match()
can also be used to generate a relative URL.
toHref({ path: "/account/:accountId" }, { accountId: "123" });
result = "/account/123";
If required params are not supplied, an error will be thrown:
toHref({ path: "/account/:accountId" }, {});
// Error!
Similarly, if the condition
is not met, an error will alos be thrown:
toHref(
{
condition: ({ url }) => url.hash === "#secret"
},
"/#incorrect"
);
// Error!
This ensures that for any rule, a URL generated by toHref()
will also match()
:
const href = toHref(myRule, myParams);
const matchResult = match(myRule, href);
expect(matchResult).not.toBe(null);
expect(matchResult.params).toEqual(myParams);
FAQs
Primitives for building your own router
We found that core-router demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.