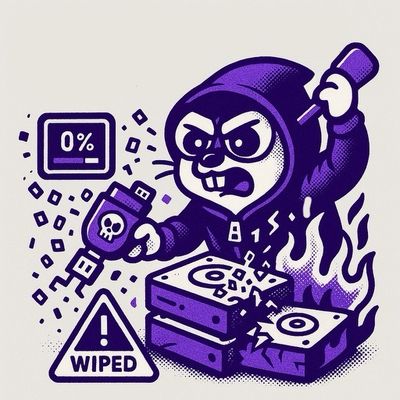
Research
wget to Wipeout: Malicious Go Modules Fetch Destructive Payload
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Cryptr is a simple encryption and decryption library for Node.js. It uses the AES-256-GCM algorithm to securely encrypt and decrypt strings.
Encryption
This feature allows you to encrypt a string using a secret key. The encrypted string can be safely stored or transmitted.
const Cryptr = require('cryptr');
const cryptr = new Cryptr('myTotallySecretKey');
const encryptedString = cryptr.encrypt('myString');
console.log(encryptedString);
Decryption
This feature allows you to decrypt a previously encrypted string using the same secret key. The decrypted string is the original plaintext.
const Cryptr = require('cryptr');
const cryptr = new Cryptr('myTotallySecretKey');
const decryptedString = cryptr.decrypt(encryptedString);
console.log(decryptedString);
CryptoJS is a widely-used library that provides a variety of cryptographic algorithms including AES, DES, and SHA. It offers more flexibility and options compared to Cryptr, but may require more configuration.
Bcrypt is a library specifically designed for hashing passwords. While it does not provide general-purpose encryption and decryption like Cryptr, it is highly effective for securely storing passwords.
Node-forge is a comprehensive library that provides a wide range of cryptographic tools including encryption, decryption, hashing, and digital signatures. It is more feature-rich compared to Cryptr but also more complex to use.
cryptr is a simple aes-256-gcm
encrypt and decrypt module for node.js
It is for doing simple encryption of values UTF-8 strings that need to be decrypted at a later time.
If you require anything more than that you probably want to use something more advanced or crypto directly.
The Cryptr constructor takes 1 required argument, and an optional options object.
Cryptr(secret[, options])
<string>
<Object>
<string>
Defaults to 'hex' (see Node.js Buffer documentation for valid options)<number>
Defaults to 100000<number>
Defaults to 64The salt
and iv
are randomly generated and prepended to the result.
DO NOT USE THIS MODULE FOR ENCRYPTING PASSWORDS!
Passwords should be a one way hash. Use bcrypt for that.
npm install cryptr
const Cryptr = require('cryptr');
const cryptr = new Cryptr('myTotallySecretKey');
const encryptedString = cryptr.encrypt('bacon');
const decryptedString = cryptr.decrypt(encryptedString);
console.log(encryptedString); // 2a3260f5ac4754b8ee3021ad413ddbc11f04138d01fe0c5889a0dd7b4a97e342a4f43bb43f3c83033626a76f7ace2479705ec7579e4c151f2e2196455be09b29bfc9055f82cdc92a1fe735825af1f75cfb9c94ad765c06a8abe9668fca5c42d45a7ec233f0
console.log(decryptedString); // bacon
const Cryptr = require('cryptr');
const cryptr = new Cryptr('myTotallySecretKey', { encoding: 'base64', pbkdf2Iterations: 10000, saltLength: 10 });
const encryptedString = cryptr.encrypt('bacon');
const decryptedString = cryptr.decrypt(encryptedString);
console.log(encryptedString); // CPbKO/FFLQ8lVKxV+jYJcLcpTU0ZvW3D+JVfUecmJmLYY10UxYEa/wf8PWDQqhw=
console.log(decryptedString); // bacon
FAQs
a simple encrypt and decrypt module for node.js
The npm package cryptr receives a total of 164,053 weekly downloads. As such, cryptr popularity was classified as popular.
We found that cryptr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
Product
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.