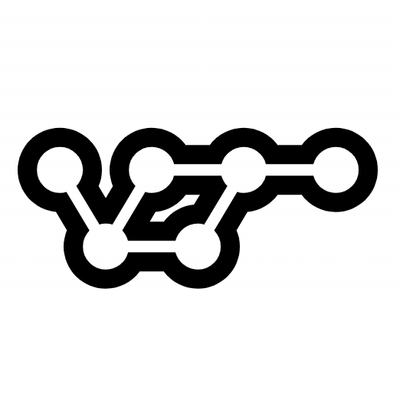
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
cosmicjs-node
Advanced tools
The official client module for Cosmic JS. This module helps you easily add dynamic content to your website or application.
This is the Official Cosmic JS JavaScript Client which allows you to easily create, read, update and delete content from your Cosmic JS Buckets. Includes cosmicjs.browser.min.js
for easy integration in the browser.
Go to https://cosmicjs.com, create an account and set up a Bucket.
npm install cosmicjs
<script src="https://unpkg.com/cosmicjs@latest/cosmicjs.browser.min.js"></script>
<script>
// Exposes the global variable Cosmic
// Never expose your private keys or credentials in any public website's client-side code
</script>
Use your Cosmic JS account email and password to create an authentication token. At this time, authentication is only necessary for adding Buckets.
const Cosmic = require('cosmicjs')() // empty init
Cosmic.authenticate({
email: 'you@youremail.com',
password: 'yourpassword'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Add a new Bucket to your account.
const Cosmic = require('cosmicjs')({
token: 'your-token-from-auth-request' // from Cosmic.authenticate
})
Cosmic.addBucket({
title: 'My New Bucket',
slug: 'my-new-bucket' // must be unique across all Buckets in system
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Use the Cosmic.bucket
method to connect to different Buckets in your account. If you would like to restrict read and write access to your Bucket, you can do so in Your Bucket > Basic Settings in your Cosmic JS Dashboard.
// Use the Cosmic.bucket method to connect to different Buckets in your account.
const Cosmic = require('cosmicjs')({
token: 'your-token-from-auth-request' // optional
})
const bucket = Cosmic.bucket({
slug: 'my-new-bucket',
read_key: '',
write_key: ''
})
const bucket2 = Cosmic.bucket({
bucket: 'my-other-bucket',
read_key: '',
write_key: ''
})
Returns the entire Bucket including Object Types, Objects, Media and more.
bucket.getBucket().then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Add a new Object Type to your Bucket.
const params = {
title: 'Pages',
singular: 'Page',
slug: 'pages',
metafields: [
{
type: 'text',
title: 'Headline',
key: 'headline',
required: true
},
{
type: 'file',
title: 'Hero',
key: 'hero',
required: true
}
]
}
bucket.addObjectType(params).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Get all Object Types in your Bucket.
bucket.getObjectTypes().then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Edit an existing Object Type in your Bucket.
bucket.editObjectType({
slug: 'posts',
title: 'New Posts Title'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Delete an existing Object Type from your Bucket. *This does not delete Objects in this Object Type.
bucket.deleteObjectType({
slug: 'posts'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Add a new Object to your Bucket.
const params = {
title: 'Cosmic JS Example',
type_slug: 'examples',
content: 'Learning the Cosmic JS API is really fun and so easy',
metafields: [
{
key: 'Headline',
type: 'text',
value: 'Learn Cosmic JS!'
},
{
key: 'Author',
type: 'text',
value: 'Quasar Jones'
}
],
options: {
slug_field: false
}
}
bucket.addObject(params).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Returns all Objects from your Bucket.
bucket.getObjects({
limit: 2
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Get Objects from an Object Type.
const params = {
type_slug: 'posts',
limit: 5,
skip: 0,
sort: '-created_at', // optional, if sort is needed. (use one option from 'created_at,-created_at,modified_at,-modified_at,random')
locale: 'en' // optional, if localization set on Objects
status: 'all' // optional, if need to get draft Objects
}
bucket.getObjectsByType(params).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Search Objects in an Object Type.
// Search by connected Object Metafield
const params = {
type_slug: 'posts',
metafield_key: 'author',
metafield_object_slug: 'carson-gibbons',
limit: 5,
skip: 0,
sort: '-created_at', // optional, if sort is needed. (use one option from 'created_at,-created_at,modified_at,-modified_at,random')
locale: 'en' // optional, if localization set on Objects,
status: 'all' // optional, if need to get draft Objects,
}
// Search by Metafield Value
const params = {
type_slug: 'posts',
metafield_key: 'headline',
metafield_value: 'Hello World',
limit: 5,
skip: 0,
sort: '-created_at', // optional, if sort is needed. (use one option from 'created_at,-created_at,modified_at,-modified_at,random')
}
// Search by Metafield Has Value
const params = {
type_slug: 'posts',
metafield_key: 'headline',
metafield_has_value: 'World',
limit: 5,
skip: 0,
sort: '-created_at', // optional, if sort is needed. (use one option from 'created_at,-created_at,modified_at,-modified_at,random')
}
bucket.searchObjectType(params).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Edit an existing Object in your Bucket.
bucket.editObject({
slug: 'cosmic-js-example',
title: 'New Title Edit'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Delete an existing Object in your Bucket.
bucket.deleteObject({
slug: 'cosmic-js-example'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
The only required post value is media which is the name of your media sent.
bucket.addMedia({
media: '<FILE_DATA>',
folder: 'your-folder-slug',
metadata: {
caption: 'Beautiful picture of the beach',
credit: 'Tyler Jackson'
}
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
You can add the folder
parameter to get Media from a certain folder. You can use the full Imgix suite of image processing tools on the URL provided by the imgix_url
property value. Check out the Imgix documentation for more info.
bucket.getMedia({
folder: 'groomsmen',
limit: 3
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
bucket.deleteMedia({
id: '5a4b18e12fff7ec0e3c13c65'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Sends a POST request to the endpoint of your choice when the event occurs. The data payload in the same fomat as Object and Media. Read more about Webhooks including the payload sent to the endpoint on the Webhooks documentation page.
bucket.addWebhook({
event: 'object.created.published',
endpoint: 'http://my-listener.com'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
bucket.deleteWebhook({
id: 'c62defe0-5f93-11e7-8054-873245f0e98d'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
Adds an Extension to your Bucket. Required post values include zip which is the name of your file sent. Read more about Extensions on the Extensions documentation page.
bucket.addExtension({
zip: '<ZIP_FILE_DATA>'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
bucket.deleteExtension({
id: 'c62defe0-5f93-11e7-8054-873245f0e98d'
}).then(data => {
console.log(data)
}).catch(err => {
console.log(err)
})
FAQs
The official client module for Cosmic JS. This module helps you easily add dynamic content to your website or application.
The npm package cosmicjs-node receives a total of 0 weekly downloads. As such, cosmicjs-node popularity was classified as not popular.
We found that cosmicjs-node demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.