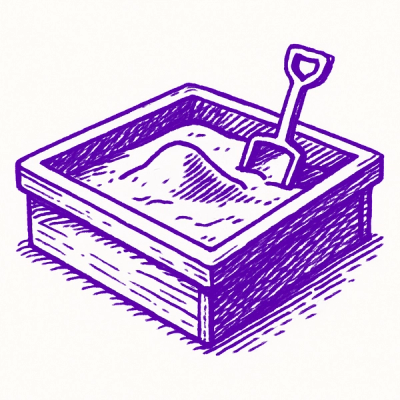
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
cosdata-sdk
Advanced tools
A TypeScript/JavaScript SDK for interacting with the Cosdata Vector Database.
npm install cosdata-sdk
import { createClient } from 'cosdata-sdk';
// Initialize the client (all parameters are optional)
const client = createClient({
host: 'http://127.0.0.1:8443', // Default host
username: 'admin', // Default username
password: 'test_key', // Default password
verifySSL: false // SSL verification
});
// Create a collection
const collection = await client.createCollection({
name: 'my_collection',
dimension: 128,
dense_vector: {
enabled: true,
dimension: 128,
auto_create_index: false
}
});
// Create an index
const index = await collection.createIndex({
name: 'my_collection_dense_index',
distance_metric: 'cosine',
quantization_type: 'auto',
sample_threshold: 100,
num_layers: 16,
max_cache_size: 1024,
ef_construction: 128,
ef_search: 64,
neighbors_count: 10,
level_0_neighbors_count: 20
});
// Generate some vectors
function generateRandomVector(dimension: number): number[] {
return Array.from({ length: dimension }, () => Math.random());
}
const vectors = Array.from({ length: 100 }, (_, i) => ({
id: `vec_${i}`,
dense_values: generateRandomVector(128),
document_id: `doc_${i}`
}));
// Add vectors using a transaction
const txn = collection.transaction();
await txn.batch_upsert_vectors(vectors);
await txn.commit();
// Search for similar vectors
const results = await collection.getSearch().dense({
query_vector: generateRandomVector(128),
top_k: 5,
return_raw_text: true
});
// Verify vector existence
const exists = await collection.getVectors().exists('vec_1');
console.log('Vector exists:', exists);
// Get collection information
const collectionInfo = await collection.getInfo();
console.log('Collection info:', collectionInfo);
// List all collections
const collections = await client.listCollections();
console.log('Available collections:', collections);
// Version management
const currentVersion = await collection.getVersions().getCurrent();
console.log('Current version:', currentVersion);
// Clean up
await collection.delete();
The main client for interacting with the Vector Database API.
const client = createClient({
host: 'http://127.0.0.1:8443', // Optional
username: 'admin', // Optional
password: 'test_key', // Optional
verifySSL: false // Optional
});
Methods:
createCollection(options: { name: string, dimension: number, dense_vector?: { enabled: boolean, dimension: number, auto_create_index: boolean }, sparse_vector?: { enabled: boolean, auto_create_index: boolean }, tf_idf_options?: { enabled: boolean } }): Promise<Collection>
listCollections(): Promise<string[]>
getCollection(name: string): Promise<Collection>
The Collection class provides access to all collection-specific operations.
const collection = await client.createCollection({
name: 'my_collection',
dimension: 128,
dense_vector: {
enabled: true,
dimension: 128,
auto_create_index: false
}
});
Methods:
createIndex(options: { name: string, distance_metric: string, quantization_type: string, sample_threshold: number, num_layers: number, max_cache_size: number, ef_construction: number, ef_search: number, neighbors_count: number, level_0_neighbors_count: number }): Promise<Index>
getInfo(): Promise<CollectionInfo>
interface CollectionInfo {
name: string;
description?: string;
dense_vector?: {
enabled: boolean;
dimension: number;
auto_create_index: boolean;
};
sparse_vector?: {
enabled: boolean;
auto_create_index: boolean;
};
metadata_schema?: Record<string, any>;
config?: {
max_vectors?: number;
replication_factor?: number;
};
}
delete(): Promise<void>
transaction(): Transaction
getVectors(): Vectors
getSearch(): Search
getVersions(): Versions
The Transaction class provides methods for vector operations.
const txn = collection.transaction();
await txn.batch_upsert_vectors(vectors);
await txn.commit();
Methods:
upsert_vector(vector: Vector): Promise<void>
batch_upsert_vectors(vectors: Vector[], maxWorkers?: number, maxRetries?: number): Promise<void>
commit(): Promise<void>
abort(): Promise<void>
The Search class provides methods for vector similarity search.
const results = await collection.getSearch().dense({
query_vector: vector,
top_k: 5,
return_raw_text: true
});
Methods:
dense(options: { query_vector: number[], top_k?: number, return_raw_text?: boolean }): Promise<SearchResponse>
interface SearchResponse {
results: SearchResult[];
}
interface SearchResult {
id: string;
document_id?: string;
score: number;
text?: string | null;
}
sparse(options: { query_terms: number[][], top_k?: number, early_terminate_threshold?: number, return_raw_text?: boolean }): Promise<SearchResponse>
text(options: { query_text: string, top_k?: number, return_raw_text?: boolean }): Promise<SearchResponse>
The Vectors class provides methods for vector operations.
const exists = await collection.getVectors().exists('vec_1');
const vector = await collection.getVectors().get('vec_1');
Methods:
get(vector_id: string): Promise<any>
interface VectorObject {
id: string;
document_id?: string;
dense_values?: number[];
sparse_indices?: number[];
sparse_values?: number[];
text?: string;
}
exists(vector_id: string): Promise<boolean>
delete(vector_id: string): Promise<void>
The Versions class provides methods for version management.
const currentVersion = await collection.getVersions().getCurrent();
const allVersions = await collection.getVersions().list();
Methods:
getCurrent(): Promise<Version>
interface Version {
hash: string;
version_number: number;
timestamp: number;
vector_count: number;
}
list(): Promise<ListVersionsResponse>
interface ListVersionsResponse {
versions: Version[];
current_hash: string;
}
getByHash(versionHash: string): Promise<Version>
Connection Management
createClient()
to initialize the clientVector Operations
commit()
after successful operationsabort()
in case of errorsError Handling
Performance
Version Management
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
TypeScript SDK for Cosdata Vector Database API
We found that cosdata-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.