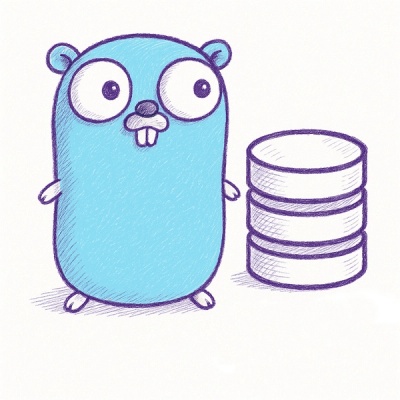
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
create-conical-gradient
Advanced tools
A pretty extension for CanvasRenderingContext2D to create a pattern of the conical gradient.
A pretty extension for CanvasRenderingContext2D to create a pattern of the conical gradient.
CSS3 already supports the conical gradients by the property conic-gradient
, but unfortunately, HTML Canvas API does not yet. One good news to you is that use this package to implement that, by a method similar to CanvasRenderingContext2D.createLinearGradient()
and CanvasRenderingContext2D.createRadialGradient()
.
Install the npm package for development:
yarn add create-conical-gradient # OR `npm i --save create-conical-gradient`
Of course, you can also use the umd resources for production:
<script src="https://unpkg.com/create-conical-gradient@latest/umd/create-conical-gradient.min.js"></script>
Codes:
<canvas id="my-canvas" width="480" height="270">
Your browser does not support canvas...
</canvas>
import 'create-conical-gradient'; // If you use the npm package.
const canvas = document.getElementById('my-canvas');
const ctx = canvas.getContext('2d');
const gradient = ctx.createConicalGradient(240, 135, -Math.PI, Math.PI);
gradient.addColorStop(0, '#f00');
gradient.addColorStop(0.2, '#00f');
gradient.addColorStop(0.4, '#0ff');
gradient.addColorStop(0.6, '#f0f');
gradient.addColorStop(0.8, '#ff0');
gradient.addColorStop(1, '#f00');
ctx.fillStyle = gradient.pattern;
ctx.fillRect(0, 0, canvas.width, canvas.height);
Output:
void ctx.createConicalGradient(ox, oy, startAngle, endAngle, anticlockwise);
ox
The x-axis coordinate of the origin of the gradient pattern, which default value is 0
.
oy
The y-axis coordinate of the origin of the gradient pattern, which default value is 0
.
startAngle
The angle at which the arc starts in radians measured from the positive x-axis, which default value is 0
.
endAngle
The angle at which the arc ends in radians measured from the positive x-axis, which default value is 2 * Math.PI
.
anticlockwise
An optional Boolean
. If true, draws the gradient counter-clockwise between the start and end angles. The default is false
(clockwise).
void gradient.addColorStop(offset, color);
offset
A number between 0
and 1
, inclusive, representing the position of the color stop. 0
represents the start of the gradient and 1
represents the end; an INDEX_SIZE_ERR
is raised if the number is outside that range.
color
A CSS <color> value representing the color of the stop. A SYNTAX_ERR
is raised if the value cannot be parsed as a CSS <color> value.
FAQs
A pretty extension for CanvasRenderingContext2D to create a pattern of the conical gradient.
The npm package create-conical-gradient receives a total of 91 weekly downloads. As such, create-conical-gradient popularity was classified as not popular.
We found that create-conical-gradient demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.