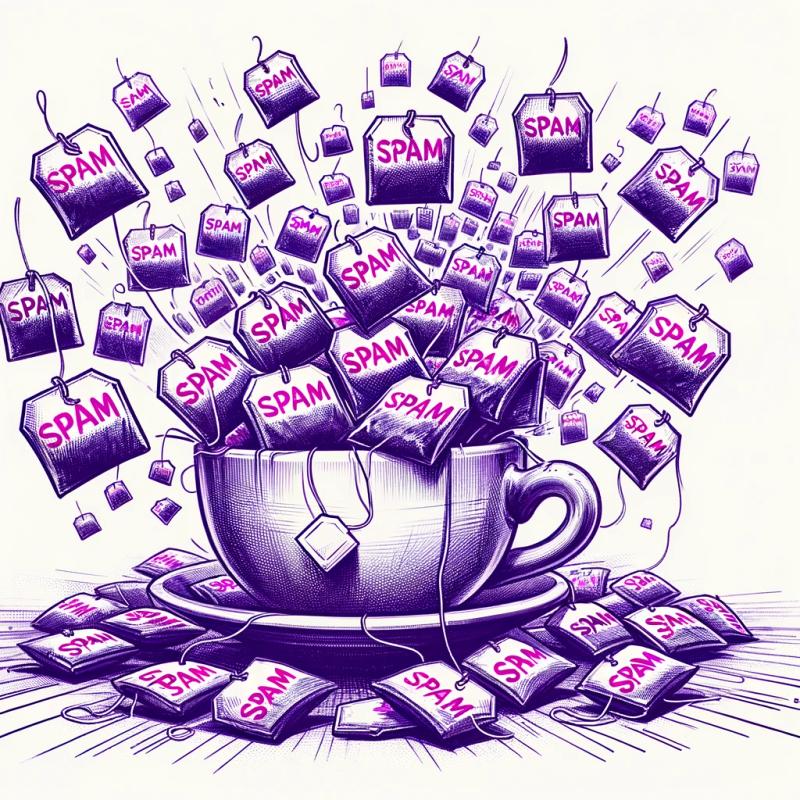
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
crypto-aggregator
Advanced tools
Readme
Continuously scans exchanges and calculates the volume-weighted average market pair price (VWAMPP) of each cryptocurrency.
Volume-Weighted Average Market Market Pair Price (VWAMPP) is the average of the market pair prices from all exchanges where each market pair price is weighted by its volume.
For more details on how VWAMPP is calculated and why it is used, see this article.
npm install crypto-aggregator --save
In its simplest form, it can run as:
let options = {
// Forex conversions
getForexData_oxr: true, // Mocked values will be uses if set to false
osx_app_id: "YOUR_OSX_APP_ID" // openexchangerates.org app id
};
var ca = require("../index.js")(options);
ca.start(options);
Below are other options and their default values:
options: {
loopForEver: true,
maxNumberOfIterations: 10, // ignored if loopForEver == true
printBanner: true,
getCoinGeckoPrices: true, // Get CoinGecko prices as a reference to compare our prices (optional)
// Forex conversions
getForexData_oxr: false, // Mocked values will be uses if set to false
osx_app_id: "YOUR_OSX_APP_ID", // openexchangerates.org app id
// control
enable: true, // Used for start/stop
// Outlier detection
bypassOutliers: true, // Ignores outliers
outlierStandardDeviationDistanceFromMean: 3, // distance from mean for an outlier in sigma
aggregatePriceInterval_ms: 5000, // Aggregate price rate
coingGeckoUpdateInterval_ms: 100 * 1000, // CoinGecko Refresh rate
statusBarTextInterval_ms: 1000, // Status bar update refersh rate
aggregatePricesCallBack: null, // Called whenever the partial WVAP is calculated
iterationCallBack: null, // Calculated whenever all exchanges and pairs were queired at the end of one iteration
discoveredOneTickerCallBack:null, // Called whenever found a new pair on an exchange
bPrintStatus: true, //Print the status bar
printAllPrices: true, // Print a summary table in the log file
coinsInStatusBar: ["BTC", "ETH"], // Coins to be shown in the summary status bar
ccxtExchangeRateLimitDivider:1, // Divides CCXT's recommended rateLimit time if filling adventerous!
// Exchanges to query from
trustedExchanges: [
"huobipro",
"kraken",
"binance",
"bittrex",
"bitmex",
"bitstamp",
"coinbasepro",
"gemini",
"itbit",
"bitflyer",
"poloniex",
"independentreserve",
"liquid",
"upbit"
],
// Exchanges to exclude even if they are in trustedExchanges
excludeExchanges: [
"_1btcxe",
"allcoin",
"theocean",
"xbtce",
"cointiger",
"bibox",
"coolcoin",
"uex",
"dsx",
"flowbtc",
"bcex"
]
}
For simplicity, all discovered data and VWAMPP price for all cryptocurrencies is kept in memory. Callback functions are provided which can be used to write data in your own database.
The in-memory values are as follows:
The VWAMPP price for all cryptos are in pricesInUSD
. Example:
let options = {
// Forex conversions
getForexData_oxr: false, // Mocked values will be uses if set to false
osx_app_id: "YOUR_OSX_APP_ID" // openexchangerates.org app id
};
var ca = require("../index.js")(options);
ca.start(options);
// Print the calculated VWAMPP price of BTC after 5 minutes:
setTimeout(() => {
let pricesInUSD = ca.pricesInUSD;
let log = ca.log;
log.info(`BTC price is: `, pricesInUSD["BTC"]);
}, 5 * 60 * 1000);
Makrket pairs are kept inside an object called tickers
. They are organized under a tree of:
let cryptoName = "BTC"; // String
let exchangeName = "binance"; // String
let index = 0; // String
tickers[cryptoName][exchangeName]["pairs"][index].ticker;
tickers[cryptoName][exchangeName]["pairs"][index].market;
where market
and ticker
are taken directly from ccxt
output of fetchTicker
and fetchMarkets
functions.
Example:
let options = {
// Forex conversions
getForexData_oxr: true, // Mocked values will be uses if set to false
osx_app_id: "YOUR_OSX_APP_ID" // openexchangerates.org app id
};
var ca = require("../index.js")(options);
ca.start(options);
// Print discovered tickers after 5 minutes:
setTimeout(() => {
let tickers = ca.tickers;
let log = ca.log;
log.info(`tickers=`, JSON.stringify(tickers));
}, 5 * 60 * 1000);
// Output:
// INFO: tickers = {
// 'BTC' : {
// 'binance': {
// pairs: [{ticker: {...}, market: {symbol: 'BTC/USDT', ...}},
// {ticker: {...}, market: {symbol: 'BTC/BNB', ...}}
// ]
// },
// 'kraken': {
// pairs: [{ticker: {...}, market: {symbol: 'ETH/BTC', ...}},
// {ticker: {...}, market: {symbol: 'BTC/USDT', ...}},
// ]
// }
// }
// ...
// }
The logger used in this package is available separately in log-with-statusbar npm package
Free to use under ICS. Backlinks and credit are greatly appreciated!
Issues, contributions, and pull requests are welcome!
FAQs
Aggregates cryptocurrency prices from various exchanges. Prices from different exchanges are weighted by their volume. The result is the Volume-Weighted-Average-Market-Pair-Price (VWAMPP) for each cryptocurrency.
The npm package crypto-aggregator receives a total of 7 weekly downloads. As such, crypto-aggregator popularity was classified as not popular.
We found that crypto-aggregator demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.