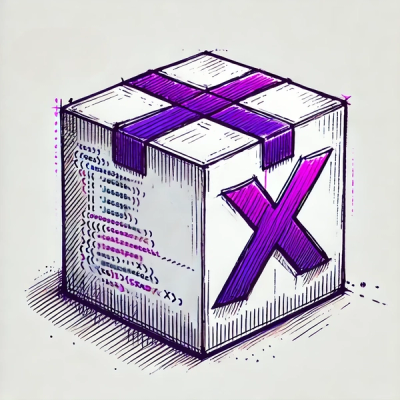
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
This package is no longer supported and has been deprecated. To avoid malicious use, npm is hanging on to the package name.
The 'crypto' npm package provides cryptographic functionality that includes a set of wrappers for OpenSSL's hash, HMAC, cipher, decipher, sign, and verify functions. It is used for secure data encryption, decryption, hashing, and more.
Hashing
This feature allows you to create a hash of data using various algorithms like SHA-256. The code sample demonstrates how to create a SHA-256 hash of a string.
const crypto = require('crypto');
const hash = crypto.createHash('sha256');
hash.update('some data to hash');
console.log(hash.digest('hex'));
HMAC
HMAC (Hash-based Message Authentication Code) is used for data integrity and authenticity. The code sample shows how to create an HMAC using SHA-256 and a secret key.
const crypto = require('crypto');
const hmac = crypto.createHmac('sha256', 'a secret key');
hmac.update('some data to hash');
console.log(hmac.digest('hex'));
Encryption
This feature allows you to encrypt data using various algorithms like AES. The code sample demonstrates how to encrypt a string using AES-256-CBC.
const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const key = crypto.randomBytes(32);
const iv = crypto.randomBytes(16);
const cipher = crypto.createCipheriv(algorithm, key, iv);
let encrypted = cipher.update('some data to encrypt', 'utf8', 'hex');
encrypted += cipher.final('hex');
console.log(encrypted);
Decryption
This feature allows you to decrypt data that was encrypted using the 'crypto' package. The code sample demonstrates how to decrypt a string that was encrypted using AES-256-CBC.
const crypto = require('crypto');
const algorithm = 'aes-256-cbc';
const key = crypto.randomBytes(32);
const iv = crypto.randomBytes(16);
const encrypted = '...'; // previously encrypted data
const decipher = crypto.createDecipheriv(algorithm, key, iv);
let decrypted = decipher.update(encrypted, 'hex', 'utf8');
decrypted += decipher.final('utf8');
console.log(decrypted);
Digital Signatures
This feature allows you to create digital signatures for data. The code sample demonstrates how to sign data using RSA and SHA-256.
const crypto = require('crypto');
const { privateKey, publicKey } = crypto.generateKeyPairSync('rsa', {
modulusLength: 2048,
});
const sign = crypto.createSign('SHA256');
sign.update('some data to sign');
sign.end();
const signature = sign.sign(privateKey, 'hex');
console.log(signature);
Verification
This feature allows you to verify digital signatures. The code sample demonstrates how to verify a signature using RSA and SHA-256.
const crypto = require('crypto');
const { privateKey, publicKey } = crypto.generateKeyPairSync('rsa', {
modulusLength: 2048,
});
const sign = crypto.createSign('SHA256');
sign.update('some data to sign');
sign.end();
const signature = sign.sign(privateKey, 'hex');
const verify = crypto.createVerify('SHA256');
verify.update('some data to sign');
verify.end();
console.log(verify.verify(publicKey, signature, 'hex'));
bcrypt is a library to help you hash passwords. It is designed to be computationally expensive to slow down brute-force attacks. Unlike 'crypto', which provides a wide range of cryptographic functionalities, bcrypt is specialized for password hashing.
jsonwebtoken is a library to sign, verify, and decode JSON Web Tokens (JWT). It is commonly used for authentication and authorization in web applications. While 'crypto' can be used to create and verify signatures, jsonwebtoken simplifies the process of working with JWTs.
crypto-js is a JavaScript library of crypto standards. It provides a variety of cryptographic algorithms for hashing, encryption, and decryption. It is similar to 'crypto' but is designed to work in both Node.js and browser environments.
node-forge is a native implementation of TLS (and various other cryptographic tools) in JavaScript. It provides a wide range of cryptographic functionalities similar to 'crypto', but with additional features like TLS/SSL support.
This package is no longer supported and has been deprecated. To avoid malicious use, npm is hanging on to the package name.
It's now a built-in Node module. If you've depended on crypto, you should switch to the one that's built-in.
Please contact support@npmjs.com if you have questions about this package.
FAQs
This package is no longer supported and has been deprecated. To avoid malicious use, npm is hanging on to the package name.
The npm package crypto receives a total of 1,159,605 weekly downloads. As such, crypto popularity was classified as popular.
We found that crypto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.